module.exports vs. export default in Node.js and ES6
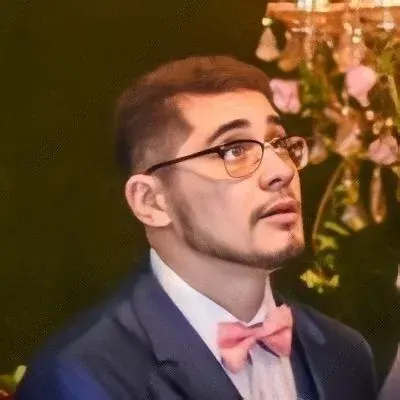
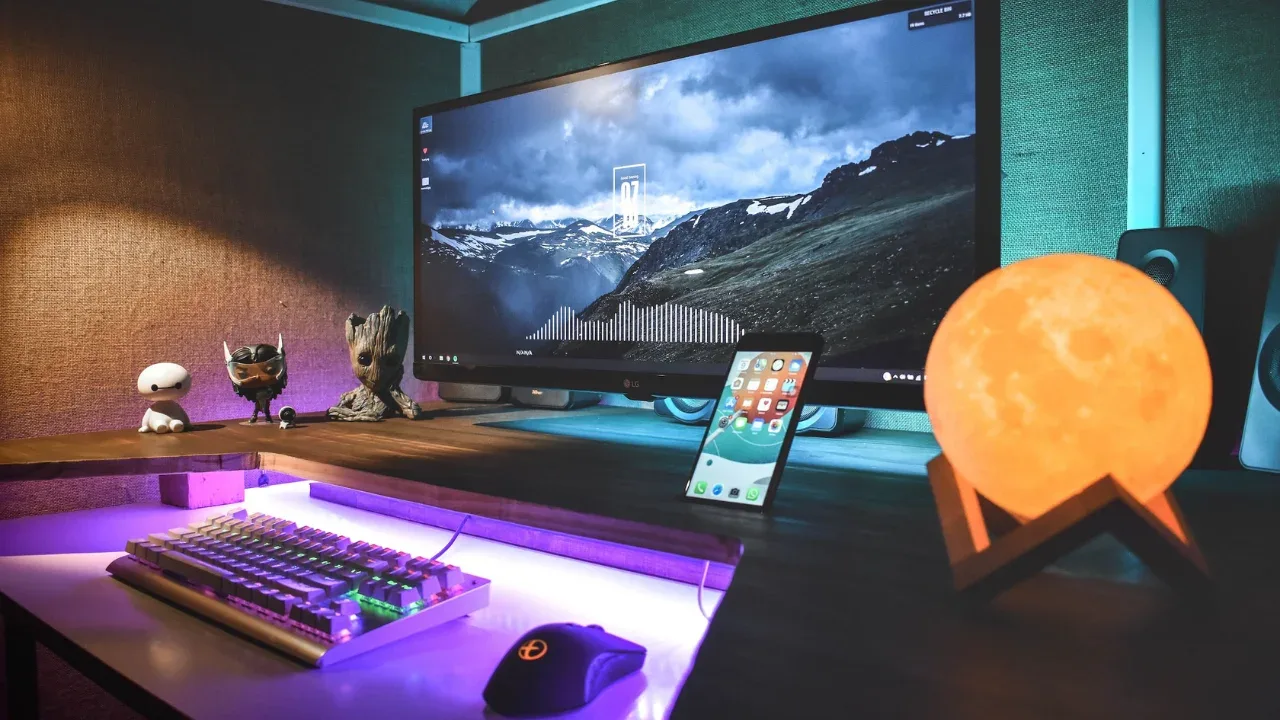
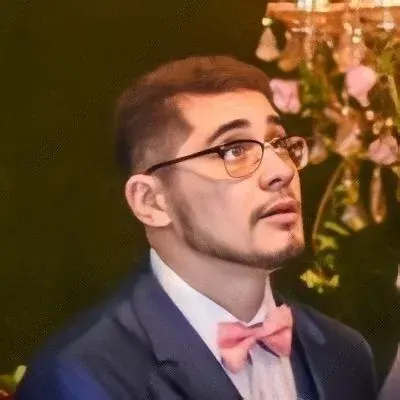
📦 module.exports vs. 📤 export default in 🗂️ Node.js and ES6
So you're trying to understand the difference between Node's module.exports
and ES6's export default
, and you're wondering why you're getting the __ is not a constructor
error when you try to use export default
in Node.js 6.2.2. Don't worry, I've got you covered! Let's dive into it and find out what's going on.
🎯 The Difference
The main difference between module.exports
and export default
lies in how they are used and how they are imported in other files.
📥 Importing with module.exports
When you use module.exports
, you are essentially exporting an entire object or a single value from a file. In the example below, we have a class called SlimShady
that is being exported using module.exports
:
'use strict';
class SlimShady {
constructor(options) {
this._options = options;
}
sayName() {
return 'My name is Slim Shady.';
}
}
// This works
module.exports = SlimShady;
To import this class in another file, you would use the require
function:
const SlimShady = require('./path-to-file');
📤 Importing with export default
On the other hand, when you use export default
, you are only exporting a single value or default export from a file. In the example below, we have the same SlimShady
class being exported using export default
:
'use strict';
class SlimShady {
constructor(options) {
this._options = options;
}
sayName() {
return 'My name is Slim Shady.';
}
}
// This will cause the "SlimShady is not a constructor" error
// if in another file I try `let marshall = new SlimShady()`
export default SlimShady;
To import it in another file, you can use either the import
statement or the require
function with some extra steps:
// Using import statement (ES6)
import SlimShady from './path-to-file';
// Using require function (Node.js with Babel)
const SlimShady = require('./path-to-file').default;
❌ The Error
Now, let's address the specific error you mentioned: "SlimShady is not a constructor"
. This error occurs when you try to create a new instance of SlimShady
using the new
keyword, but SlimShady
is not recognized as a constructor.
This error is likely happening because when you use export default
, the default export is not automatically assigned to the variable name you choose. In other words, when you import the default export, you need to explicitly access it using the .default
property.
In the example above, when importing with require
, you would need to access the default export using .default
:
const SlimShady = require('./path-to-file').default;
Alternatively, if you are using the import
statement, you can directly import the default export without the need for .default
:
import SlimShady from './path-to-file';
💡 Solution
To avoid the __ is not a constructor
error, make sure you are importing the default export correctly, depending on whether you are using the import
statement or the require
function.
📣 Call-to-Action
Understanding the difference between module.exports
and export default
is crucial when working with Node.js and ES6. Remember to be careful when importing default exports and ensure you are using the correct syntax.
I hope this guide has cleared up any confusion you had. If you found it helpful, feel free to share it with others who might be facing the same issue. And if you have any more questions or need further assistance, don't hesitate to leave a comment below! 🤓🚀