Node.js check if path is file or directory
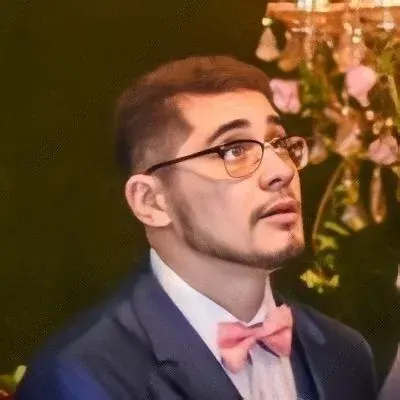
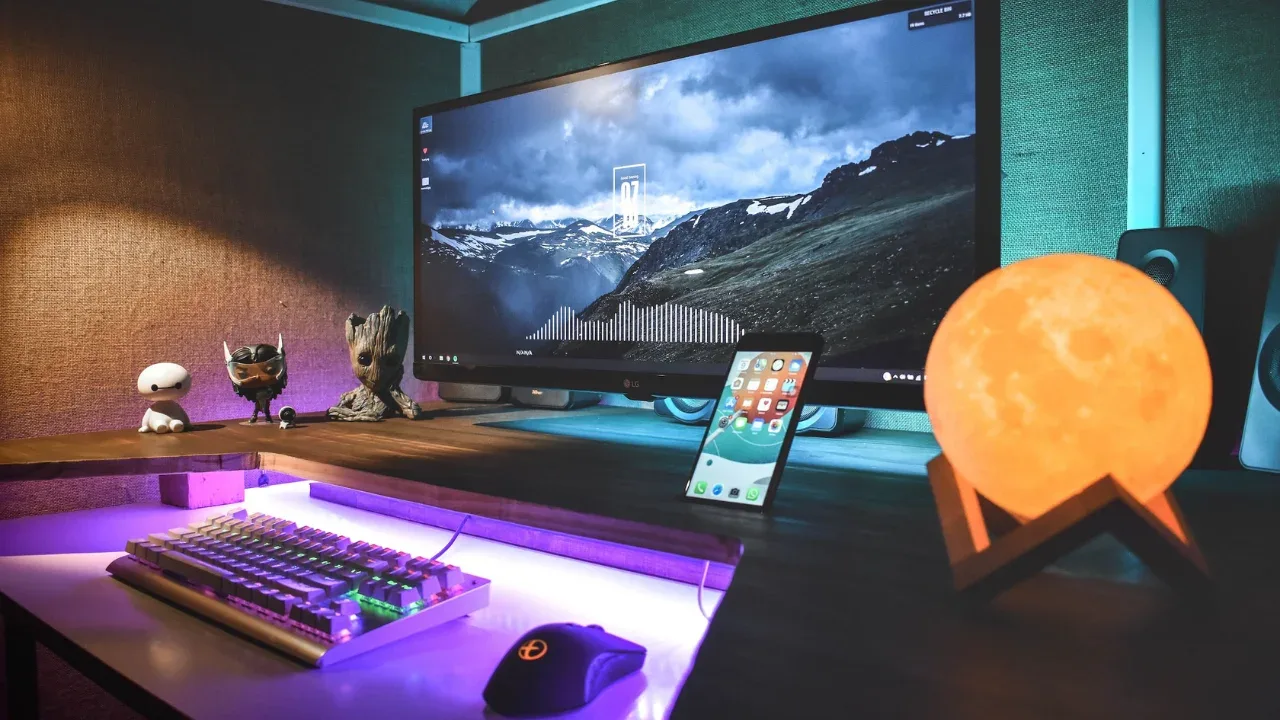
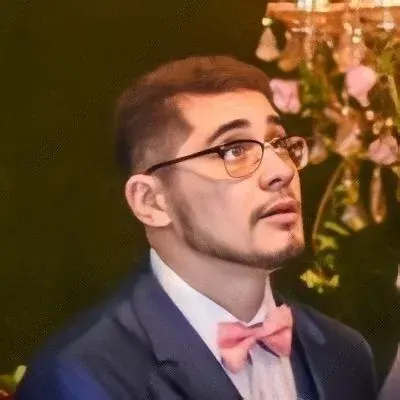
How to Check if a Path is a File or Directory in Node.js
š Are you struggling to determine whether a given path is a file or a directory in your Node.js application? You're not alone! Many developers find it challenging to find clear explanations and solutions to this common problem. But worry not, because in this blog post, we will guide you through the process step-by-step and provide easy-to-implement solutions. Let's dive in! šāāļø
The Challenge
š¤ It seems like you're having trouble finding search results that explain how to check if a given path is a file or a directory in Node.js. This can be a daunting task, especially if you're new to file system operations in Node.js. But don't worry, we've got your back! š¤
Solution 1: fs.stat()
āļø One simple solution is to use the built-in fs
module in Node.js. Specifically, the fs.stat()
function can be used to retrieve information about a given path, and help us determine whether it's a file or a directory. Here's how you can use it in your code:
const fs = require('fs');
fs.stat(path, (err, stats) => {
if (err) {
console.error(err);
return;
}
if (stats.isFile()) {
console.log('The path is a file.');
} else if (stats.isDirectory()) {
console.log('The path is a directory.');
} else {
console.log('The path does not exist.');
}
});
š Replace path
with the actual path (absolute or relative) you want to check. The fs.stat()
function retrieves information about the file or directory at the given path, and the resulting stats
object provides useful methods like .isFile()
and .isDirectory()
to determine the type of the path.
Solution 2: fs.lstat()
š Another approach is to use fs.lstat()
instead of fs.stat()
. The difference is that fs.lstat()
does not follow symbolic links, whereas fs.stat()
does. Depending on your specific use case, you might need to choose between these two methods. Here's an example of using fs.lstat()
:
const fs = require('fs');
fs.lstat(path, (err, stats) => {
if (err) {
console.error(err);
return;
}
if (stats.isFile()) {
console.log('The path is a file.');
} else if (stats.isDirectory()) {
console.log('The path is a directory.');
} else {
console.log('The path does not exist.');
}
});
ā ļø Remember, when using fs.lstat()
, symbolic links will not be followed.
The Call-to-Action
š We hope these solutions have helped you in checking whether a path is a file or a directory in Node.js. Now it's your turn to take action! Implement these solutions in your code, analyze the results, and feel the joy of solving the problem. Don't forget to share your success story with fellow developers in the comments below! š¬
š ļø If you have any questions, need further assistance, or want more guides on Node.js development, follow our blog and join our community on social media. Let's learn and grow together! š±
Happy coding! āØš