How to sort a collection by date in MongoDB?
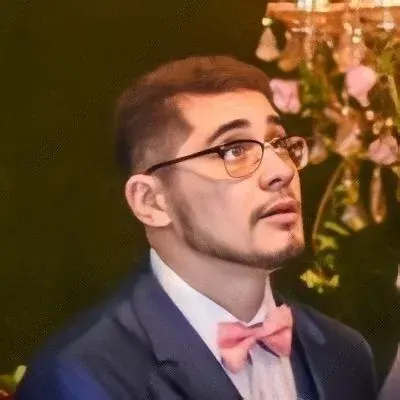
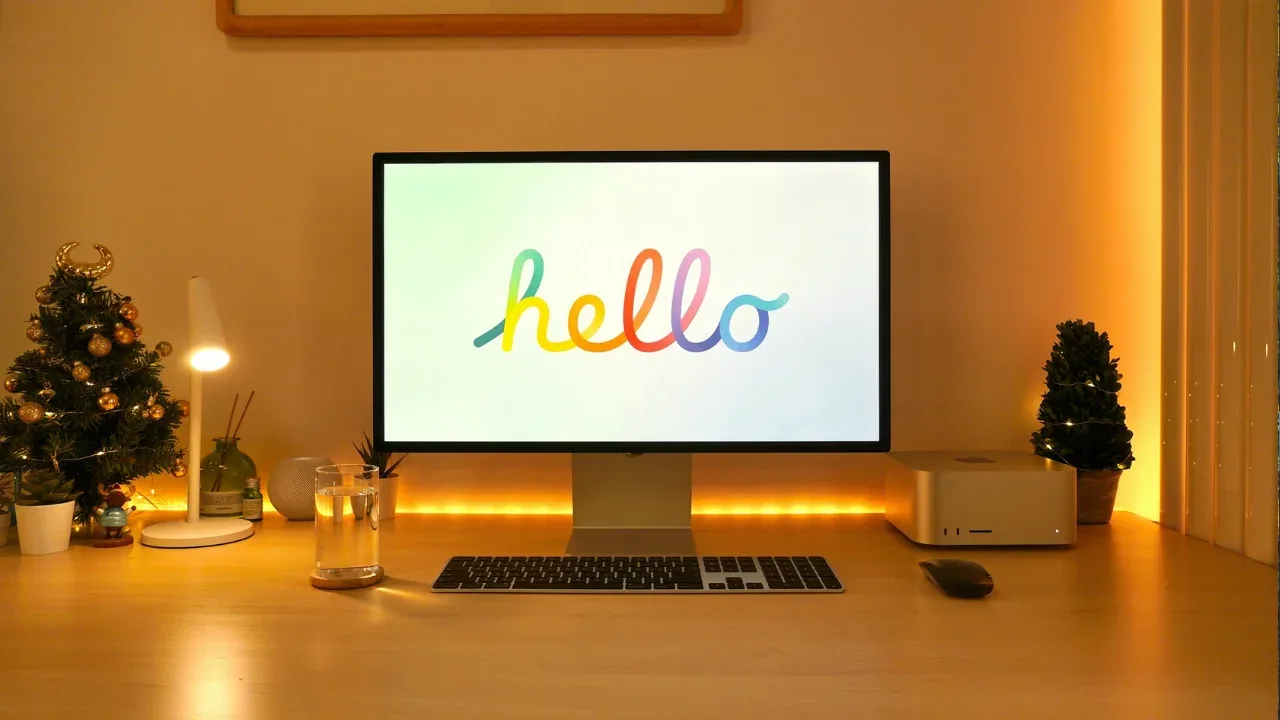
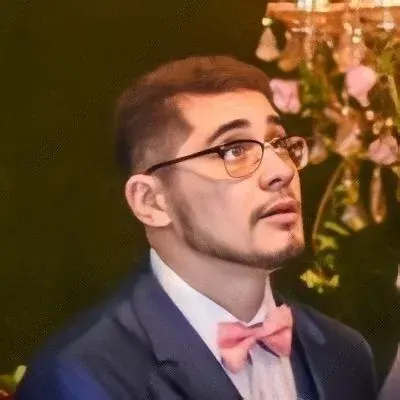
Sorting a Collection by Date in MongoDB: A Simple Guide 📅
MongoDB is a powerful NoSQL database that offers great flexibility in data handling. If you're using MongoDB with Node.js and need to sort a collection by date, you've come to the right place! Sorting collections by date can be a bit tricky, but fret not, we're here to lend a helping hand. In this guide, we'll walk you through the common issues you may encounter and provide easy solutions to sort a collection by date in MongoDB.
Understanding the Issue 🤔
In MongoDB, sorting documents by date can be a challenge when the dates are stored as JavaScript Date
objects. To sort a collection by date, we need to consider the limitations of sorting on non-native data types.
Solution 1: Storing Dates as ISOStrings 📝
One simple way to sort a collection by date is by storing the date values as ISO 8601 formatted strings. The ISOString format ensures both readability and ordering, making it a great choice for sorting.
To convert a JavaScript Date
object to an ISOString before inserting it into MongoDB, use the toISOString()
method:
const date = new Date();
const isoString = date.toISOString();
Now, when you insert the document into your MongoDB collection, the date will be stored as an ISOString:
db.collection.insertOne({date: isoString});
To retrieve the documents sorted by date, you can simply use the sort
method with the date
field:
db.collection.find().sort({date: 1});
Easy peasy! Your collection should now be sorted by date.
Solution 2: Using the Aggregation Framework 🔄
If you prefer to keep your dates as JavaScript Date
objects but still need to sort the collection, MongoDB's Aggregation Framework can come to the rescue.
The Aggregation Framework allows us to manipulate and transform documents, providing powerful sorting capabilities. To sort the collection by date using the Aggregation Framework, we can use the $sort
operator along with the $dateToString
operator.
Here's how it works:
db.collection.aggregate([
{
$sort: {
date: 1
}
}
]);
Voila! Your collection will be sorted based on the date
field.
Conclusion and Call-to-Action ✅
Sorting a collection by date in MongoDB may seem challenging, but with the right approach and a little know-how, you'll have your data sorted in no time. Whether you choose to store dates as ISOStrings or leverage the Aggregation Framework, MongoDB provides the tools you need for efficient sorting.
We hope this guide has helped you conquer the daunting task of sorting a collection by date in MongoDB. If you found this article useful, make sure to share it with fellow developers facing the same issue. Happy coding! 💻🚀
Have you faced any challenges sorting collections in MongoDB? Share your experience in the comments below! We'd love to hear from you.