Node.js throws "btoa is not defined" error
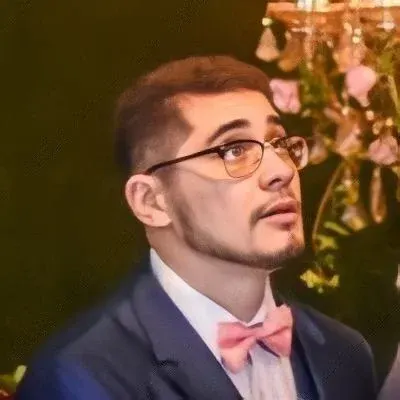
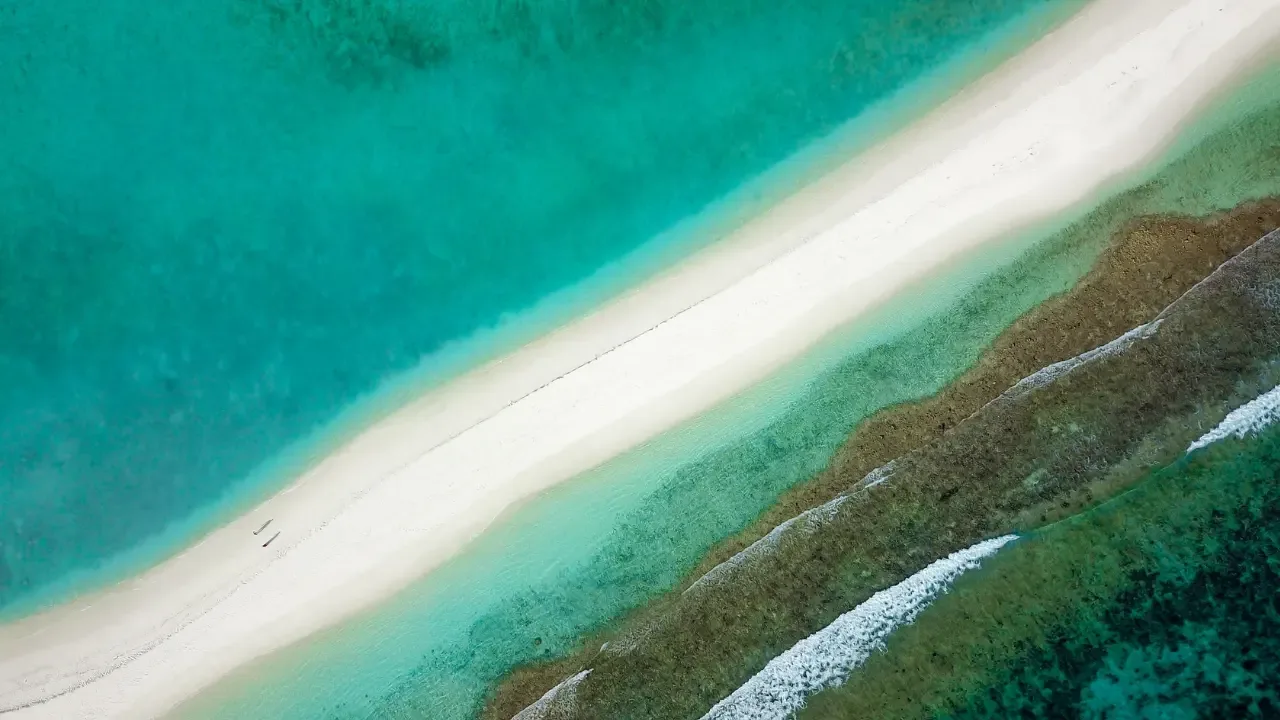
How to Fix the "btoa is not defined" Error in Node.js 🚀
So, you're working on your Node.js application and you want to use the btoa()
function, but it throws an "btoa is not defined" error. 😩 Don't worry, we've got you covered! In this article, we'll explore the common issues and provide easy solutions to get rid of this pesky error. 💪
Understanding the Problem
The error occurs because the btoa()
function is not available in Node.js by default. It is a client-side JavaScript function that encodes a string to Base64. However, fear not! We can easily fix this issue by using a package called btoa-atob
. 📦
Solution
Here are the steps to solve the "btoa is not defined" error:
Install the
btoa-atob
package using npm:npm install btoa-atob
Once the installation is complete, verify that the
btoa-atob
package is present in thenode_modules
directory.Ensure that you have added
btoa-atob
as a dependency in yourpackage.json
file. Open thepackage.json
file and check for the following entry:"dependencies": { "btoa-atob": "^x.x.x" }
Note: Replace
^x.x.x
with the actual version number of thebtoa-atob
package.Import the
btoa
function at the top of your JavaScript file:const btoa = require('btoa-atob').btoa;
Now, you can use the
btoa()
function in your Node.js application!Test the
btoa()
function by encoding a string:console.log(btoa('Hello World!'));
You should now see the expected output in the console:
"SGVsbG8gV29ybGQh"
.
Possible Issues and Troubleshooting
1. Incorrect Installation
Double-check if you properly installed the btoa-atob
package. Make sure to run npm install btoa-atob
in the correct directory.
2. Missing or Incorrect Dependency Entry
Verify that you have added the correct dependency entry for btoa-atob
in your package.json
file. Check for typos and ensure that the version number is accurate.
3. Importing the Function Incorrectly
Ensure that you are correctly importing the btoa
function from the btoa-atob
package. Follow the import statement provided in the solution section.
Conclusion and Action Time! 🎉
You've successfully resolved the "btoa is not defined" error in your Node.js application. Pat yourself on the back, you rock! 🎸
Now, go ahead and implement the btoa()
function wherever you need it in your code. Utilize the power of Base64 encoding in your Node.js application, and take your project to the next level. 🚀
Remember, if you have any questions or encounter any roadblocks, feel free to leave a comment below. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
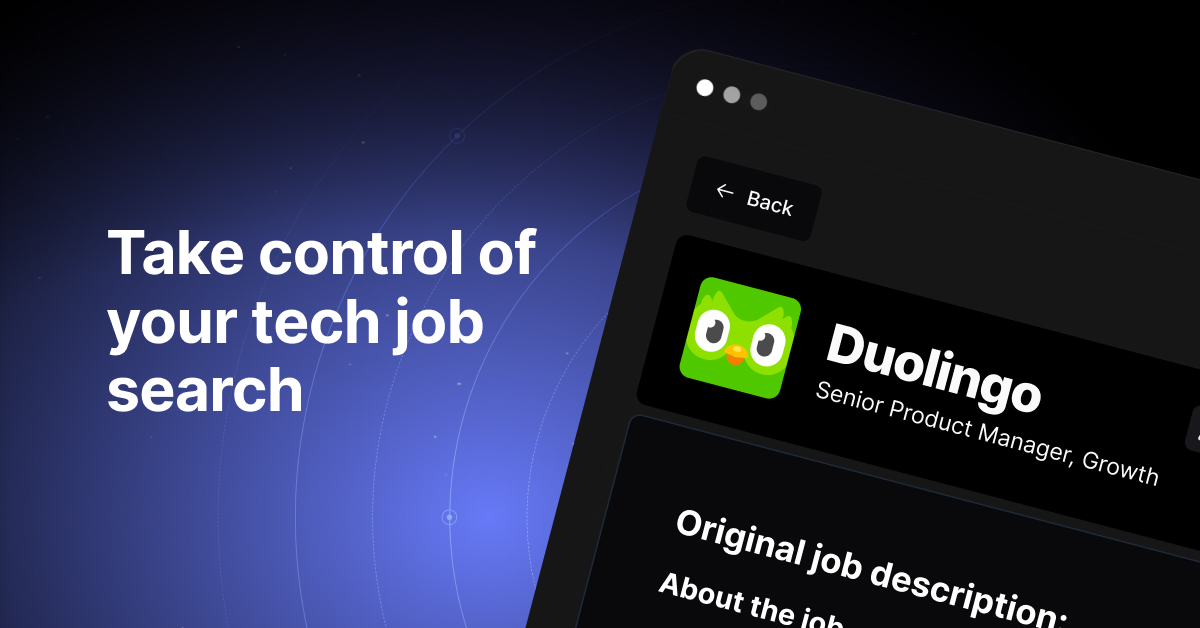