Remove directory which is not empty
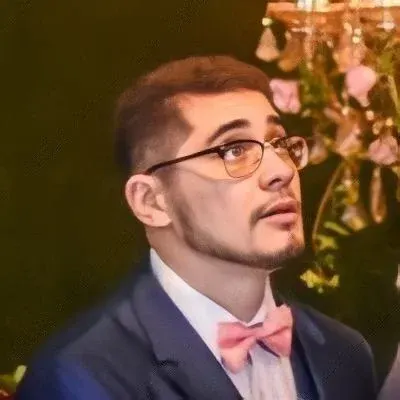

🔄 How to Remove a Non-Empty Directory in Node.js?
Have you ever come across the problem of trying to remove a directory in your Node.js application, only to find out that it's not empty? 📂😓
For those of you who don't know, the fs.rmdir
function in Node.js is specifically designed to remove directories. However, there's one little caveat: it only works on empty directories. 😱
But fear not! I'm here to show you a few easy solutions to tackle this common issue. Let's get started! 🚀
Solution 1: Remove Directory Recursively
One way to solve the problem is to remove all the files and subdirectories inside the target directory before attempting to remove the directory itself. 🗑️
Here's a simple example using the built-in fs
module and its asynchronous method fs.readdir
to read the contents of the directory:
const fs = require('fs');
const path = require('path');
function removeDir(directoryPath) {
if (!fs.existsSync(directoryPath)) {
return;
}
fs.readdirSync(directoryPath).forEach(file => {
const filePath = path.join(directoryPath, file);
const stat = fs.lstatSync(filePath);
if (stat.isDirectory()) {
removeDir(filePath);
} else {
fs.unlinkSync(filePath);
}
});
fs.rmdirSync(directoryPath);
}
// Usage
const directoryPath = '/path/to/directory';
removeDir(directoryPath);
In this code snippet, we use recursion to traverse the directory structure, removing both files and nested directories until the target directory is empty. Finally, we remove the empty directory using fs.rmdirSync
.
Solution 2: Use a Third-Party Library
If writing custom code seems like a hassle, another option is to use a third-party library that simplifies the process. One such library is rimraf, which provides a cross-platform solution to remove directories recursively.
To use rimraf
, you need to install it as a dependency in your project:
npm install rimraf
Then, you can remove a non-empty directory with a single line of code:
const rimraf = require('rimraf');
// Usage
const directoryPath = '/path/to/directory';
rimraf.sync(directoryPath);
🎉 That's it! Your non-empty directory is now gone!
🌟 Bonus Tip: Graceful Error Handling
Sometimes, unexpected errors might occur when removing a directory. To prevent your application from crashing, it's a good practice to handle errors gracefully.
Here's an example of how you can use a try...catch
block to handle errors:
try {
removeDir(directoryPath);
console.log('Directory removed successfully!');
} catch (err) {
console.error('An error occurred while removing the directory:', err);
}
By wrapping the removal code in a try...catch
block, any errors that are thrown during the process will be caught, allowing you to handle them accordingly.
📢 Share Your Experience
So, have you ever encountered this tricky situation when dealing with non-empty directories in Node.js? How did you solve it? Share your experience in the comments below and let's help each other! 🗣️💬
If you found this guide helpful, don't forget to share it with your fellow developers! Let's spread the knowledge and make our programming lives easier. 🤝🔗
Until next time, happy coding! 😄👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
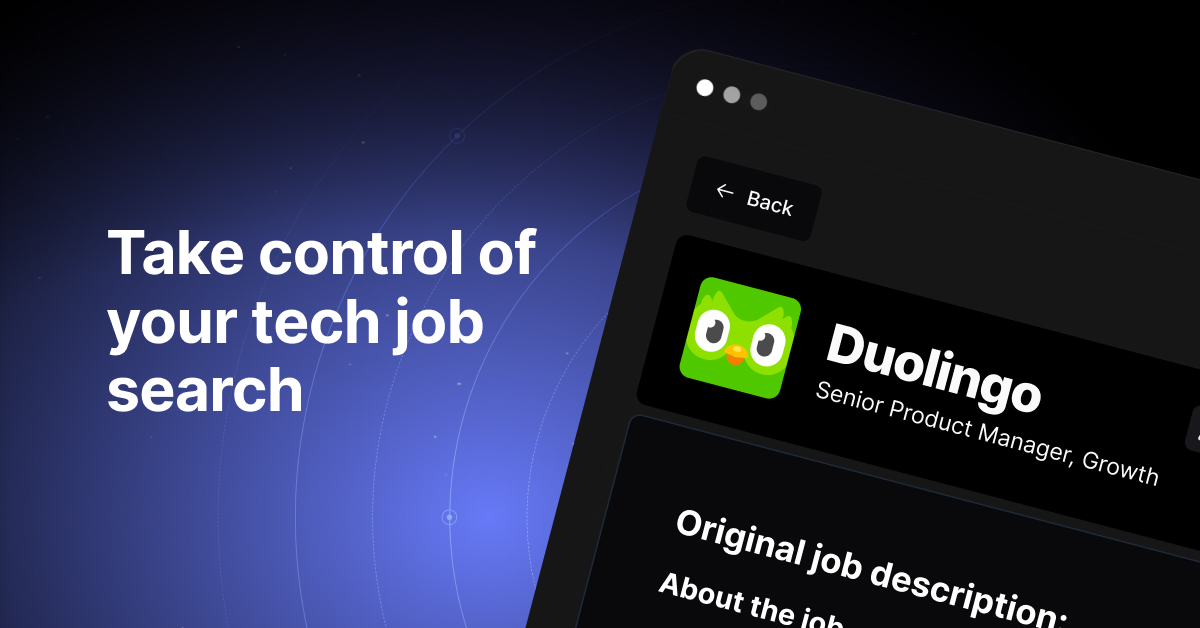