Block Declaration Syntax List
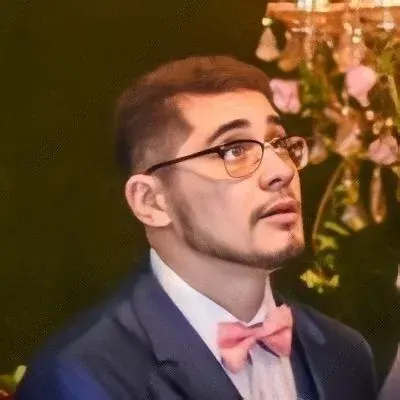
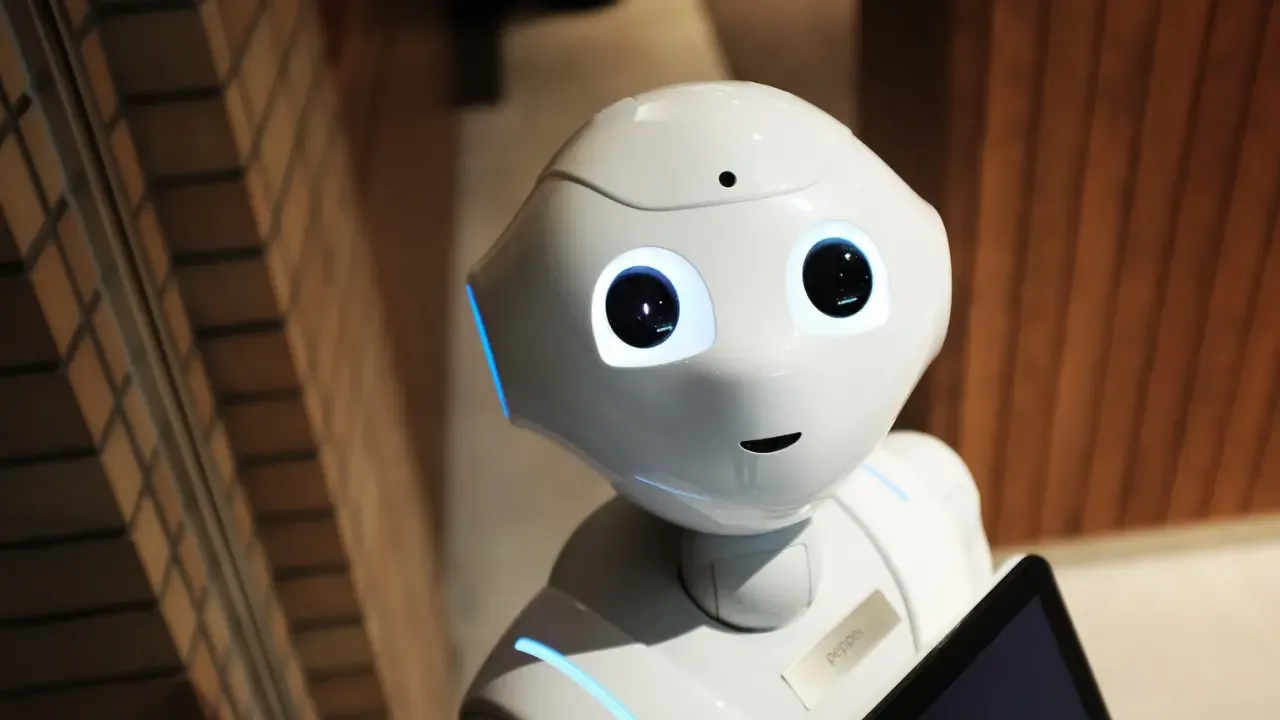
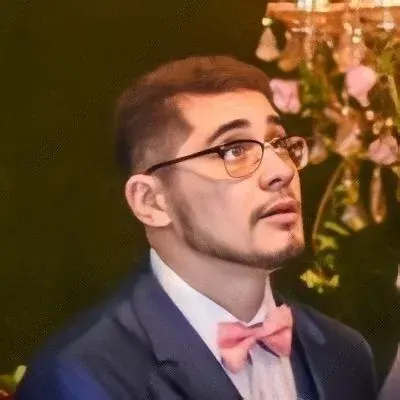
š Blog Post: Unraveling the Mystery of Block Declaration Syntax
š Hey there tech enthusiasts and budding Objective C developers! Are you feeling baffled by the convoluted world of block declaration syntax? Don't worry, you're not alone! š
In the realm of Objective C (and even C), the syntax for declaring blocks can be quite perplexing. š¤ Passing blocks as arguments may look different from declaring them as instance variables, and typedef'ing blocks can add another layer of confusion. But fear not, because I've got your back! š
š The Comprehensive Block Declaration Syntax List
To save you from reaching for the aspirin every time you encounter block declaration syntax, I've compiled a comprehensive list for quick reference. Here we go:
Basic Block Declaration
returnType (^blockName)(parameterTypes) = ^returnType(parameters) { // Block code goes here };
Passing Blocks as Arguments
returnType (^blockName)(parameterTypes) = ^(parameterTypes parameters) { // Block code goes here };
Declaring Blocks as Instance Variables or Properties
@property (nonatomic, copy) returnType (^blockName)(parameterTypes);
Typedef'ing Blocks
typedef returnType (^BlockType)(parameterTypes); BlockType blockName = ^(parameterTypes parameters) { // Block code goes here };
There you have it, a concise yet comprehensive list of block declaration syntax! šāØ
š” Easy Solutions for Common Issues
Now that you have a handy reference for block declaration syntax, let's tackle some common issues you may encounter and provide easy solutions.
š§ Issue 1: Forgot to Make Blocks Nullable
Problem: You're getting a compile-time error stating that a block is missing a nullability specifier.
Solution: Simply add the nullability specifier to the block declaration like this:
returnType (^blockName)(parameterTypes) __nullable = ^returnType(parameters) {
// Block code goes here
};
š§ Issue 2: Block is Not Capturing Variables Correctly
Problem: You're experiencing unexpected behavior because your block is not capturing variables as expected.
Solution: Use the __block
storage type qualifier when declaring variables that need to be captured by the block, like this:
__block returnType capturedVariable = initialValue;
returnType (^blockName)(parameterTypes) = ^(parameterTypes parameters) {
// Use the capturedVariable here
};
š§ Issue 3: Block is Causing Retain Cycles
Problem: Your blocks are causing retain cycles, leading to memory leaks.
Solution: Weakify objects within the block using a weakSelf
reference to avoid retain cycles, like this:
__weak returnType weakSelf = self;
returnType (^blockName)(parameterTypes) = ^(parameterTypes parameters) {
returnType strongSelf = weakSelf;
// Use strongSelf here without causing a retain cycle
};
š£ Join the Block Declaration Syntax Sleuths!
Now that you're equipped with a comprehensive list of block declaration syntax and easy solutions to common issues, it's time to put it to the test! šŖ I challenge you to unleash your coding skills and become a master of block syntax.
Share your block declaration triumphs, epic fails, and valuable tips in the comments section below. Let's learn and grow as a community of tech aficionados! šš¬
So go ahead, bookmark this blog post, and never fear the enigmatic realm of block declaration syntax again! š
Happy coding! šš»
Note: Remember to replace returnType
and parameterTypes
with the actual return type and parameter types of your block.