Creating an abstract class in Objective-C
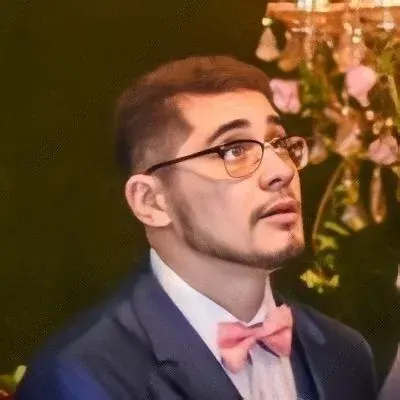
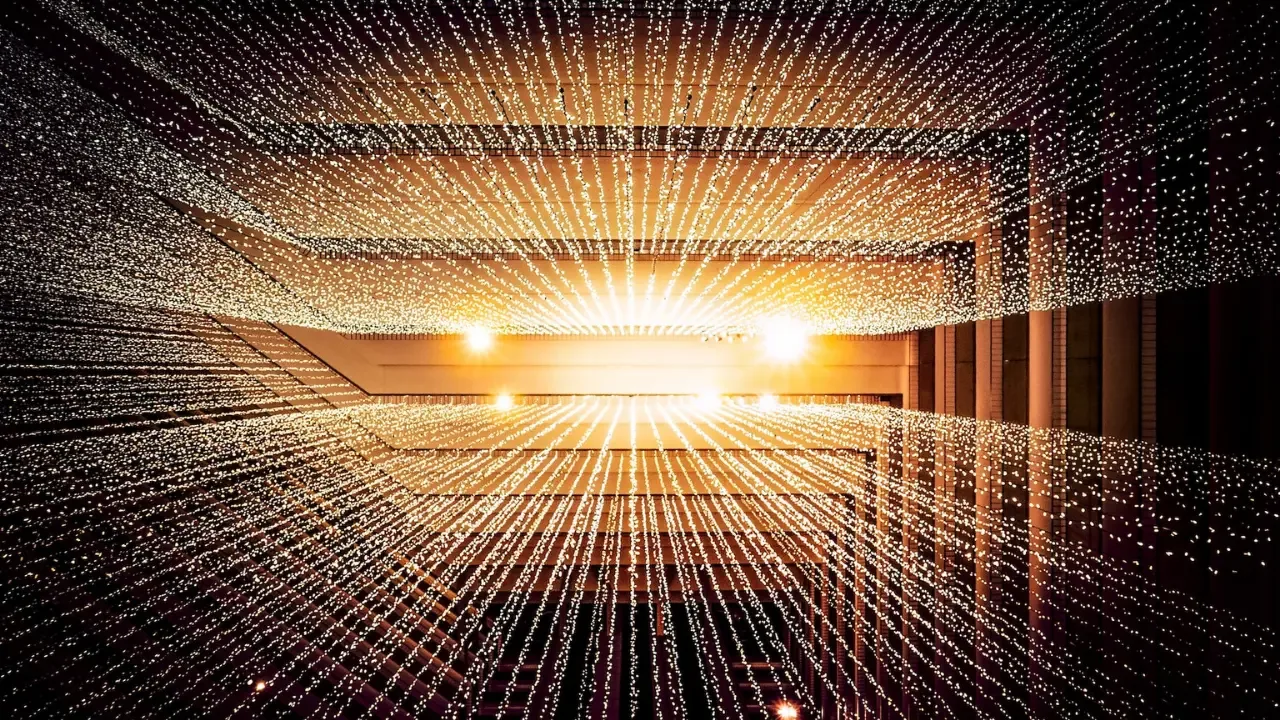
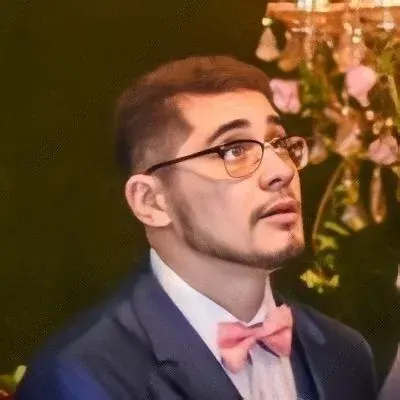
🎉 Creating Abstract Classes in Objective-C: A Complete Guide
Are you a Java programmer who recently dived into the world of Objective-C? 😱 Are you missing the abstract classes you used to work with? We feel you! 🤓
Abstract classes are a powerful tool for organizing code and defining common behavior that can be inherited by other classes. 🚀 Although Objective-C doesn't have a built-in support for abstract classes like Java, fear not! 😎 In this blog post, we'll explore how close you can get to an abstract class in Objective-C and provide you with easy solutions to overcome this hurdle. Let's get started! 💪
✨ The Challenge: Abstract Classes in Objective-C
The concept of abstract classes doesn't exist in Objective-C, but we can mimic their behavior using a combination of techniques. 🎭 Here are a few approaches to consider:
1. Inheritance with No Implementation
To create an abstract class in Objective-C, you can start by defining a base class that contains no implementation details. This class will serve as a blueprint for other classes to inherit from. 🏗️ The methods in this class will be declared but not implemented.
@interface AbstractClass : NSObject
- (void)someMethod; // No implementation
@end
2. Using Protocols
Protocols in Objective-C allow you to define a set of methods that a class must implement. You can leverage protocols to achieve similar functionality to abstract classes. 🔄
@protocol AbstractClassProtocol <NSObject>
- (void)someMethod;
@end
Then, create a concrete class that adopts this protocol and implements the required methods:
@interface ConcreteClass : NSObject <AbstractClassProtocol>
@end
@implementation ConcreteClass
- (void)someMethod {
// Implementation
}
@end
3. Combination of Inheritance and Protocols
An alternative approach is to combine inheritance with protocols to create a stronger abstraction. 🤝 This allows you to define a base class with common implementation details and protocols directly on the base class.
@protocol AbstractClassProtocol <NSObject>
- (void)someMethod;
@end
@interface AbstractClass : NSObject <AbstractClassProtocol>
@end
You can then create concrete classes that inherit from the abstract class and implement the necessary methods.
🎯 Take it to the Next Level
While these approaches might not offer the strictness of abstract classes in other languages, they provide us with flexible alternatives in Objective-C. But don't just stop here! Embrace the power of Objective-C and explore how these techniques can be applied to your projects. 🔍
To level up your Objective-C skills and learn more about advanced programming techniques, consider checking out our blog and joining our community of passionate developers. We'd love to have you on board! 🚀
In conclusion, while Objective-C might not have native support for abstract classes, you can create abstract-like behavior using a combination of inheritance and protocols. By employing these techniques, you'll be able to organize your code effectively, promote code reuse, and achieve cleaner and more maintainable codebases. Happy coding! 🎉
Do you have any other questions or have unique approaches to tackle this problem? Share in the comments below! We're excited to hear from you. 👇