using process.env in TypeScript
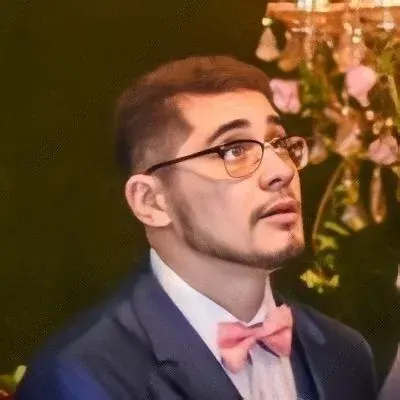
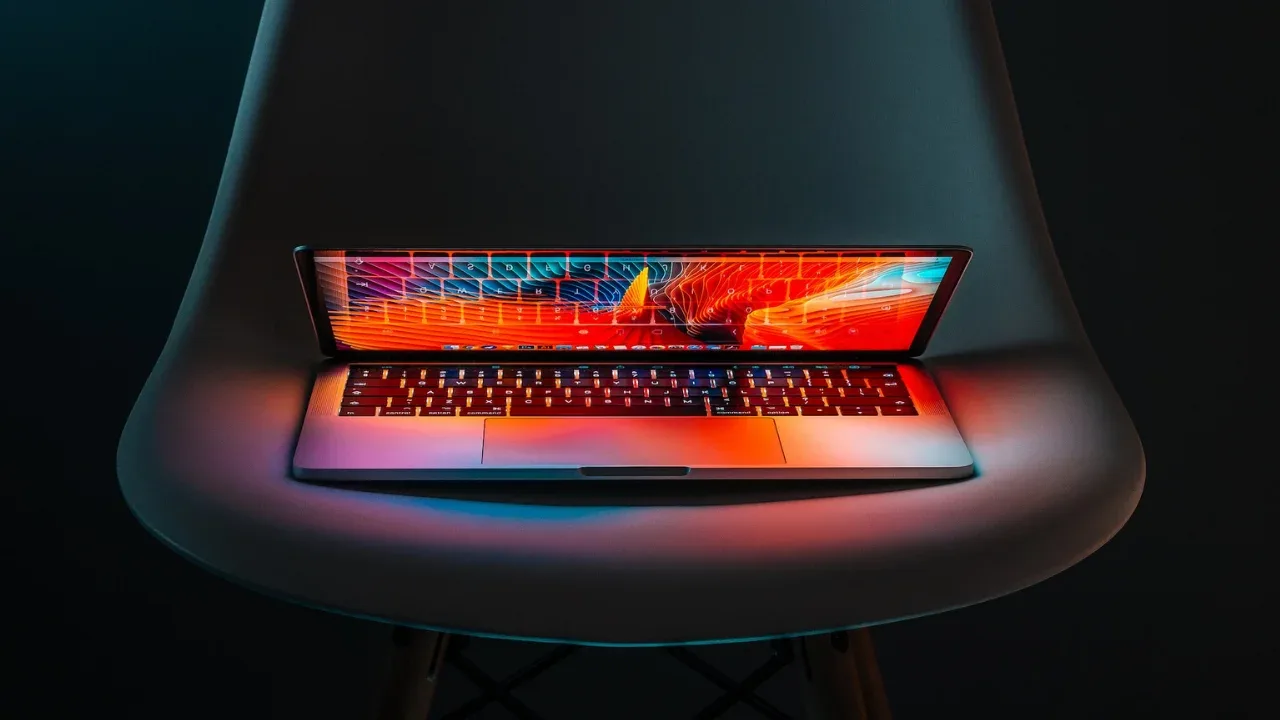
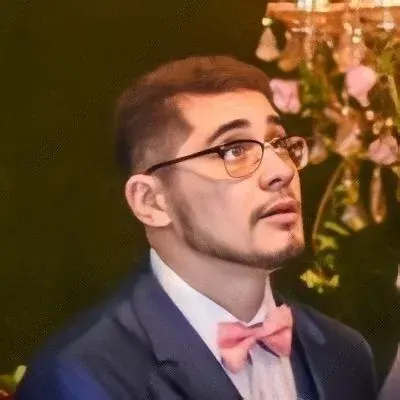
Using process.env
in TypeScript: How to Solve the 'Property does not exist' Error
So you're working on a TypeScript project and need to read node environment variables? 🌳 Environment variables are a great way to store sensitive or dynamic information, but integrating them into your TypeScript code may sometimes present challenges.
One common issue you may encounter is the error message:
Property 'NODE_ENV' does not exist on type 'ProcessEnv'
😱 Don't panic! In this blog post, we'll address this specific problem and provide easy solutions so you can smoothly access and use process.env
in your TypeScript code. Let's jump right in! 🚀
🛠️ The Problem
Specifically, you mentioned that you installed @types/node
but still encountered the error. This error occurs because TypeScript, by default, doesn't recognize the properties of process.env
and its type ProcessEnv
, which leads to the error message you encountered. But worry not, there are a couple of ways to fix this!
💡 Solution 1: Changing TypeScript Configuration
The first solution involves tweaking your TypeScript configuration file (tsconfig.json
). Locate this file in the root directory of your project and open it in your favorite text editor.
In the compilerOptions
section, add the following line:
"types": ["node"]
This informs the TypeScript compiler to include the typings for the Node.js environment. Save the file, and you're good to go. TypeScript should now recognize process.env
and its properties without any errors.
💡 Solution 2: Type Assertion
If you don't want to modify your TypeScript configuration, or if you prefer a different approach, you can use a type assertion to bypass the error.
Instead of directly accessing process.env.NODE_ENV
, you can assert it as a string:
const nodeEnv = process.env.NODE_ENV as string;
By asserting the type as string
, you let TypeScript know that you want to treat process.env.NODE_ENV
specifically as a string. This way, you won't encounter any errors when accessing it.
✅ Let's Test It Out!
To verify that the error is resolved, let's try running a simple code snippet:
// Assuming you have an environment variable named 'MY_VARIABLE'
const myVariable = process.env.MY_VARIABLE as string;
console.log(`The value of MY_VARIABLE is: ${myVariable}`);
Save this code in a TypeScript file, and then compile and run it using your preferred tooling (e.g., tsc
and node
). If everything's working correctly, you should see the value of your environment variable displayed in the console!
🎉 Get Involved!
We hope this blog post helped you solve the 'Property does not exist' error when using process.env
in TypeScript. Now it's your turn to get involved!
📢 Share this blog post with fellow developers who might be facing the same issue. Let's spread the knowledge and make development smoother for everyone!
💬 Have any other TypeScript-related questions or issues? Leave a comment below, and our vibrant community will be more than happy to assist you!
Let's keep coding and exploring the wonderful world of TypeScript together! 💪🔥