Understanding passport serialize deserialize
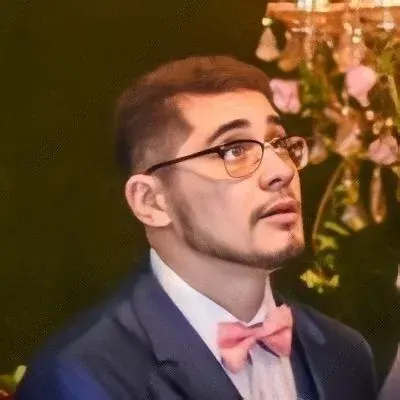
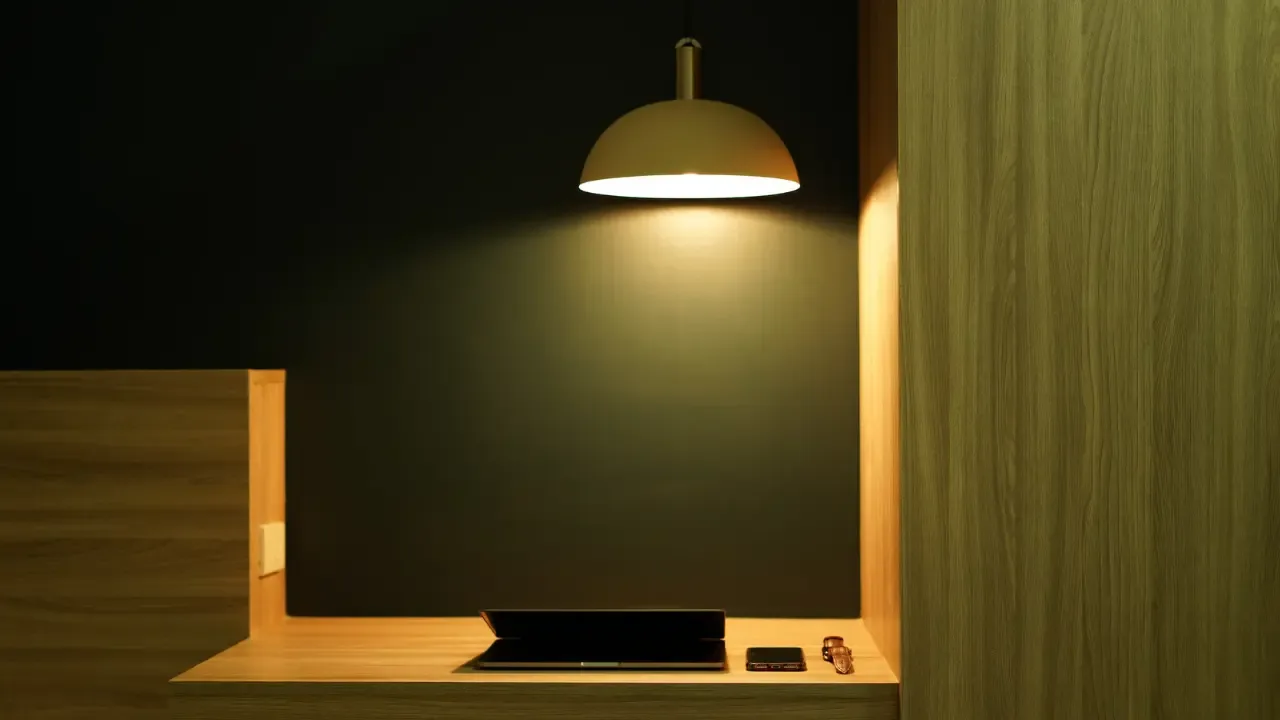
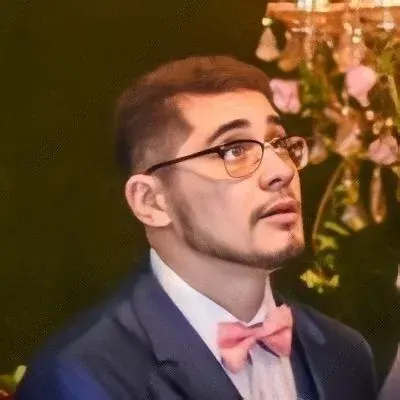
Understanding Passport's serialize and deserialize Methods 📝
Passport is a popular authentication middleware for Node.js that simplifies the process of implementing authentication in web applications. One of the essential components of Passport is the serialize
and deserialize
methods, which allow you to store and retrieve user information during the authentication process.
Let's break it down in a layman's terms! 🤓
Serialize Method 🖊️
The passport.serializeUser
method is called when a user logs in or registers successfully. It takes two parameters: the user
object and a done
function.
Here's an example of a serialize method:
passport.serializeUser(function(user, done) {
done(null, user.id);
});
In simple words, this method decides what piece of information from the user
object should be stored in the session. In this case, we're using the user.id
to uniquely identify the user and store it in the session.
So when the serialize method is called, it saves the user.id
(in this case) to the session store or any other storage mechanism you have configured for your application. It could be a database, file, or memory store.
Deserialize Method 📖
The passport.deserializeUser
method is called whenever a user makes a request to your application after logging in. It takes two parameters: the id
stored in the session and a done
function.
Here's an example of a deserialize method:
passport.deserializeUser(function(id, done) {
User.findById(id, function(err, user) {
done(err, user);
});
});
The deserialize method takes the id
(stored in the session through the serialize process) and retrieves the user information based on that id
. In this example, we're assuming that the User
model has a static method findById
that returns the user object based on the id
provided.
The done
function is used to pass control back to Passport after deserialization completes. If there's an error, we can pass it as the first argument of the done
function. Otherwise, we pass the retrieved user
object.
How They Work Together 🤝
To understand how serialize and deserialize work together, let's consider a scenario:
A user logs in or registers successfully on your application.
Passport's serialize method is called, and it saves the
user.id
to the session store.On subsequent requests from the user, Passport's deserialize method is called first.
The deserialize method retrieves the
id
from the session and uses it to fetch the user object from the database using theUser.findById()
function (or any other retrieval mechanism).The user's object is then attached to the
request
object asrequest.user
, allowing you to access it within your application routes or middleware.
So, in a nutshell, serialize
method stores the necessary user information in the session, and deserialize
method retrieves that information and makes it available for you to use in your application.
Summary ✅
Understanding how Passport's serialize
and deserialize
methods work can be a bit confusing at first, but it's crucial to grasp their significance in the authentication process.
serializeUser
stores relevant user information (e.g.,user.id
) in the session.deserializeUser
fetches the user information from the session based on the storedid
and makes it accessible for further use.
By using these methods, Passport simplifies the process of managing user sessions and authentication in your Node.js applications.
If you have more questions or need further assistance, leave a comment below, and I'll be happy to help! 😊
CALL-TO-ACTION 📣
Do you use Passport in your Node.js applications for authentication? Share your experience and thoughts in the comments. Let's discuss! 💬