What"s the difference between process.cwd() vs __dirname?
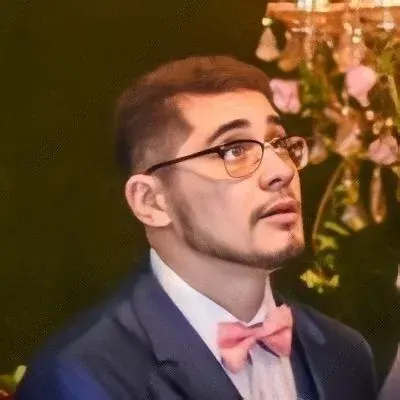
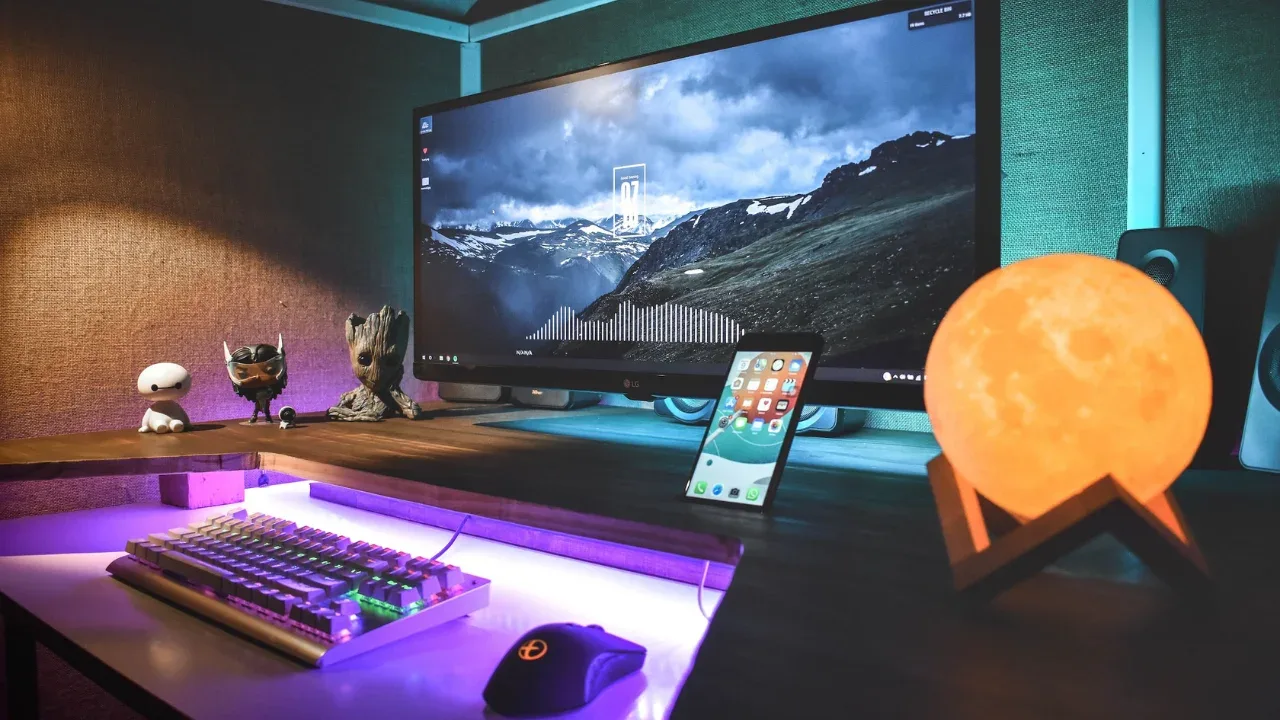
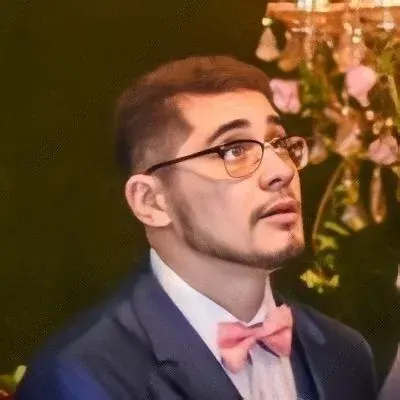
📝 Title: Navigating the Path Maze: Understanding process.cwd() vs __dirname
Hey there, tech enthusiasts! 👋 Are you constantly baffled by JavaScript's path-related functions? 🤷♀️ Today, we're diving into one of the most perplexing questions: What's the difference between process.cwd()
and __dirname
? 🤔 Stay tuned to unravel this mystery! 🔍
Common Issues: What Are These Functions?
Both process.cwd()
and __dirname
are widely used in JavaScript to retrieve the current working directory and the directory of the current module, respectively.
process.cwd()
When you use process.cwd()
, you're basically asking Node.js to give you the current working directory of the Node.js process. It returns an absolute path as a string. 😮
Here's an example:
console.log(process.cwd()); // /User/johndoe/Documents
__dirname
On the other hand, __dirname
provides the directory path of the current module file, which can be handy when you need to manipulate files relative to the module's location. Like process.cwd()
, it returns an absolute path as a string. 😎
Check out this example:
console.log(__dirname); // /User/johndoe/Documents/blog
Now that we understand what they do, let's explore the key differences between these two functions! 🧐
The Key Differences Explained
Point of Reference:
process.cwd()
is relative to where the process was launched. It can be different from the directory where your script resides. 🚀__dirname
is the directory path of the current module file itself. It remains constant regardless of where the process was launched. 📂
Usage in Module Exports:
When using
process.cwd()
within a module'sexports
(e.g.,module.exports = function() { console.log(process.cwd()); }
), the current working directory will be resolved based on the location where the module was required. 🌐In contrast,
__dirname
within module exports will always contain the directory path of the module itself, irrespective of where it's required. 📁
Now that we've covered the main differences, you can avoid confusion and make informed decisions about which function suits your specific needs. 🙌
Handy Solutions: How to Use Them
To better understand their effectiveness, let's explore a couple of use cases:
Case 1: File Manipulation
When dealing with file manipulation, __dirname
can be incredibly useful. Suppose you want to read a file relative to the module's location:
const fs = require('fs');
const path = require('path');
const filePath = path.join(__dirname, 'myFolder', 'myfile.txt');
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Case 2: Cross-Platform Scripts
When writing cross-platform scripts that require the use of absolute paths, process.cwd()
is your best friend. Consider this scenario:
const configPath = path.join(process.cwd(), 'config', 'settings.json');
// Now you can access configPath from any location where this script is executed 🌍
By using these functions in the appropriate context, you can navigate through the path maze with ease! 🚶♂️
Engage With Us
We hope this blog post has shed some light on the nuances of process.cwd()
and __dirname
. Reach out to us with your own use cases or any lingering questions we didn't address! Let's engage in a vibrant discussion in the comments section below. Let's crack the code together! 💪🔑💬
Happy coding! 💻✨