Properly close mongoose"s connection once you"re done
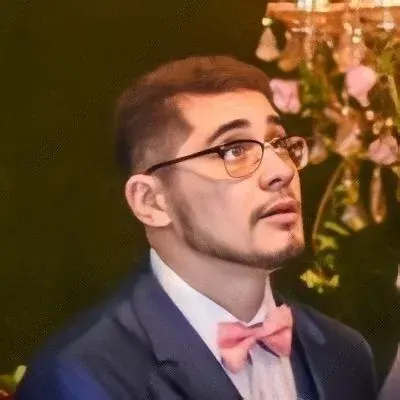
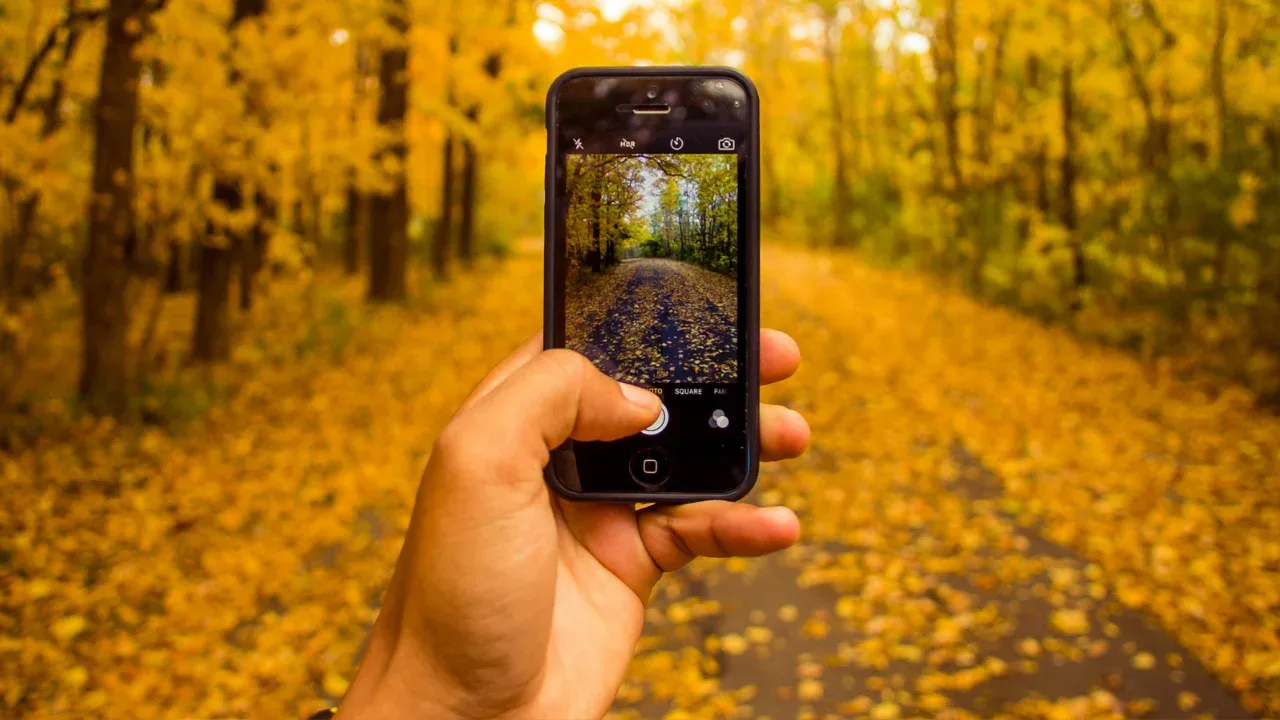
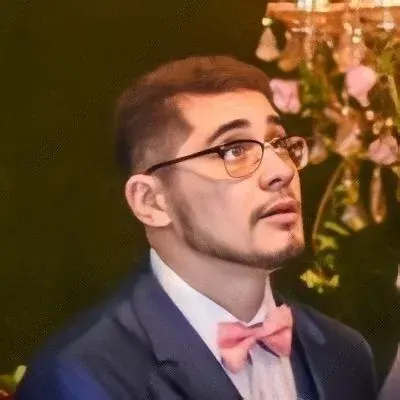
📝 How to Properly Close Mongoose's Connection Once You're Done
Are you using Mongoose in a script that doesn't run continuously? Do you find that your node.js instance never stops after making a call to any Mongoose function that sends requests to MongoDB? You're not alone! This seemingly simple issue can be frustrating to deal with, but fear not! We're here to provide you with easy solutions and help you properly close the Mongoose connection.
🛠️ The Code Issue: Connection Not Closing Properly
Let's take a look at the code provided:
var mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/somedb');
var MyModel = mongoose.model('MyModel', MySchema);
The issue arises when you include the line var MyModel = mongoose.model('MyModel', MySchema);
. After this line, your node.js instance never stops. What's happening here?
💡 The Problem Explained
It turns out that calling mongoose.connect()
does not guarantee that the connection is closed once your script ends. The connection might still be open if there are ongoing queries or operations.
🔧 Easy Solutions to Properly Close the Connection
Now, let's dive into the solutions that will help you properly close the Mongoose connection and prevent your node.js instance from running indefinitely:
Solution 1: Using callbacks or promises
// Perform required Mongoose operations
// Use a callback or promise to ensure all queries are completed
MyModel.find({}, function(err, docs) {
if (err) {
console.error(err);
} else {
// All queries completed - close the connection
mongoose.connection.close(function() {
console.log('Mongoose connection closed');
});
}
});
Solution 2: Using async/await
// Perform required Mongoose operations
// Use async/await to wait for all queries to complete
await MyModel.find({});
// Close the connection
mongoose.connection.close(function() {
console.log('Mongoose connection closed');
});
💡 The Key Takeaway: Ensure All Queries Are Processed
The key takeaway here is to make sure that every query or operation has been processed before calling mongoose.connection.close()
. This ensures that the connection is truly closed and prevents your node.js instance from running indefinitely.
🚀 Call-To-Action: Share Your Experience and Connect with Us!
We hope this guide helped you solve the issue of closing the Mongoose connection properly. If you have faced similar problems or have any additional tips, we'd love to hear from you in the comments section below!
✨ Remember to share this guide with anyone else who might be dealing with this issue. Sharing is caring! 💙
#mongoose #nodejs #backenddevelopment #mongodb