What is the difference between __dirname and ./ in node.js?
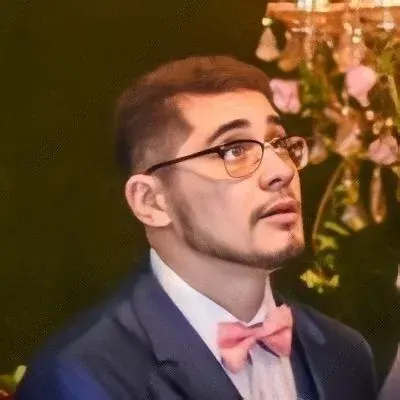
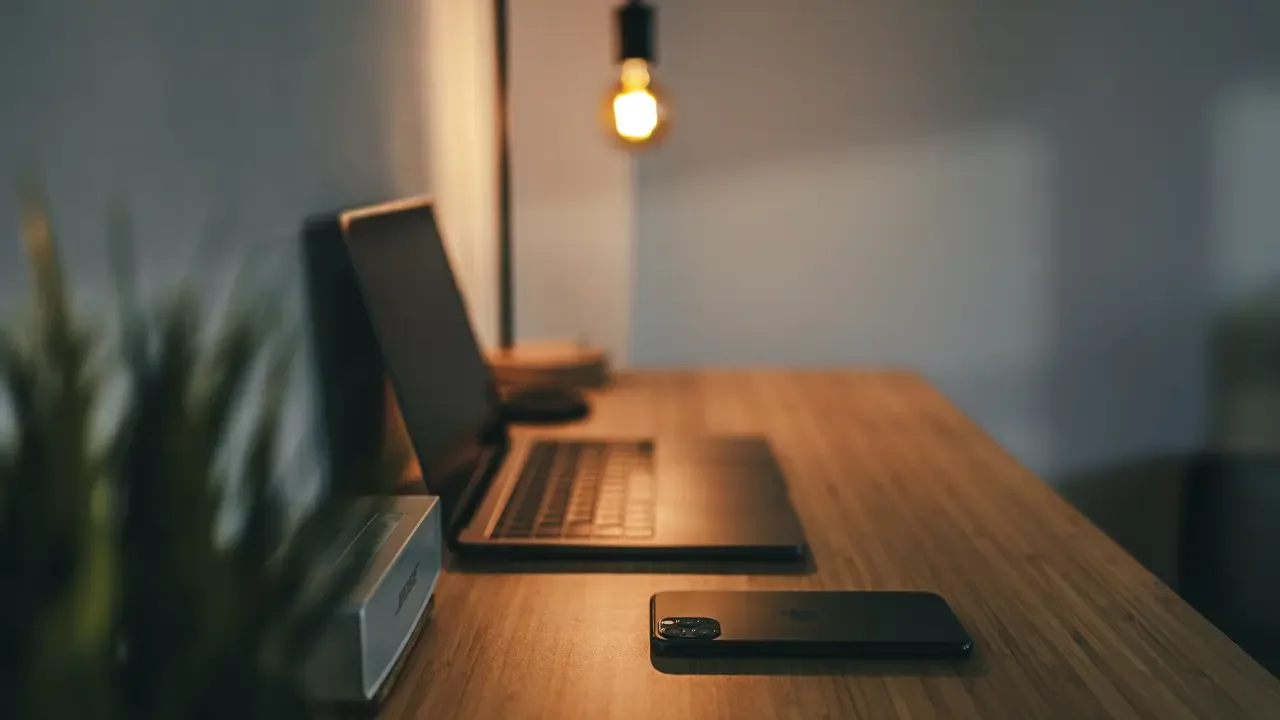
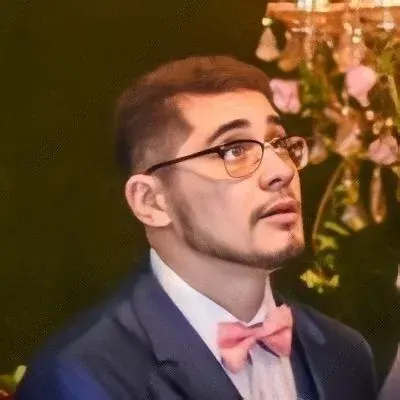
What's the Difference Between __dirname and ./ in Node.js? π€
Introduction
So you're coding in Node.js and suddenly come across the terms __dirname
and ./
. You can't help but wonder, what's the difference? π€·ββοΈ Don't worry, you're not alone! Many developers have faced this same question. In this post, we'll explain the difference between __dirname
and ./
and help you understand when to use each one. Let's dive in! πββοΈ
Understanding the Problem
Before we get into the specifics, let's make sure we understand the problem at hand. When referencing files in Node.js, you might need to provide the path to those files. The question is, should you use __dirname
or ./
for the path? Let's break it down. π§©
The Current Working Directory (CWD) π
To understand the difference between __dirname
and ./
, we first need to understand the concept of the Current Working Directory (CWD). The CWD is the directory from which your Node.js process is running. When you run your script, the CWD is set to the location of your script. Keep this in mind as we explore further. π€
__dirname π
__dirname
is a global variable in Node.js that represents the directory of the current module. It gives you the absolute path of the directory containing the file in which __dirname
is used. πΊοΈ
Here's an example:
console.log(__dirname);
If you run this code, it will print the absolute path of the current module's directory. So if your script is located at /home/user/myproject/myscript.js
, __dirname
would resolve to /home/user/myproject
. π
./ (Dot Slash) π©
On the other hand, ./
represents the relative path from the current module's directory. It allows you to navigate through the file system in relation to the current directory. πΆββοΈ
Let's consider an example:
const someFile = require('./myfile');
Here, ./myfile
is referring to a file called myfile.js
located in the same directory as the current module. If the file is in a different directory, you would need to modify the relative path accordingly. π§
When to Use __dirname and ./ π―
Now that we understand the difference between __dirname
and ./
, let's discuss when to use each one. It all boils down to whether you need an absolute or relative path. π€
Use __dirname
when you need an absolute path to a file or directory. This is useful when you want to reference files located in a fixed location, regardless of the current working directory. For example:
const filePath = path.join(__dirname, 'data', 'file.txt');
Using __dirname
ensures that file.txt
is always referenced from the same directory, even if your script is executed from a different location. π
On the other hand, use ./
when you need a relative path to a file or directory. This is useful when you want to reference files located in the same directory as your current module or in a specific relative position. For example:
const someFile = require('./myfile');
Here, myfile.js
is located in the same directory as the current module, so we use ./
to indicate the file's relative path. π
Conclusion and Call-to-Action β
By now, you should have a clear understanding of the difference between __dirname
and ./
in Node.js. Remember, __dirname
provides an absolute path to the current module's directory, while ./
represents a relative path from the current module's directory. Choose the appropriate approach based on whether you need an absolute or relative path for your specific use case. Happy coding! π»
Now it's your turn! Have you ever encountered any quirks or challenges with directory paths in Node.js? Share your experiences or any additional questions in the comments below. Let's help each other out! π§βπ»