Mongoose, update values in array of objects
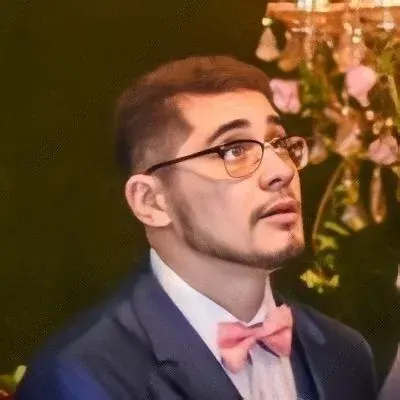
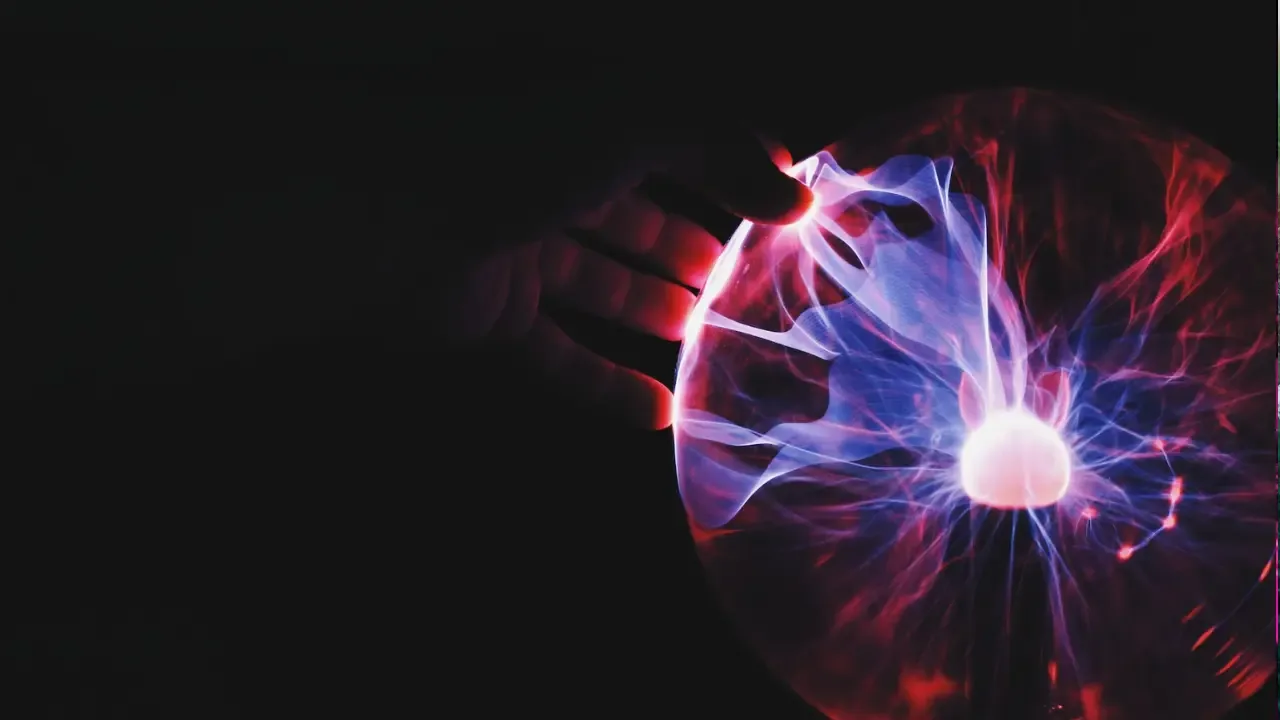
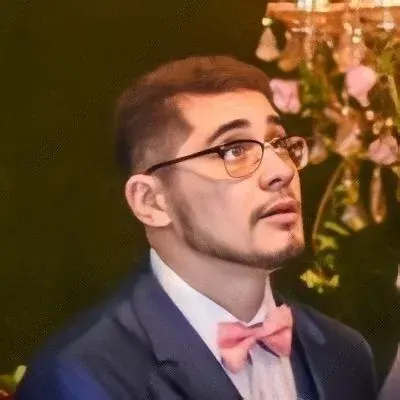
š Mongoose, update values in array of objects š
Are you facing the challenging task of updating values in an array of objects using Mongoose? Don't worry, we've got you covered! š ļø
šØ The Problem šØ
Let's start by understanding the problem at hand. You have a document structure in Mongoose with an array of objects. For example, consider the following structure:
{
_id: 1,
name: 'John Smith',
items: [{
id: 1,
name: 'item 1',
value: 'one'
},{
id: 2,
name: 'item 2',
value: 'two'
}]
}
Now, suppose you want to update the name
and value
fields of an item where id = 2
. š
You might attempt to update it using the following Mongoose code:
var update = {name: 'updated item 2', value: 'two updated'};
Person.update({'items.id': 2}, {'$set': {'items.$': update}}, function(err) {...
š The Issue š
Unfortunately, the above approach replaces the entire object in the array, causing you to lose the id
field. š±
š” A Better Solution š”
But fear not, there is a better way in Mongoose to update only certain values in an array while leaving other values intact. Let's dive into the solution! šŖ
First, you can query for the Person
document using Person.find()
. Once you have the document, you can iterate through the items
array to find the item with id = 2
. Once you find the item, you can update the desired fields and then save the document using person.save()
. Here's an example:
Person.find({...}, function(err, person) {
person.items.forEach(function(item) {
if (item.id === 2) {
item.name = 'updated item 2';
item.value = 'two updated';
}
});
person.save(function(err) {
// Handle errors and perform any additional actions
});
});
This solution allows you to selectively update only the desired fields and retain the original structure of the document. š
š Let's Recap š
To update values in an array of objects using Mongoose, you can follow these steps:
Query for the document using
Person.find()
.Iterate through the array and find the desired object based on the specified criteria, such as
id
.Update the desired fields in the object.
Save the document using
person.save()
.
š¬ Engage with Us š¬
We hope this guide helped you solve the problem of updating values in an array of objects using Mongoose. If you have any questions or suggestions, feel free to leave a comment below. Let's keep the conversation going! š