In Mongoose, how do I sort by date? (node.js)
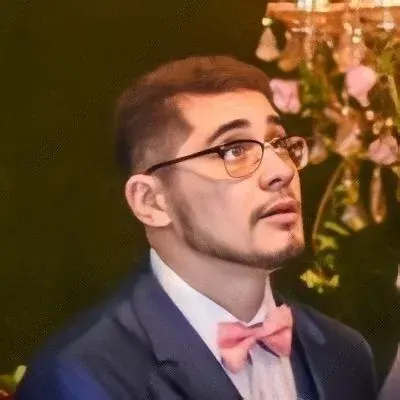
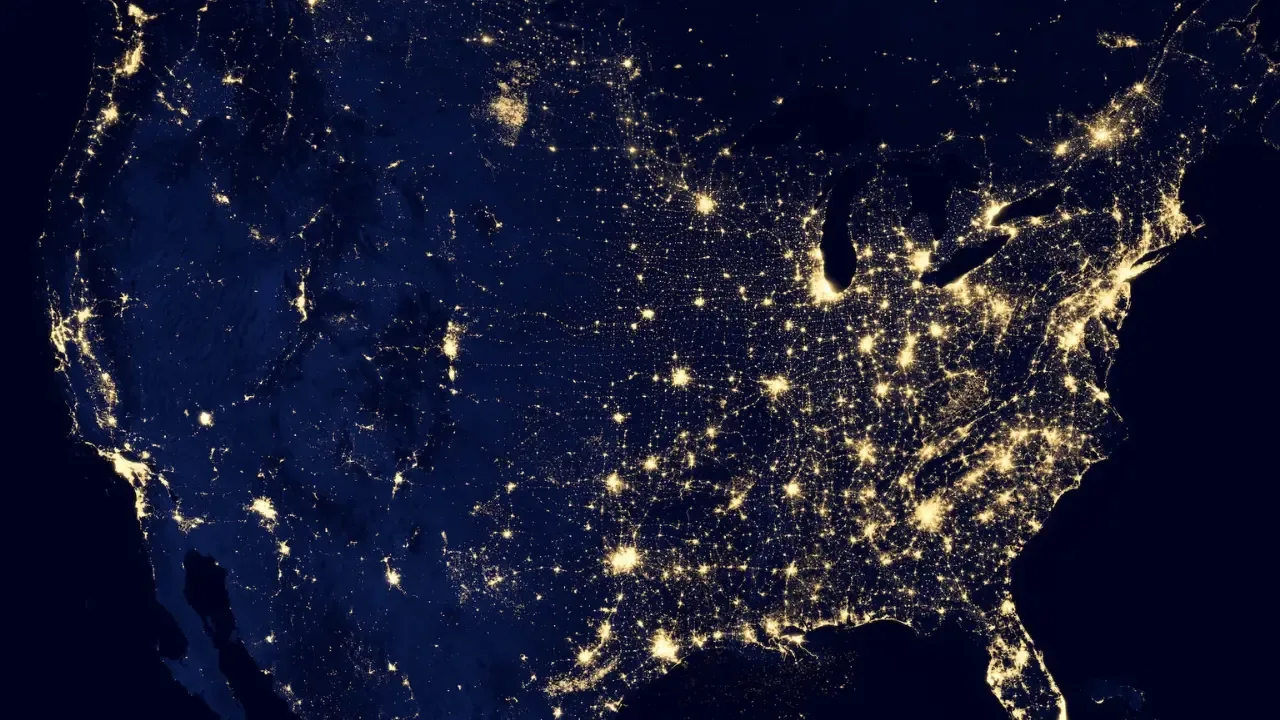
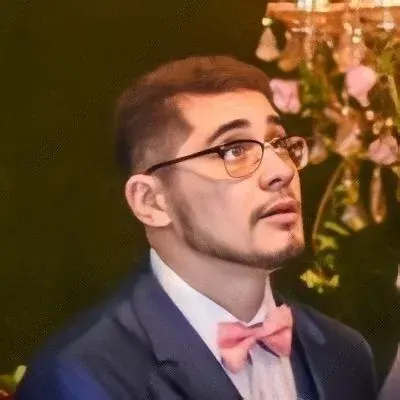
📝✨📅 How to Sort by Date in Mongoose (node.js) 🚀
Are you scratching your head, wondering how to sort documents by date in Mongoose? 🤔 Look no further! In this guide, we will address the common issue you're facing and provide you with easy solutions to get your data sorted in no time. Let's dive right in! 💪
The Problem: Sorting Woes 😫
So, you tried running the following query in Mongoose:
Room.find({}, (err, docs) => {
// Your code here
}).sort({ date: -1 });
But sadly, it seems like something went wrong! 😩 The sorting didn't work as expected, leaving you puzzled and frustrated.
The Solution: Sorting Like a Pro! 💡
Fear not, fellow developer! We have a couple of solutions up our sleeves to help you conquer this sorting obstacle with ease. 🙌
Solution 1: Use an Alternative Date Format 📅
Mongoose relies on the JavaScript Date
object by default, which might cause unexpected behavior when sorting. One way to overcome this is to store your date as a Unix timestamp (e.g., milliseconds since January 1, 1970). Let's see how this can be done:
const roomSchema = new mongoose.Schema({
// Your other schema fields here
date: {
type: Number,
default: Date.now,
index: true,
},
});
By using the Unix timestamp format, you can now sort your documents effortlessly using Mongoose's .sort()
method.
Solution 2: Implement a Custom Comparator Function 🔄
If you prefer to keep your date field as a JavaScript Date
object, you can create a custom sorting function using the .sort()
method. Here's an example to get you started:
Room.find({}, (err, docs) => {
docs.sort((a, b) => b.date - a.date);
// Your code with sorted documents here
});
By providing a custom comparator function inside the .sort()
method, you can achieve the desired sorting order based on the date
field. 🎉
Your Turn: Time to Sort! ⏱️
Now that you are equipped with these handy solutions, it's your turn to give it a try! 😎 Choose the solution that best fits your needs, apply it to your code, and see those documents sorted flawlessly.
Do you have any other Mongoose sorting tricks up your sleeve? Share them in the comments below! 💬 Let's make sorting by date in Mongoose a breezy task for everyone!
Until next time, happy coding! 💻🌈
PS: Don't forget to smash that share button and spread the sorting wisdom! 🚀