Mongoose: findOneAndUpdate doesn"t return updated document
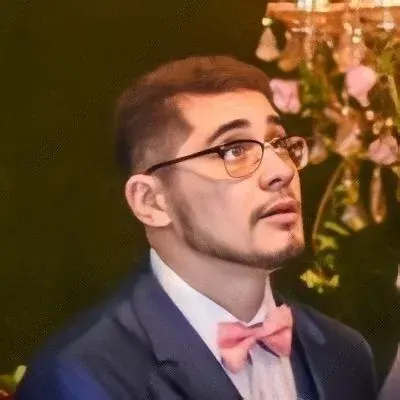
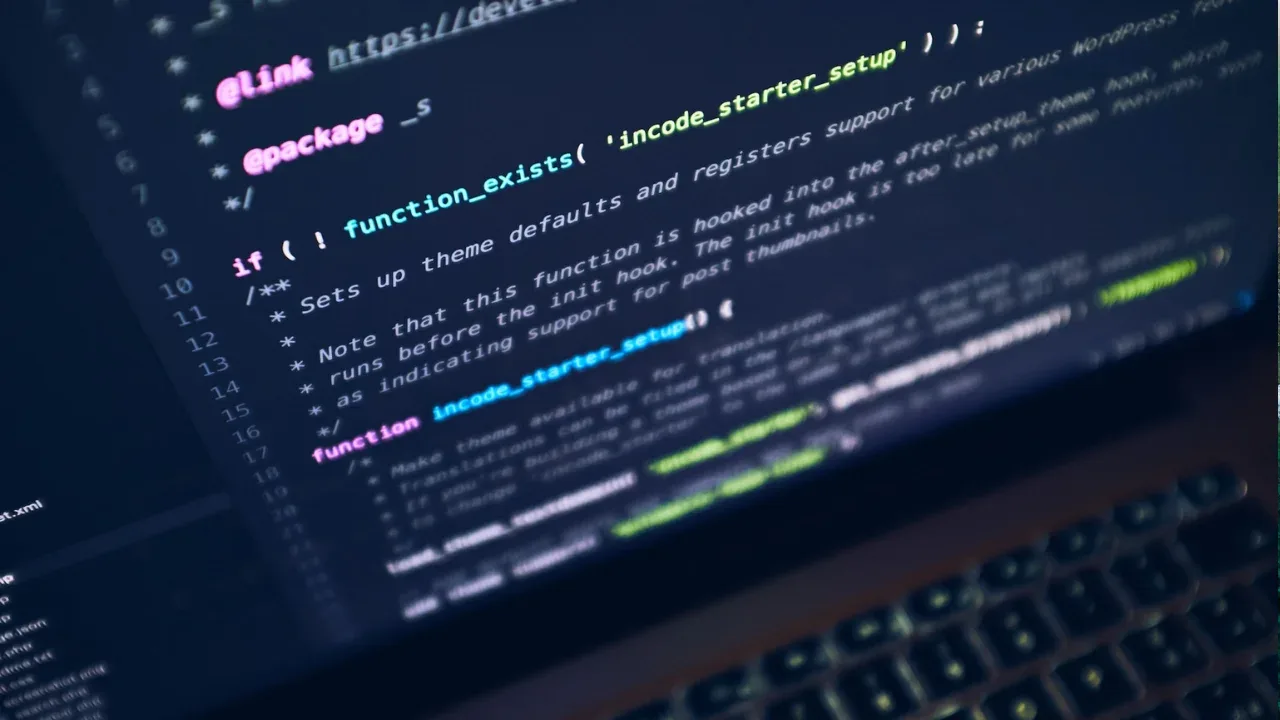
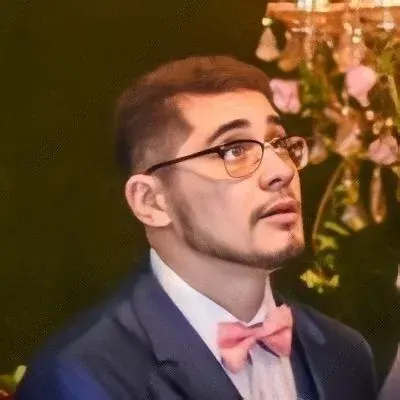
🐍 Mongoose: findOneAndUpdate Doesn't Return Updated Document
Are you experiencing an issue where the findOneAndUpdate
method in Mongoose doesn't return the updated document? You're not alone! This can be quite confusing, but don't worry, we've got you covered. In this blog post, we'll dive into common issues and provide easy solutions to this problem. 😊
The Code
Let's start by taking a look at the code you provided:
var mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/test');
var Cat = mongoose.model('Cat', {
name: String,
age: {type: Number, default: 20},
create: {type: Date, default: Date.now}
});
Cat.findOneAndUpdate({age: 17}, {$set:{name: "Naomi"}}, function(err, doc){
if(err){
console.log("Something wrong when updating data!");
}
console.log(doc);
});
The Issue
You mentioned that you have some records in your MongoDB database and you want to update the name of the cat with an age of 17. However, when you run the code and log the result, you still get the original data instead of the modified name. It's important to note that when you query the database separately using db.cats.find()
in the MongoDB command line, you do see the modified name. So why does the initial console log show the original data?
The Explanation
The reason for this behavior lies in the asynchronous nature of JavaScript and Mongoose. When you call findOneAndUpdate
, it doesn't wait for the update operation to complete before moving on to the next line of code. This means that the console.log(doc)
statement is executed before the document is actually updated.
The Solution
To ensure that you get the updated document, you need to modify your code slightly. Instead of relying on the callback of findOneAndUpdate
, you can chain the exec()
method to the query and handle the result within a then()
block. Here's an updated version of your code:
Cat.findOneAndUpdate({ age: 17 }, { $set: { name: "Naomi" } })
.exec()
.then(doc => {
console.log(doc);
})
.catch(err => {
console.log("Something went wrong when updating data!");
console.error(err);
});
By using .exec().then()
, we ensure that the console.log(doc)
statement will only be executed after the update operation is complete, and you will see the updated document in the console.
🚀 Take Action!
Now that you have an easy solution to the issue, it's time to try it out and see the updated document. Update your code, run it, and sit back as you witness the power of the updated findOneAndUpdate
method in Mongoose. Don't forget to share your success with us and engage in the comments section below!
Happy coding! 🎉