How to determine a user"s IP address in node
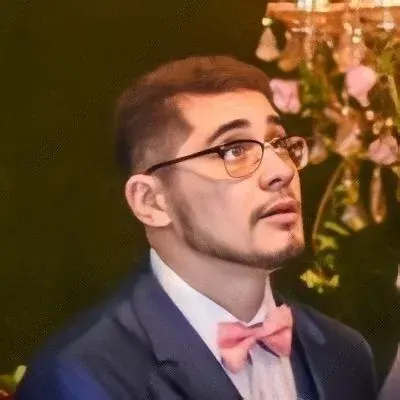
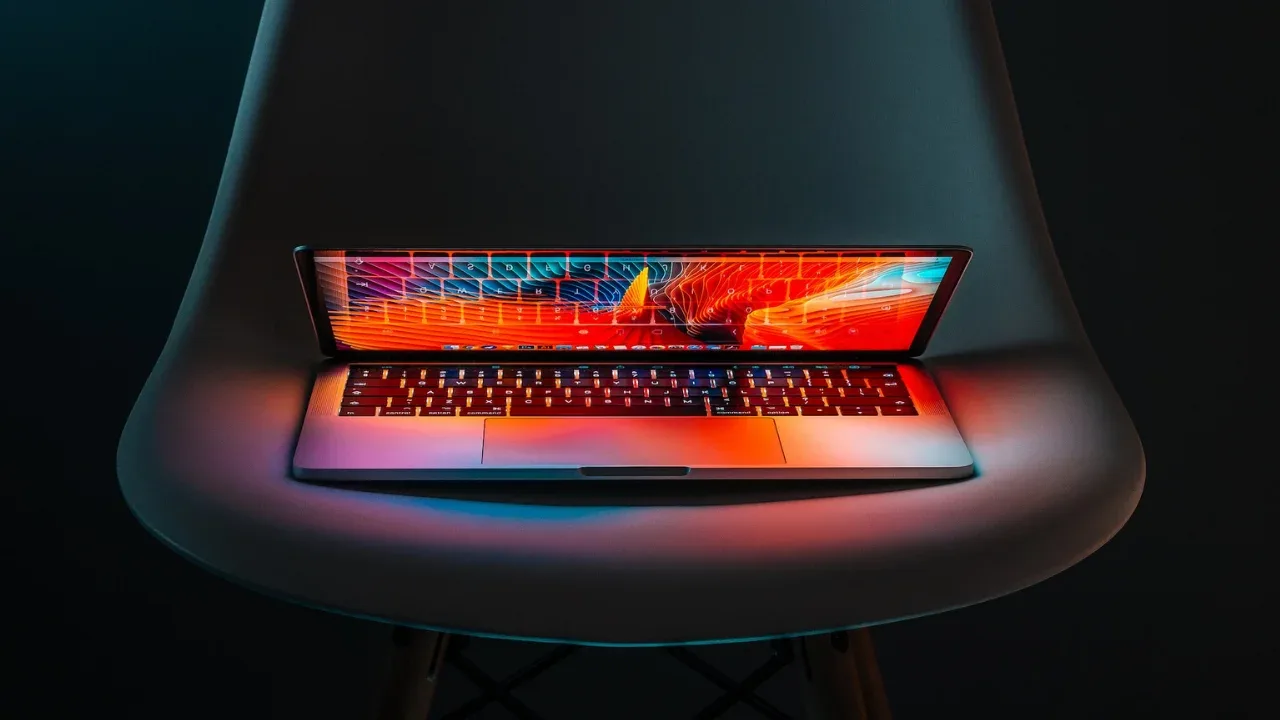
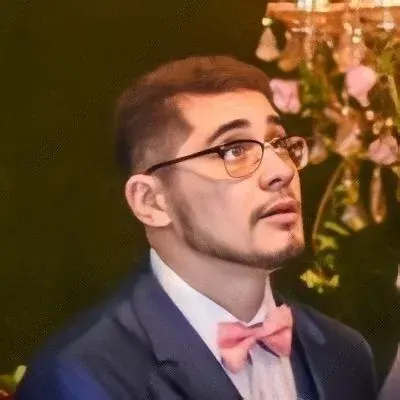
🌐 How to Determine a User's IP Address in Node 🖥️
So you're building a web application with Node.js and you find yourself in a situation where you need to determine the IP address of a user making a request. 🤔 Fear not! I've got you covered. In this blog post, I'll guide you through the process of retrieving a user's IP address in Node.js, specifically in an Express application. Let's dive in! 💻
📦 Setting Up Express
Before we can start retrieving the IP address, we need to have Express installed in our project. If you haven't already, open up your terminal and run the following command:
npm install express
Once that's done, we can proceed to set up our Express application.
🔎 Accessing the IP Address
In Express, the request object (req
) represents the HTTP request made by the client. Luckily, it contains a property called ip
that holds the IP address of the client making the request. To access this property, we can use req.ip
. Let's see this in action:
const express = require('express');
const app = express();
app.post('/get/ip/address', function (req, res) {
const ipAddress = req.ip;
// Now you have access to the IP address! 🚀
});
app.listen(3000, function () {
console.log('Server started on port 3000');
});
By assigning req.ip
to the ipAddress
variable, we retrieve the IP address of the user making the request. Easy peasy! 🎉
🐛 Dealing with Proxy Servers
If your application operates behind a proxy server, you may find that req.ip
returns the IP address of the proxy server itself instead of the user's actual IP address. To get the real IP address in these situations, you can rely on an additional package called proxy-addr
. Start by installing it using the following command:
npm install proxy-addr
Once installed, update your code as follows:
const express = require('express');
const app = express();
const proxyAddr = require('proxy-addr');
app.set('trust proxy', true);
app.post('/get/ip/address', function (req, res) {
const ipAddress = proxyAddr(req, true);
// Now you have the real IP address, even behind a proxy! 🚀
});
app.listen(3000, function () {
console.log('Server started on port 3000');
});
By setting app.set('trust proxy', true)
, Express will trust proxies in front of the application and interpret req.ip
correctly.
📣 Join the Conversation!
Determining a user's IP address is a fundamental aspect of many web applications. Now that you have a solid understanding of how to retrieve it in Node.js, why not share your thoughts and experiences with us? Have you encountered any challenges while retrieving IP addresses? What creative solutions have you implemented? Join the conversation in the comments section below! 👇
Remember, building great web applications is not just about coding, but also about learning from others and fostering a supportive community. We can't wait to hear what you have to say!
Happy coding! 😄🚀