Mongoose findByIdAndUpdate not returning correct model
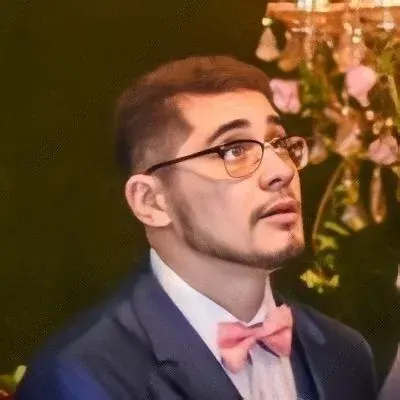
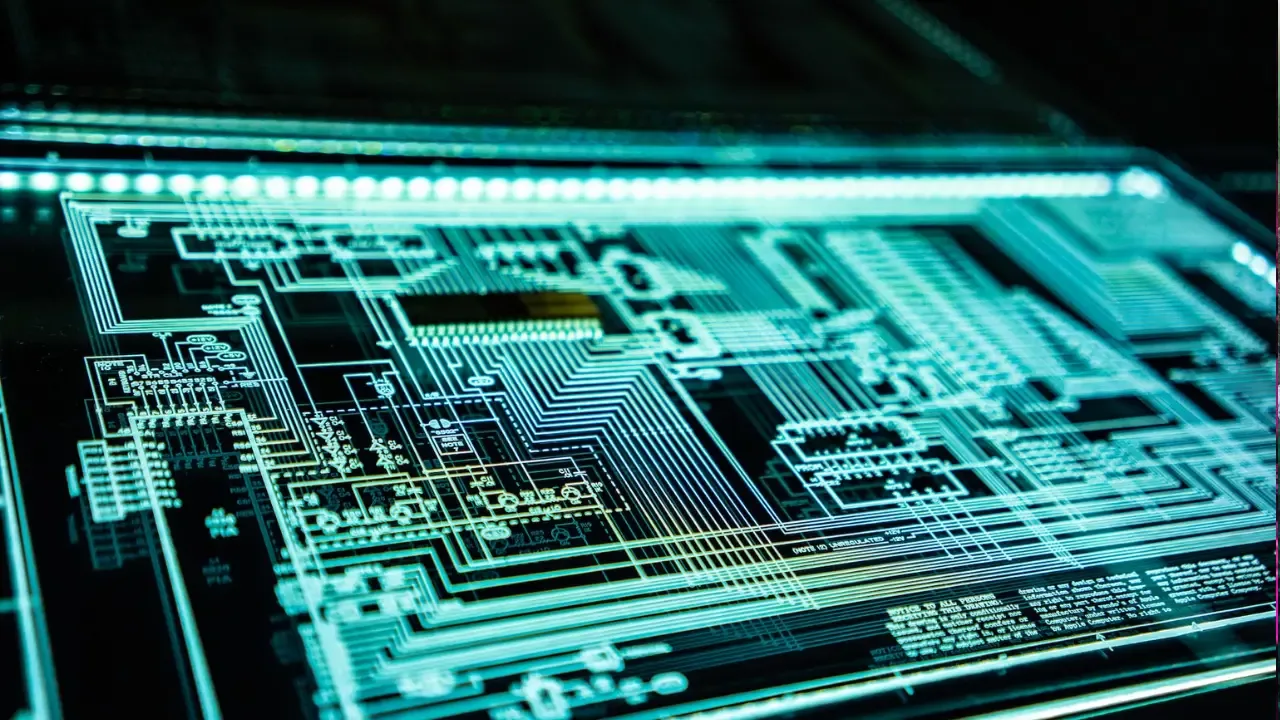
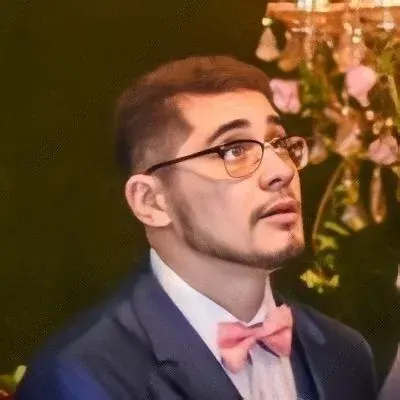
🐍 Mongoose findByIdAndUpdate not returning correct model? Let's fix it! 🛠️
So you're working with Mongoose and you're facing a weird issue where the findByIdAndUpdate
method is not returning the correct model in the callback. Don't worry, we're here to help you solve this puzzle! 🧩
📜 Understanding the problem
Let's take a closer look at the code snippet provided:
var id = args._id;
var updateObj = { updatedDate: Date.now() };
_.extend(updateObj, args);
Model.findByIdAndUpdate(id, updateObj, function(err, model) {
if (err) {
logger.error(modelString + ':edit' + modelString + ' - ' + err.message);
self.emit('item:failure', 'Failed to edit ' + modelString);
return;
}
self.emit('item:success', model);
});
The updateObj
contains the updated fields you want to apply to the document. However, the model returned in the callback is identical to the original model, not the updated one. Strange, right? 🤔
🐞 Common Pitfall
The issue here lies in how you are extending the updateObj
using _.extend
(assuming you're using Underscore or Lodash). This method is modifying the original updateObj
object and not creating a new updated object. Therefore, Mongoose will not recognize the changes and return the original model instead.
💡 Simple Solution
To fix this, we recommend creating a new updated object by combining the properties from both updateObj
and args
. Here's an updated code snippet:
var id = args._id;
var updateObj = { updatedDate: Date.now(), ...args };
Model.findByIdAndUpdate(id, updateObj, function(err, model) {
if (err) {
logger.error(modelString + ':edit' + modelString + ' - ' + err.message);
self.emit('item:failure', 'Failed to edit ' + modelString);
return;
}
self.emit('item:success', model);
});
By using the spread operator (...args
), you can merge the properties from args
into the updateObj
, creating a new updated object. This way, Mongoose will recognize the changes and return the correct model in the callback.
🚀 Call-to-Action: Share Your Thoughts!
We hope this guide helped you solve the issue with findByIdAndUpdate
not returning the correct model. If you have any other questions or similar experiences, feel free to share them in the comments below. Let's learn and troubleshoot together! 😊