Stop Mongoose from creating _id property for sub-document array items
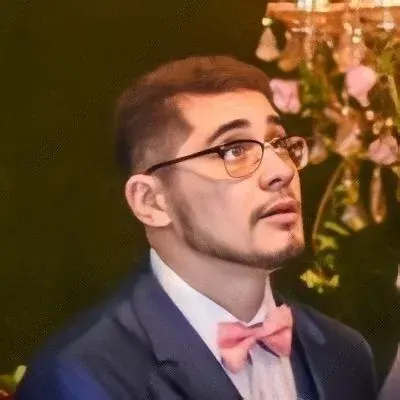
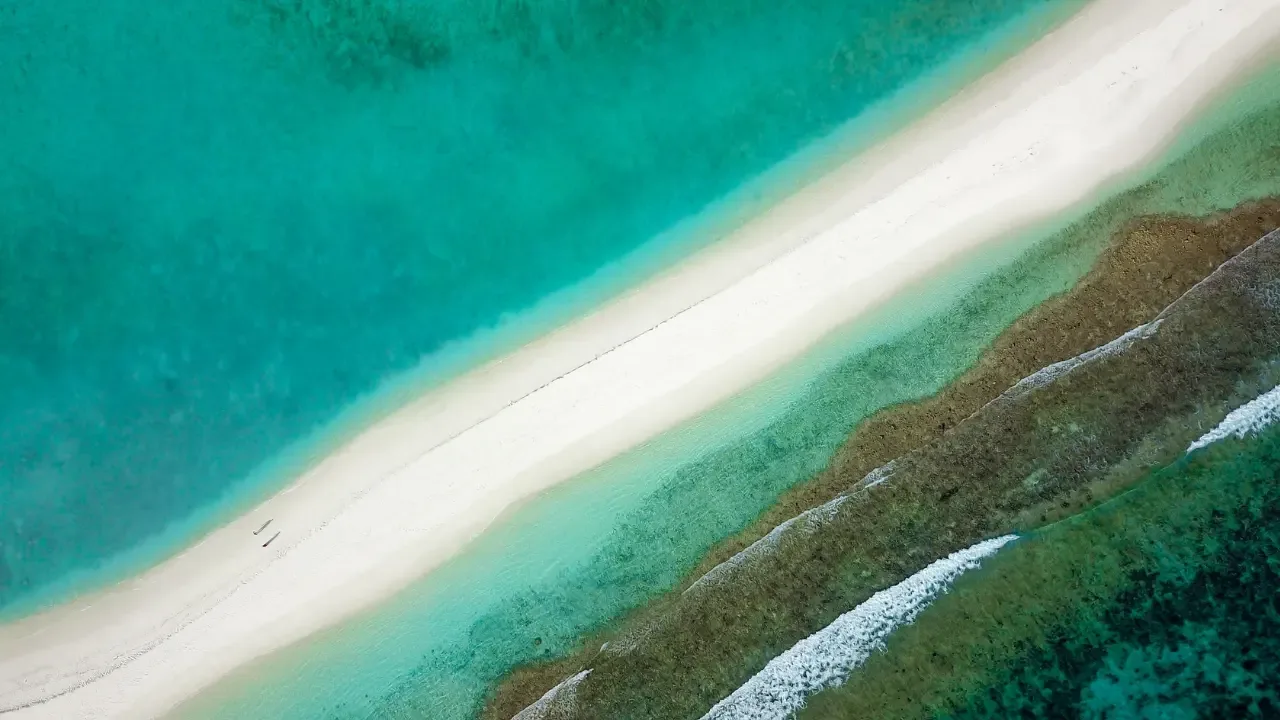
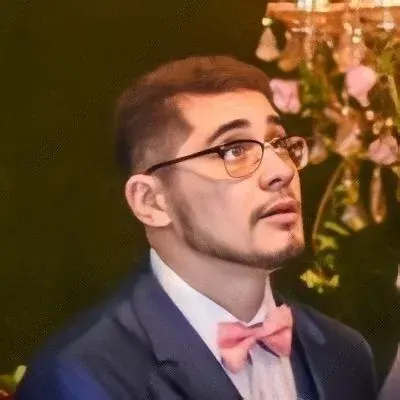
📝🚀 Stop Mongoose from creating _id
property for sub-document array items
Hey tech enthusiasts! Are you struggling with Mongoose automatically creating _id
properties for subdocument array items? 🤔 Don't worry, we've got your back! In this blog post, we'll address this common issue and provide you with easy solutions to stop Mongoose from creating those unwanted ids. Let's dive in! 💪
So, you have subdocument arrays and you noticed that Mongoose automatically generates ids for each one. Here's an example to illustrate the context:
{
_id: "mainId"
subDocArray: [
{
_id: "unwantedId",
field: "value"
},
{
_id: "unwantedId",
field: "value"
}
]
}
Now, the question arises: Is there a way to tell Mongoose to not create ids for objects within an array? Absolutely! Here are a couple of solutions that can help you get rid of those unwanted ids:
Solution 1: Use the id
option
In your Mongoose schema definition, you can utilize the id
option to control the generation of ids for subdocument array items. Simply set it to false
for the specific array you want to exclude the ids from.
const subDocSchema = new Schema({
field: String
}, { _id: false });
const mainDocSchema = new Schema({
_id: String,
subDocArray: [subDocSchema]
});
Voilà! By setting the _id
property to false
in the subdocument schema options, Mongoose won't create ids for objects within the array. 🎉
Solution 2: Use the schema.options
property
You can also achieve the desired behavior by directly modifying the schema.options
property of your subdocument schema.
const subDocSchema = new Schema({
field: String
});
subDocSchema.options._id = false;
const mainDocSchema = new Schema({
_id: String,
subDocArray: [subDocSchema]
});
With this approach, you're modifying the _id
property in the subDocSchema.options
to false
, instructing Mongoose to skip generating ids for array items.
Solution 3: Manually remove the unwanted ids
If you want more granular control, you can manually remove the unwanted ids from the array items after they are created. For instance, you can use the .lean()
method in your query and modify the resulting data before using it.
Model.find().lean().then((docs) => {
docs.subDocArray.forEach((item) => {
delete item._id;
});
console.log(docs);
});
By deleting the _id
property from each subdocument array item, you eliminate those unwanted ids.
📣 Now that you have the power to stop Mongoose from creating those annoying _id
properties for subdocument array items, it's time to level up your development workflow! Try out these solutions and let us know your thoughts in the comments section below. 💬 We'd love to hear from you!
🚀 Happy coding! 👩💻👨💻