req.body empty on posts
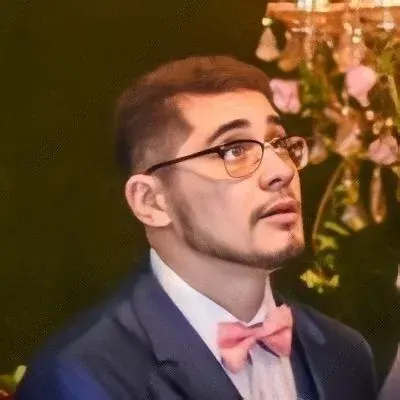
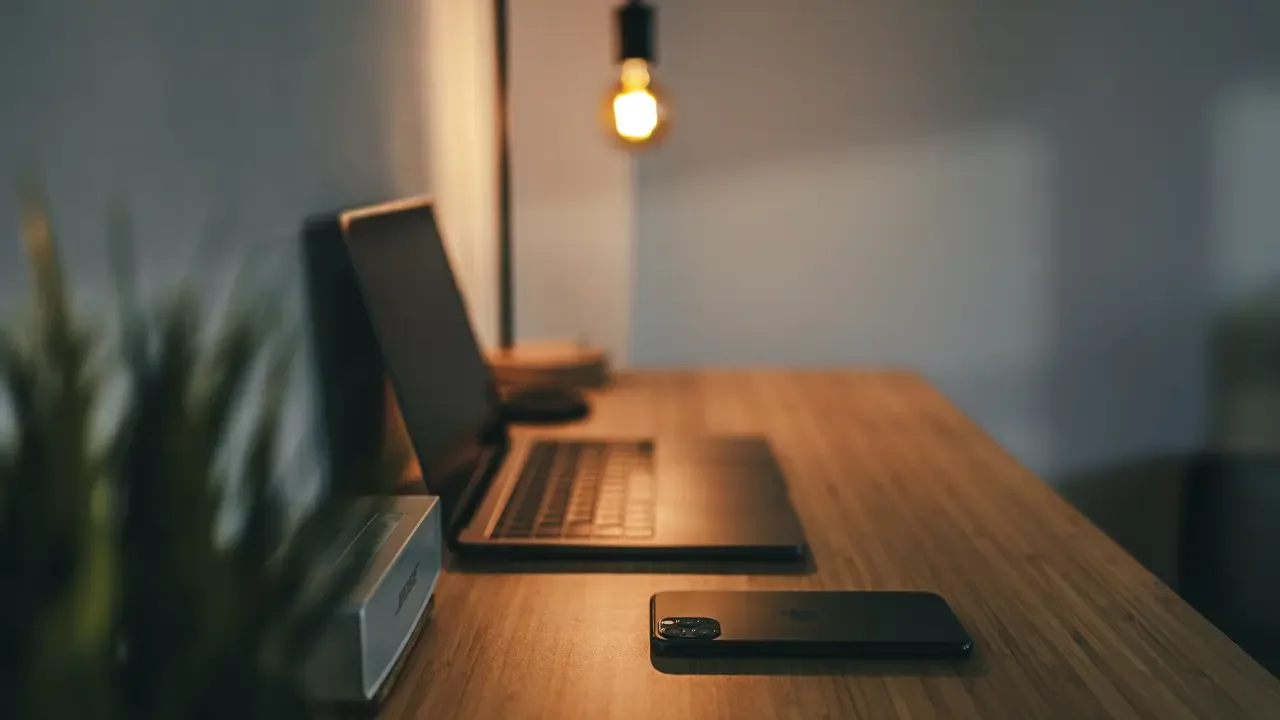
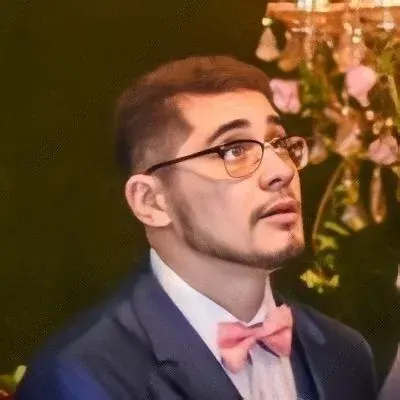
📝 Why is req.body empty on posts?
If you've been experiencing a problem where req.body
is mysteriously empty when making a POST request in Node.js using Express and Body-parser, don't worry, you're not alone! Many developers have encountered this issue, and it can be frustrating when you expect the request body to contain data but find it empty instead. But fear not, we're here to help you understand and resolve this problem once and for all. Let's dive in!
🧐 Understanding the Issue
The first step in solving any problem is understanding it. The code snippet you provided gives us some insight into the issue. In your Express application, you have set up the necessary middleware for parsing incoming requests:
var bodyParser = require('body-parser')
// ...
app.use(bodyParser.urlencoded())
app.use(bodyParser.json())
This configuration should allow you to access the request body via req.body
for both URL-encoded and JSON data. However, you're finding that req.body
is empty when making POST requests, even though it works fine when using cURL.
💡 Possible Solutions
🥤 Content-Type Header
One possible culprit for the empty req.body
is the missing or incorrect Content-Type
header. When making AJAX or Postman requests, you mentioned that the req.body
is empty, but when using cURL with the correct Content-Type
header, it works as intended. This suggests that the issue might be related to the Content-Type
header being sent by the client.
When making requests with AJAX or Postman, make sure to set the Content-Type
header to the appropriate value. For JSON data, the header should be:
Content-Type: application/json
If you're using AJAX, you can set the Content-Type
header like this:
xhr.setRequestHeader("Content-Type", "application/json");
And in Postman, you can select the "application/json" option from the "Body" tab.
🔄 Request Format
Another potential cause for the empty req.body
is the way the request payload is being sent. Double-check that you're sending the data in the correct format, which in this case is JSON. Make sure the request body is a valid JSON object and matches the one you expect on the server.
🔍 Check for Other Middleware Interfering
Sometimes, other middleware in your application can interfere with body-parser
and cause issues. It's worth revisiting your entire middleware stack and checking if there's any other middleware that might be modifying or interfering with the request body. Try temporarily removing other middlewares and see if the issue persists.
⬆️ Update Dependencies
Although you mentioned that you downgraded body-parser
, it's worth ensuring that all your dependencies are up to date, including express
and body-parser
. Outdated dependencies can sometimes cause compatibility issues, so make sure you have the latest versions installed.
📣 Your Input, Your Solution
We hope these possible solutions help you resolve the issue of req.body
being empty on posts. Remember, troubleshooting can be a trial-and-error process, so don't get discouraged if the first solution doesn't work. Keep trying different approaches, and you'll find the solution that works for you.
Have you encountered this problem before? How did you solve it? Share your experiences, tips, and tricks in the comments below. Let's help each other out and make the development community a better place!
If you found this blog post helpful, don't forget to share it with your friends and colleagues who might also be facing this issue. Together, we can overcome any coding challenge!
Happy coding! 💻🚀