Best practices for overriding isEqual: and hash
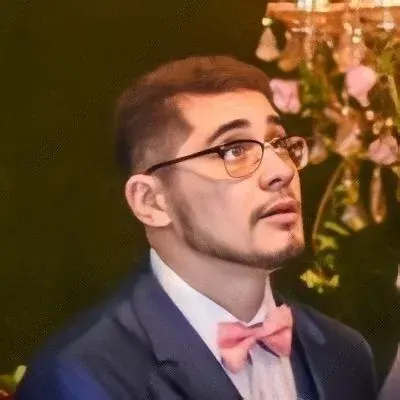
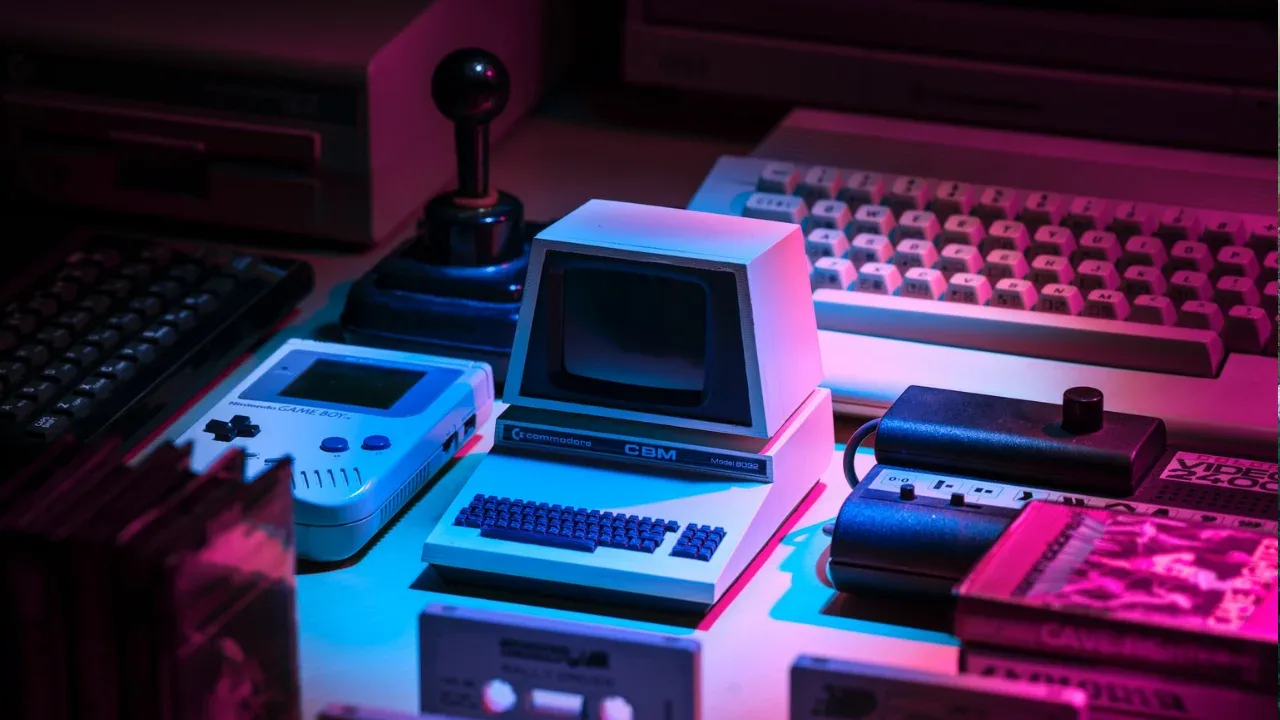
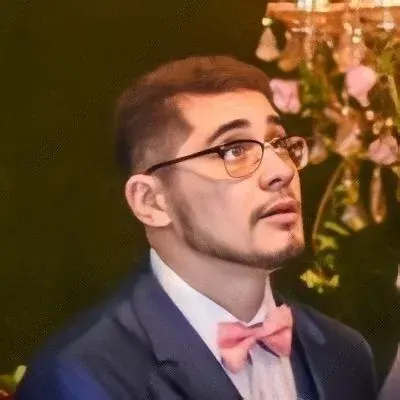
Best Practices for Overriding isEqual:
and hash
🤝🔍
Are you having trouble properly overriding isEqual:
in Objective-C? 😕 Don't worry, you're not alone! The "catch" is that if two objects are considered equal according to the isEqual:
method, they must also have the same hash value. 😮 In this blog post, we'll address common issues and provide easy solutions to help you master this aspect of Objective-C. 😉 Let's get started! 💪
Understanding isEqual:
Before we dive into the details of overriding isEqual:
and hash
, let's quickly recap what isEqual:
does. 🔄 The isEqual:
method is used to determine if two objects are equal. By default, it checks for pointer equality (i.e., whether the two objects are the exact same instance). But in most cases, you'll want to override this method to provide custom equality comparison based on your object's properties. ✨
- (BOOL)isEqual:(id)other {
if (other == self)
return YES;
if (!other || ![other isKindOfClass:[self class]])
return NO;
return [self isEqualToWidget:other];
}
- (BOOL)isEqualToWidget:(MyWidget *)aWidget {
if (self == aWidget)
return YES;
if (![(id)[self name] isEqual:[aWidget name]])
return NO;
if (![[self data] isEqualToData:[aWidget data]])
return NO;
return YES;
}
In the given example, isEqual:
first checks for pointer equality, then verifies class equality, and finally delegates the equality comparison to the isEqualToWidget:
method. This method compares the name
and data
properties to determine if two MyWidget
objects are equal. 🔀
Overriding hash
Now that we've covered isEqual:
, let's tackle the issue of overriding hash
. 😅 By default, objects in Objective-C use their memory addresses as hashes. However, when you override isEqual:
, you also need to override hash
to ensure consistency. The hash
method should return an integer that represents the object's identity. 🆔
To override hash
, you should consider the properties that affect the equality comparison. In the given example, name
and data
are the properties that determine equality. Therefore, we want the hash to be influenced by these properties only. 💡 Here's one way to implement it:
- (NSUInteger)hash {
NSUInteger hash = 0;
hash += [[self name] hash];
hash += [[self data] hash];
return hash;
}
In this implementation, we create a variable called hash
and add the hashes of the name
and data
properties to it. This ensures that if two objects are equal, their hash values will also be equal. 🤝
Handling Primitives and Structs
What about properties that aren't objects, like int
or NSRect
? 🤔 You may be tempted to convert them to NSNumber
or some other object to obtain a hash. However, this isn't necessary. Since the built-in scalar types and structs provide their own hash
implementations, you can simply use them. The resulting hash will be based on the actual values of these properties.
For example, if you have an int
property called age
, you can include it in the hash
calculation like this:
- (NSUInteger)hash {
NSUInteger hash = 0;
hash += [[self name] hash];
hash += [[self data] hash];
hash += self.age; // Include the value of the 'age' property
return hash;
}
Similarly, if you have a NSRect
struct property, you can include it as follows:
- (NSUInteger)hash {
NSUInteger hash = 0;
hash += [[self name] hash];
hash += [[self data] hash];
hash += NSStringFromRect(self.rect).hash; // Include string representation of 'rect'
return hash;
}
By including these properties in the hash
calculation, you ensure that changes in their values will affect the overall hash of the object, enabling consistent equality comparisons. 😊
Wrapping Up
Congratulations! 🎉 You've learned the best practices for overriding isEqual:
and hash
in Objective-C. By ensuring that your objects have consistent equality comparisons and hash values, you'll avoid pitfalls and make your code more robust. Remember, when overriding isEqual:
, always override hash
as well to maintain consistency. 💪
Now it's time to put your newfound knowledge into practice! ✨ Go ahead and update your classes to override isEqual:
and hash
using the techniques we discussed. If you have any questions or want to share your experiences, feel free to leave a comment below! Let's engage in a conversation about best practices for equality in Objective-C. 🗣️💬 Together, we can write better code! 💻🚀