Push items into mongo array via mongoose
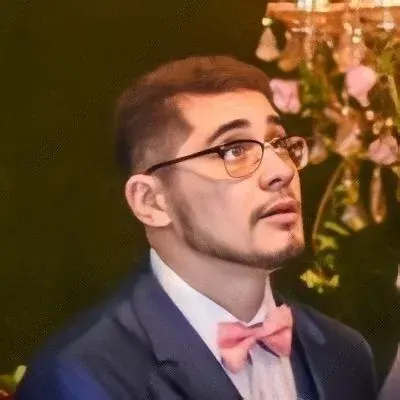
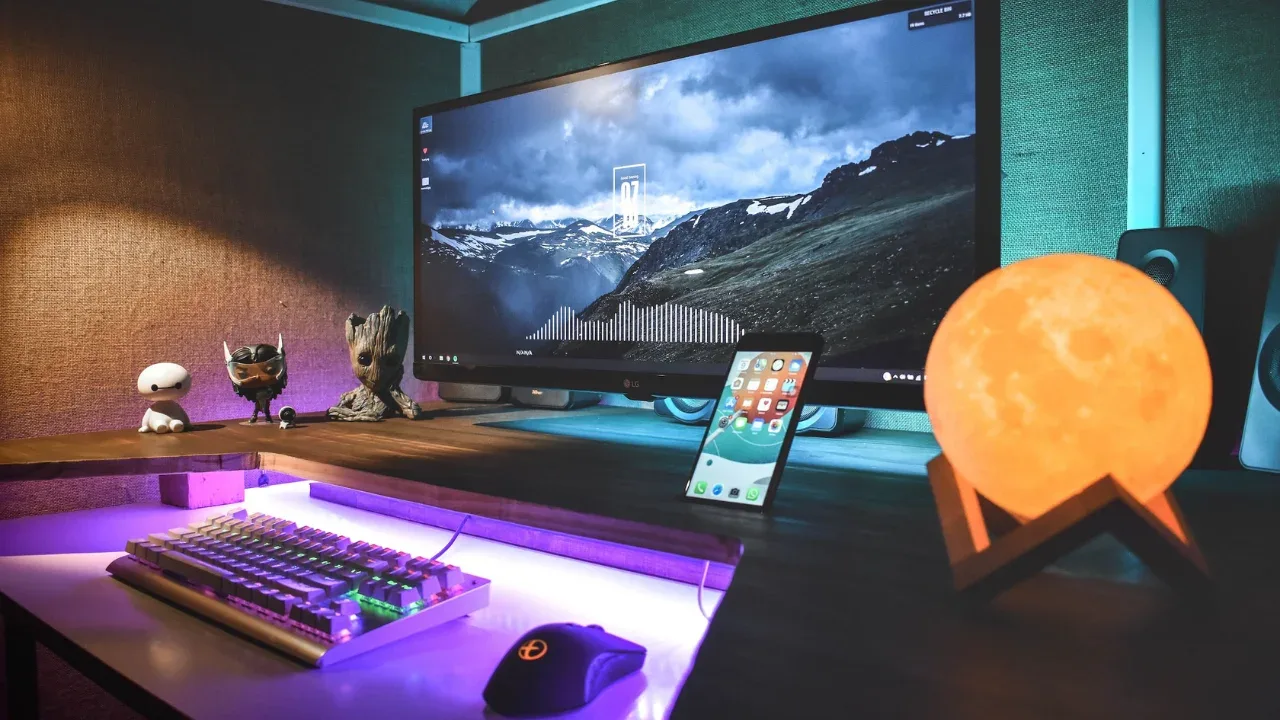
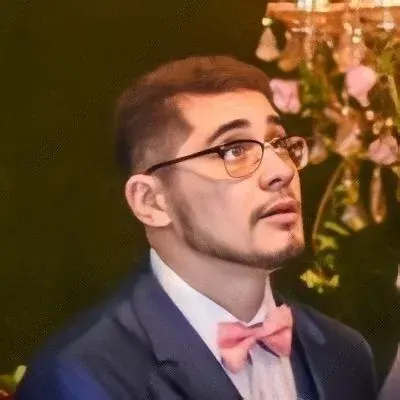
π Blog Post: How to Push Items into Mongo Array via Mongoose
Welcome to another exciting blog post, tech enthusiasts! Today, we're going to dive into the world of MongoDB and Mongoose to solve a common issue: pushing items into an array. Specifically, we'll explore how to push new friend objects into the friends
array of a people
collection using Mongoose.
Understanding the Problem
Picture this: you have a MongoDB collection called people
with the following schema:
people: {
name: String,
friends: [{firstName: String, lastName: String}]
}
In your express application, you successfully create a people
object with an empty friends
array. Now, you want to implement a form to add friends. The form takes in firstName
and lastName
and then performs a POST request with the name
field also included as a reference to the proper people
object.
The challenge lies in creating a new friend object and "pushing" it into the friends
array. While you are familiar with using the MongoDB console and the $push
operator to achieve this, you are now perplexed about how to accomplish the same task using Mongoose.
Finding the Solution
Fear not, dear reader, for we're about to unravel the mystery and provide you with a straightforward solution! To push items into a MongoDB array using Mongoose, we need to follow a few steps:
Retrieve the
people
object using the providedname
field.Create a new friend object using the
firstName
andlastName
values from the form.Push the newly created friend object into the
friends
array of the retrievedpeople
object.Save the updated
people
object back to the database.
To translate this solution into code, we can use the following Mongoose methods:
const People = require('./models/people'); // Importing the Mongoose model
const addFriend = async (name, firstName, lastName) => {
try {
const people = await People.findOne({ name }); // Retrieve the people object
const newFriend = { firstName, lastName }; // Create a new friend object
people.friends.push(newFriend); // Push the new friend into the friends array
await people.save(); // Save the updated people object back to the database
console.log('Friend added successfully!');
} catch (error) {
console.error('Error adding friend:', error);
}
};
Wrapping Up
VoilΓ ! With the code snippet above, you should now be able to push new friend objects into the friends
array of your people
collection using Mongoose. π
Remember, when faced with a challenging coding scenario, staying calm and exploring the steps required can lead you to the right solution. As you embrace this newfound knowledge, don't forget to experiment, break things, and learn from your mistakes. That's how real growth happens in the tech world!
Now it's your turn: go ahead and implement this solution in your application. Donβt hesitate to reach out if you have any questions or cool ideas to share in the comments below. π½
Happy coding! π»π