Ignore invalid self-signed ssl certificate in node.js with https.request?
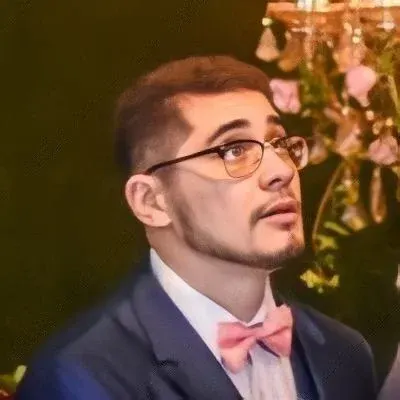
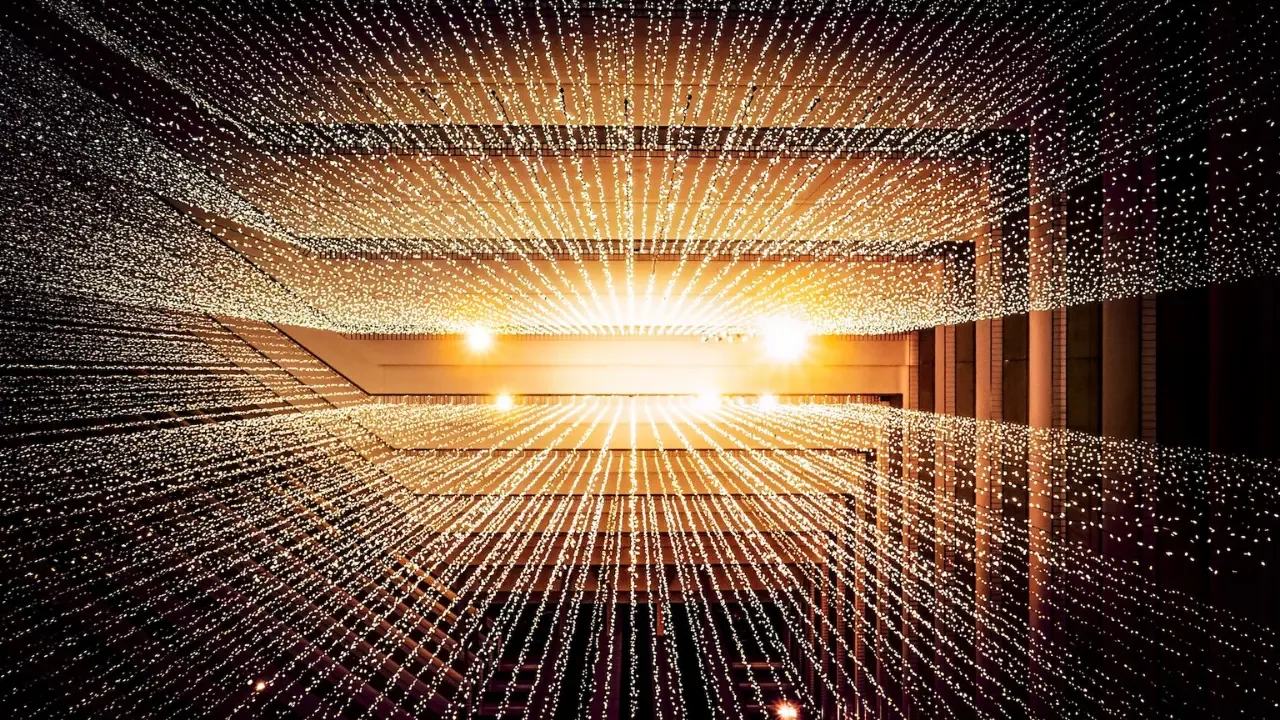
How to Ignore Invalid Self-Signed SSL Certificates in Node.js with https.request
So, you're building a cool app that needs to log into your local wireless router, but you're running into a roadblock with the router's self-signed SSL certificate. 😩 Don't worry, we've got you covered! In this guide, we'll walk you through the common issue of dealing with self-signed SSL certificates in Node.js and provide you with an easy solution. Let's dive in! 💪
Understanding the Problem
When communicating with a server over HTTPS, Node.js verifies the SSL certificate presented by the server to ensure a secure connection. However, if the server is using a self-signed SSL certificate, Node.js will throw an error since it cannot verify the certificate's authenticity. This is a security measure to protect against potential malicious attacks.
The Error Message
In your case, when you try to access the router's local IP address (e.g., 192.168.1.1
), you receive the following error message from the wget
command:
ERROR: cannot verify 192.168.1.1's certificate, issued by `/C=US/ST=California/L=Irvine/O=Cisco-Linksys, LLC/OU=Division/CN=Linksys/emailAddress=support@linksys.com':
Self-signed certificate encountered.
ERROR: certificate common name `Linksys' doesn't match requested host name `192.168.1.1'.
To connect to 192.168.1.1 insecurely, use `--no-check-certificate'.
In your Node.js code, you catch the following error:
{ [Error: socket hang up] code: 'ECONNRESET' }
The Solution: Ignoring the SSL Certificate Verification
To ignore the SSL certificate verification in Node.js, you can set the rejectUnauthorized
option to false
when making the https.request
call. This tells Node.js to bypass the certificate check and establish an insecure connection.
Here's an updated version of your sample code:
const https = require('https');
const options = {
host: '192.168.1.1',
port: 443,
path: '/',
method: 'GET',
rejectUnauthorized: false, // Ignore SSL certificate verification
};
const req = https.request(options, (res) => {
let body = [];
res.on('data', (data) => {
body.push(data);
});
res.on('end', () => {
console.log(body.join(''));
});
});
req.end();
req.on('error', (err) => {
console.log(err);
});
By including rejectUnauthorized: false
in the options
object, Node.js will no longer throw an error when encountering a self-signed SSL certificate. Just keep in mind that this approach sacrifices some security, as the connection is no longer fully secure.
Stay Informed and Engage!
Congratulations! You've successfully learned how to ignore invalid self-signed SSL certificates in Node.js. Remember to use this technique responsibly and only when dealing with trusted environments. 👍
If you found this guide helpful, feel free to share it with your fellow developers who might be facing similar challenges. Also, we'd love to hear from you! Share your experience or ask any further questions in the comments below.
Keep coding, stay secure, and happy hacking! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
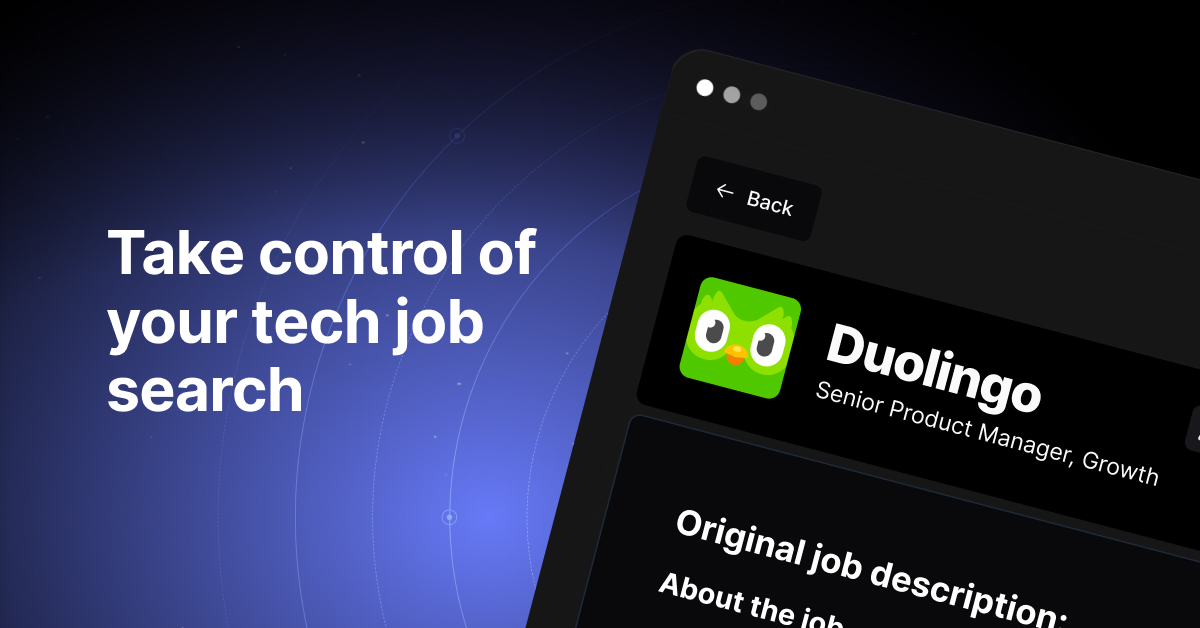