Mongoose indexing in production code
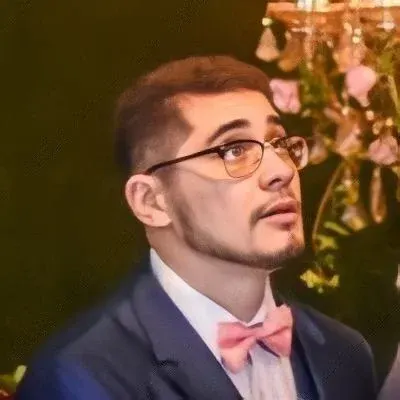
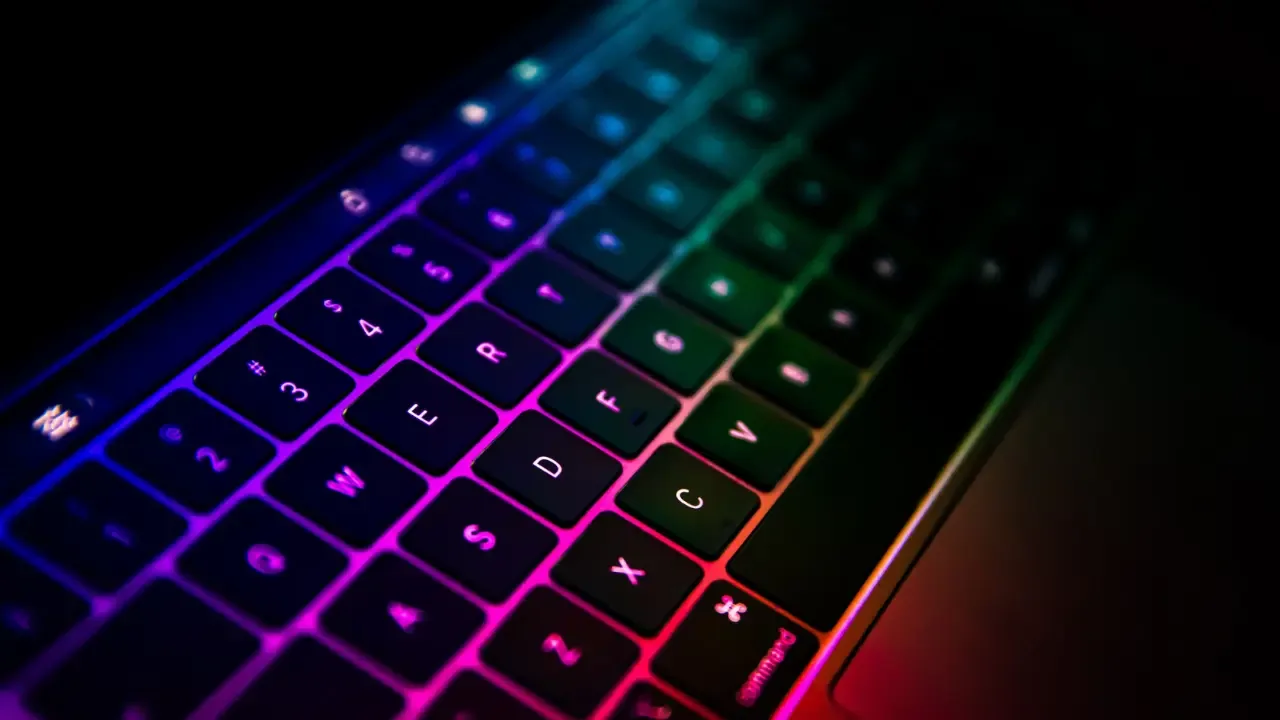
📝📈 MongoDB Indexing in Production Code: How to Optimize Mongoose for Performance
Are you using Mongoose with MongoDB and Node.js in your production code? If so, you may have come across the recommendation in the Mongoose documentation to disable auto-indexing for your schema in production. But what does this mean, and how should you handle indexing in your production code? In this blog post, we'll address these common questions and provide easy solutions to optimize Mongoose for performance.
🚀 The Problem: Auto-Indexing Impact on Performance
According to the Mongoose documentation, when your application starts up, Mongoose automatically calls ensureIndex
for each defined index in your schema. This is convenient for development purposes, as it ensures that your indexes are always up to date. However, in production, this behavior can cause a significant performance impact. So, it is recommended to disable the auto-indexing behavior by setting the autoIndex
option of your schema to false
.
💡 The Solution: External Script for Index Generation
To optimize your application's performance, you can consider using an external script to generate indexes. Instead of relying on Mongoose's auto-indexing, this approach allows you to control when and how indexes are created. By running the script during deployment or at a scheduled interval, you can ensure that index creation doesn't impact your production code's performance.
Here's an example of how you can use an external script to generate indexes:
const mongoose = require('mongoose');
const MyModel = require('./models/myModel');
mongoose.connect('mongodb://localhost/myDatabase', { useNewUrlParser: true });
MyModel.createIndexes()
.then(() => {
console.log('Indexes created successfully');
process.exit(0);
})
.catch((error) => {
console.error('Failed to create indexes', error);
process.exit(1);
});
In this example, we're using the createIndexes
method from Mongoose to generate indexes for our MyModel
schema. You can customize this script based on your specific schema and deployment needs. Running this script ensures that indexes are created outside of your production code's startup phase, avoiding any potential performance issues.
💡 Another Consideration: Indexing for Writer Applications
If your application is the sole reader and writer to a collection, you might be wondering if ensureIndex
is unnecessary. In this case, the index will be continued every time a write operation occurs on the database, making the use of ensureIndex
redundant. Thus, the autoIndex
option in Mongoose should be a no-op under a normal server restart.
🔍 Deeper Dive: MongoDB Documentation on Indexing
While we've covered the practical solution to handling indexing in production code using Mongoose, you may still be curious about the "why" and "when" behind explicit indexing directives. MongoDB provides excellent documentation on the technical aspects of indexing, but it lacks guidance on when and why you should use explicit indexing.
In general, indexes should be kept up to date by writer applications automatically, especially for collections with existing indexes. The ensureIndex
method in Mongoose is more commonly used when applying a new index, making it a one-time operation. This means that under normal circumstances, you don't need to worry about manually invoking ensureIndex
for every write operation.
📣 Your Turn: Optimize Mongoose for Performance!
Now that you have a clear understanding of how to handle indexing in your production code using Mongoose, it's time to take action. Consider implementing an external script to generate your indexes and disable Mongoose's auto-indexing in your production environment. Test and monitor the impact on your application's performance, and don't forget to share your success stories or any challenges you face along the way in the comments below. Let's optimize Mongoose together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
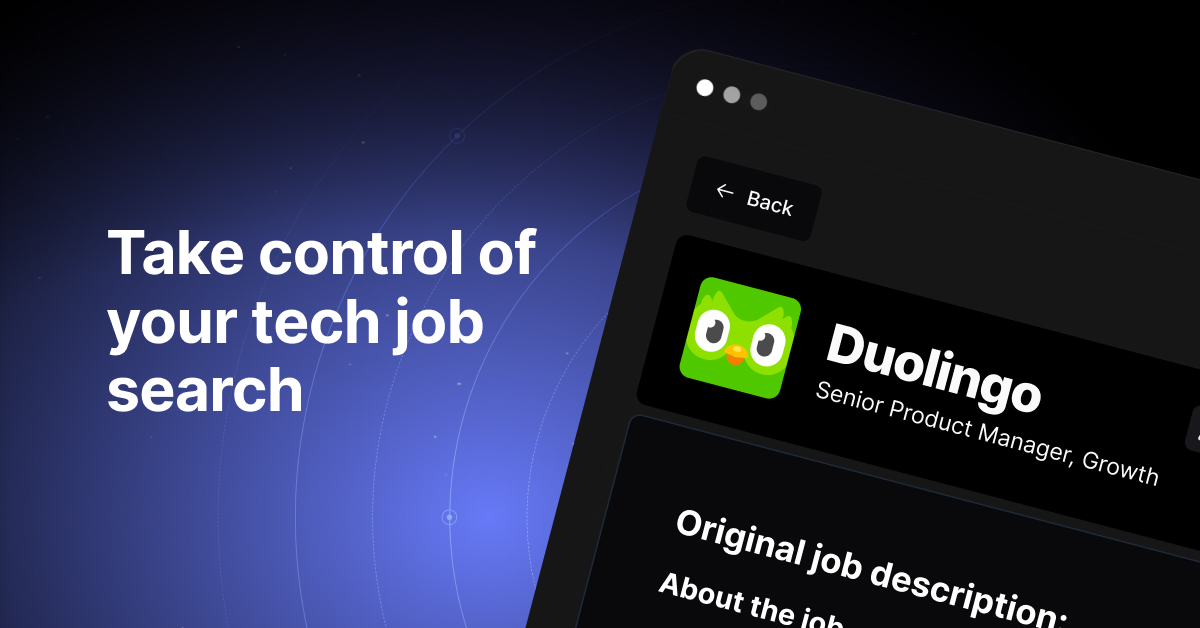