Best way to define private methods for a class in Objective-C
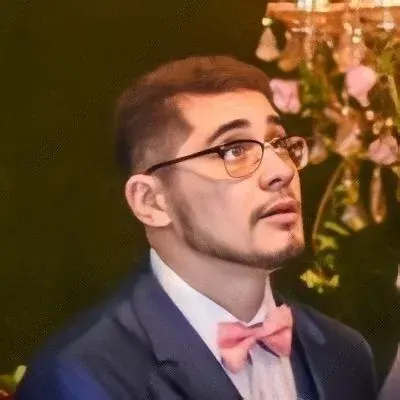
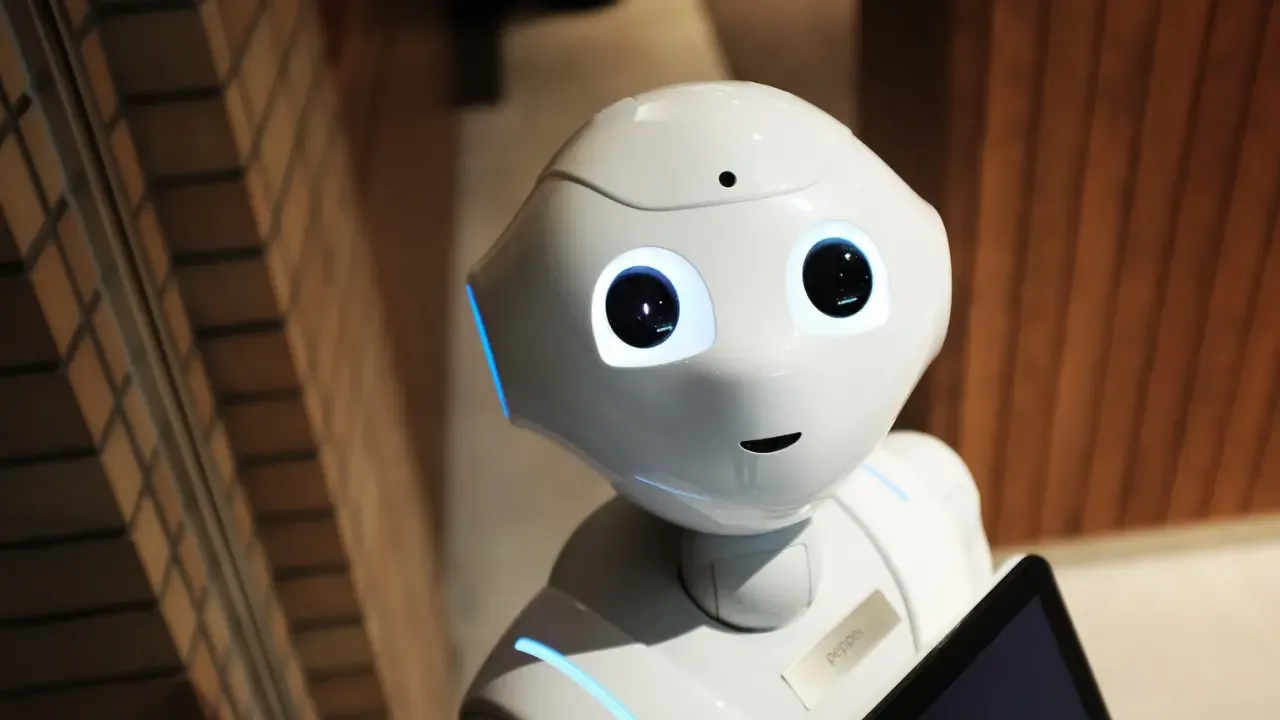
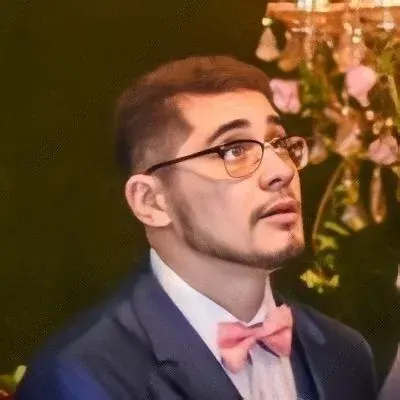
The Best Way to Define Private Methods for a Class in Objective-C 😎
So, you just started programming Objective-C and want to know how to deal with private methods? Good news! I've got you covered. 🙌
Understanding the Challenge 🧐
In Objective-C, private methods are not explicitly defined like in some other programming languages. However, there are some conventions and habits that people in the Objective-C community follow to achieve the same result.
The Aggregated Best Techniques 💡
After researching and gathering insights from experienced Objective-C developers, I found a couple of techniques that are widely used. Let's explore them:
1. Utilize Categories to Group Private Methods 📚
One common approach is to use categories to group private methods within the implementation file (e.g., MyClass.m
).
// MyClass.h (public interface)
@interface MyClass : NSObject
// Public methods
@end
// MyClass.m (implementation)
@interface MyClass (Private)
// Private method declarations
@end
@implementation MyClass
// Public method implementations
@end
@implementation MyClass (Private)
// Private method implementations
@end
This technique allows you to separate your private methods from the public ones, making your code more organized and easier to understand. However, there are a few issues to be aware of:
Issue #1 - Missing Method Definitions: The Xcode compiler does not check if you define all methods in the private category (
MyClass (Private)
) within the corresponding@implementation
block. Be careful not to miss any!Issue #2 - Ordering Requirements: To avoid Xcode complaints, you need to put the
@interface
declaration for the private category at the beginning of theMyClass.m
file. This can be frustrating if you prefer having your private methods implemented and defined near the end of the file.
2. Leverage Empty Categories for Better Control 🗂️
To address Issue #1 mentioned above, you can use an empty category (MyClass ()
) to define your private methods:
// MyClass.h (public interface)
@interface MyClass : NSObject
// Public methods
@end
// MyClass.m (implementation)
@interface MyClass () // Empty category
// Private method declarations
@end
@implementation MyClass
// Public method implementations
@end
@implementation MyClass (Private) // Optional separate category may be declared after public @implementation
// Private method implementations
@end
By using an empty category, you can ensure that all private method declarations are implemented, avoiding any compilation errors. Plus, this allows you to maintain the desired order of your private method definitions within the file.
Conclusion 🎉
Both the category grouping and empty category techniques provide solutions for defining private methods in Objective-C. Ultimately, the best approach will depend on your personal preference and project requirements.
Now that you have a better understanding of how to define private methods in Objective-C, it's time to put it into practice! Experiment with these techniques and see which one works best for you.
Share your experiences with us in the comments section below. We'd love to hear your thoughts and learn from your insights. Let's master Objective-C together! 😉