How to get all count of mongoose model?
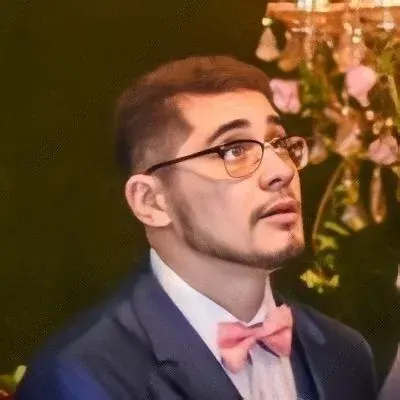
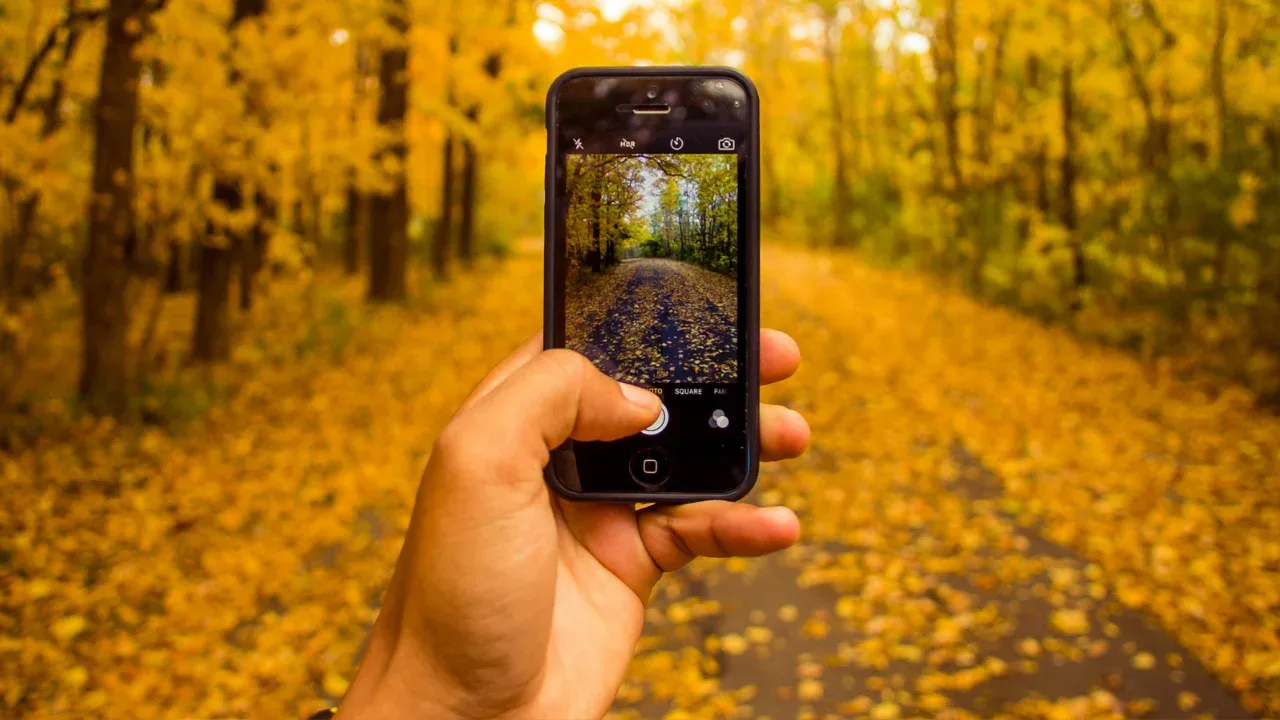
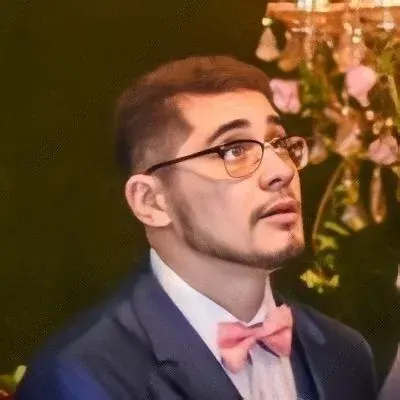
How to Get the Count of Mongoose Model? 📚
Are you struggling to find a way to get the count of a Mongoose model in your MongoDB database? 🤔 You're not alone! Many developers face this common issue when working with Mongoose. But worry not, we've got you covered. In this blog post, we'll walk you through the problem, provide easy solutions, and even give you a cool call-to-action at the end. Let's dive in! 🚀
The Problem 🤷♂️
So, you've tried using the Model.count()
method provided by Mongoose, but it doesn't seem to work in your case. 🤔 You've ended up with an object instead of the count you were expecting. What gives?
The Solution 💡
The issue here is that the Model.count()
method in Mongoose is deprecated as of Mongoose 5.9. Instead, you should use the Model.countDocuments()
method, which provides the functionality you need. Let's take a look at how you can use it:
const db = mongoose.connect('mongodb://localhost/myApp');
const userSchema = new Schema({ name: String, password: String });
const userModel = db.model('UserList', userSchema);
userModel.countDocuments({}, (err, count) => {
if (err) {
console.error(err);
} else {
console.log(`There are ${count} users in the database.`);
}
});
Here, we're using the countDocuments()
method on the userModel
, and passing an empty object {}
as the filter parameter to get the count of all documents in the collection.
The callback function receives two arguments: err
and count
. If any error occurs during the count operation, it will be available in the err
parameter. Otherwise, you'll get the count value in the count
parameter.
A Cool Trick: Async/Await ✨
If you prefer a more modern and cleaner syntax, you can also use async/await
with the same functionality. Here's how it looks:
const countUsers = async () => {
try {
const count = await userModel.countDocuments({});
console.log(`There are ${count} users in the database.`);
} catch (err) {
console.error(err);
}
};
countUsers();
Using async/await
makes the code look more concise and allows you to handle errors in a cleaner way. Feel free to choose the style that suits you best!
Your Turn! 🙌
Now that you've learned the correct method to get the count of a Mongoose model, it's time to put it into practice! 🚀 Go ahead and replace your existing Model.count()
with the Model.countDocuments()
method in your code.
If you have any other questions or want to share your experience, leave a comment below. We'd love to hear from you! 😊
Happy coding! 💻💡
Thanks for reading! If you found this post helpful, don't forget to share it with your fellow developers! 🎉