Convert NSDate to NSString
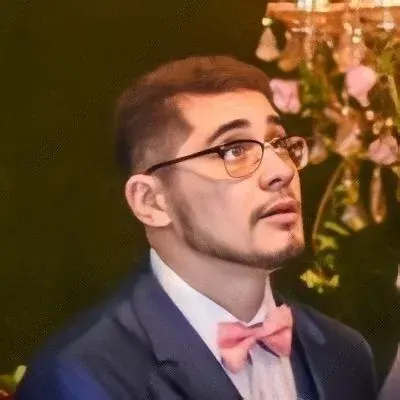
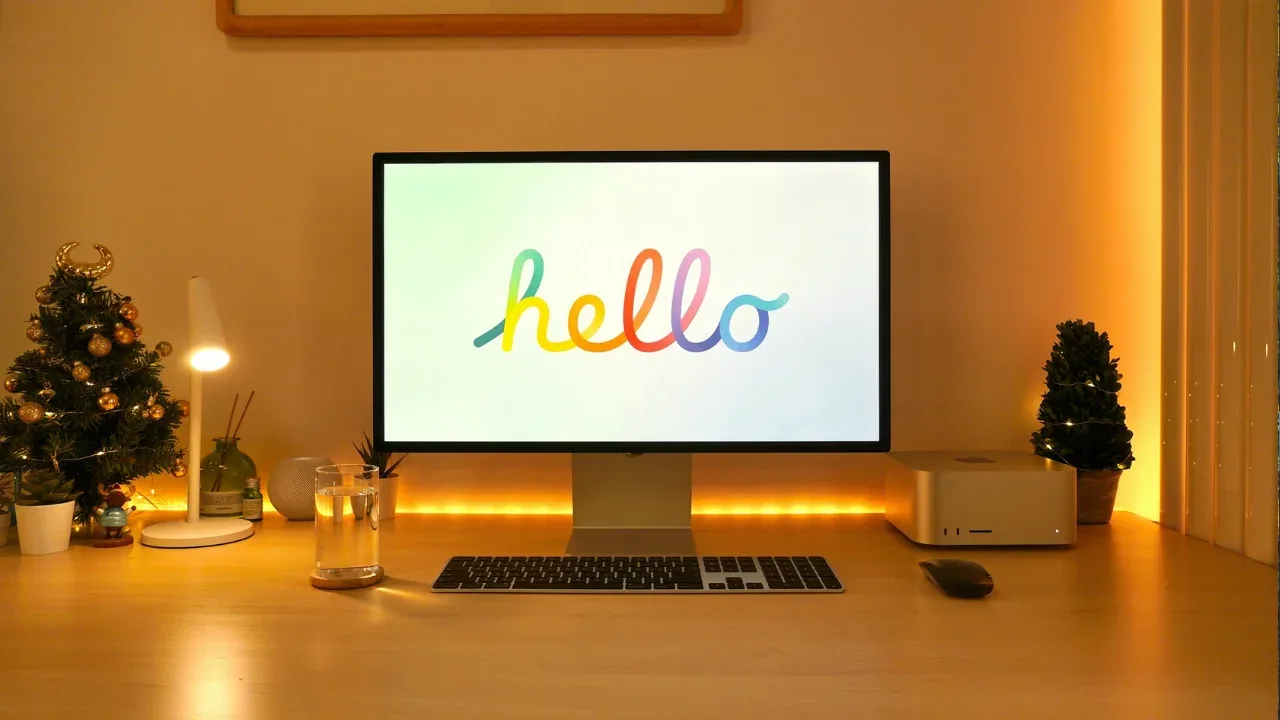
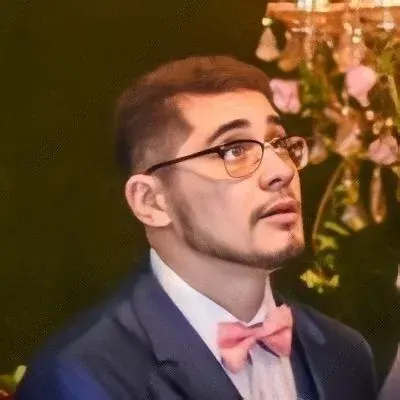
Converting NSDate to NSString: A Handy Guide
Have you ever found yourself in a situation where you have an NSDate
object, but you only need to extract the year and represent it as an NSString
in the format @"yyyy"
? Don't worry, you're not alone! Many developers encounter this common challenge when working with dates in their iOS applications.
In this blog post, we will explore this specific problem and provide you with easy-to-implement solutions to convert NSDate
to NSString
with just the year component. So, let's dive into the code and find out how to achieve this!
Common Pitfalls: Why is it Tricky?
The challenge lies in the fact that an NSDate
object carries a lot more information than just the year. It encapsulates the year, month, day, hour, minute, and second components. Extracting only the year and converting it to an NSString
requires a bit of manipulation.
Additionally, the NSDateFormatter
class provides us with a convenient way to convert between NSDate
and NSString
. However, if not used correctly, it can lead to unexpected results or errors.
Solution #1: Manual Conversion
Let's start with a basic solution that involves manual conversion. We'll leverage the NSCalendar
and NSDateComponents
classes to extract the year component from the NSDate
object.
NSDate *date = [NSDate date];
NSCalendar *calendar = [NSCalendar currentCalendar];
NSDateComponents *components = [calendar components:NSCalendarUnitYear fromDate:date];
NSInteger year = components.year;
NSString *yearString = [NSString stringWithFormat:@"%ld", (long)year];
In this code snippet, we first get the current date using [NSDate date]
. Then, we create an instance of the NSCalendar
class, allowing us to extract specific components from the date. Next, we use NSCalendarUnitYear
to specify that we only want the year component.
After extracting the year, we convert it to an NSString
using [NSString stringWithFormat:]
. This format string contains %ld
as a placeholder for the long
variable.
Solution #2: NSDateFormatter to the Rescue
While Solution #1 works perfectly fine, it involves manual manipulation and isn't as elegant. Fortunately, NSDateFormatter
comes to the rescue and provides us with a cleaner and more efficient approach.
Here's how you can use NSDateFormatter
to achieve the desired result:
NSDate *date = [NSDate date];
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateFormat:@"yyyy"];
NSString *yearString = [formatter stringFromDate:date];
In this solution, we create an instance of NSDateFormatter
and set its format to @"yyyy"
. This format specifies that we only want the year representation. We then use [formatter stringFromDate:]
to convert the NSDate
object to an NSString
with the desired format.
The Choice is Yours!
You now have two easy solutions at your disposal to convert an NSDate
object to an NSString
with only the year component. Whether you choose the manual approach or opt for NSDateFormatter
, the decision ultimately depends on your personal preference and the specific needs of your application.
Now that you have the knowledge, go ahead and implement the solution that best suits you. Make your code cleaner, your life easier, and join the club of developers who have conquered the art of converting NSDate
to NSString
!
If you found this blog post helpful, be sure to share it with your fellow developers. Let them know that converting NSDate
to NSString
can be a piece of cake! Have any questions or alternative solutions? Drop a comment below and let's start a conversation. Happy coding! 😄👩💻👨💻
For more helpful tips and tricks, follow our blog and stay tuned for future updates!
Cover photo by Pexels