How to Create and Use Enum in Mongoose
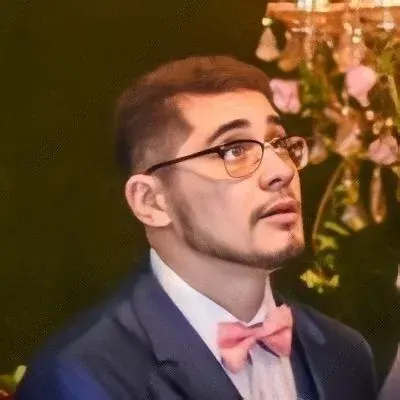
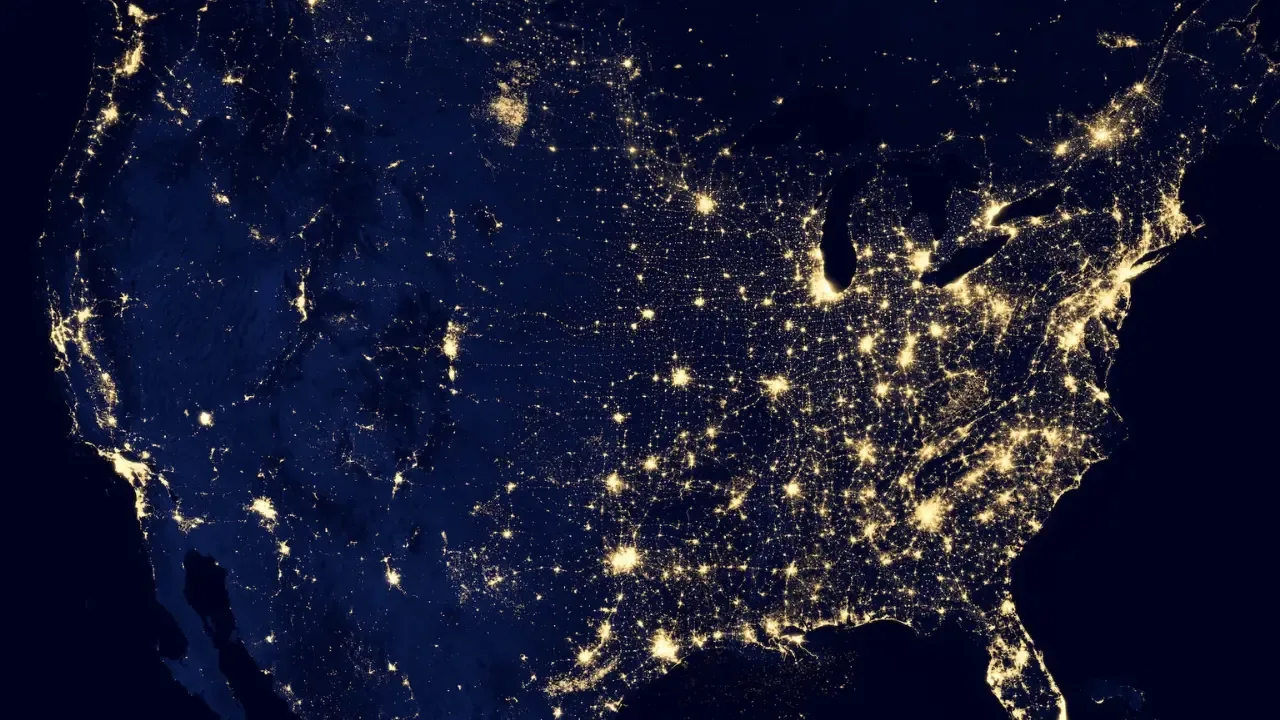
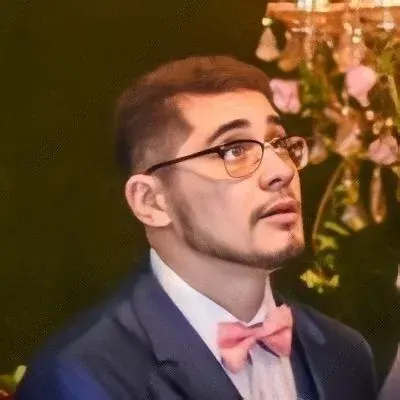
How to Create and Use Enum in Mongoose
š Are you trying to use an enum type in Mongoose, but not getting the desired result? Don't worry, I've got you covered! In this blog post, I'll explain how to create and use enums in Mongoose, and help you understand common issues you might encounter along the way.
š¤ Let's start by understanding the problem at hand. The user is trying to use an enum in their Mongoose schema, but they're confused about how to define the values and store them properly in the database.
š To better understand the problem, let's take a look at the code snippet provided:
var RequirementSchema = new mongooseSchema({
status: {
type: String,
enum: ['NEW,'STATUS'],
default: 'NEW'
},
})
š© The issue here is that the enum values are not properly defined. When defining an enum in Mongoose, each value should be enclosed in quotes and separated by commas. In the code snippet, the enum values are missing the closing quote after 'NEW'. To fix this issue, the correct code snippet would be:
var RequirementSchema = new mongooseSchema({
status: {
type: String,
enum: ['NEW', 'STATUS'], // Fix: Added closing quote after 'NEW'
default: 'NEW'
},
})
š Now that we've addressed the immediate issue, let's dive deeper into how to create and use enums in Mongoose.
Creating an Enum in Mongoose
š§ To create an enum in Mongoose, you need to define an array of string values that represent the valid options for a specific field. These options will serve as the only allowed values.
var schema = new mongoose.Schema({
fieldName: {
type: String,
enum: ['option1', 'option2', 'option3']
}
})
š In the example above, the fieldName
can only accept values from the defined enum array: 'option1', 'option2', and 'option3'. Any other value will be rejected by Mongoose.
š” Enum values should generally be descriptive and meaningful. Choose values that accurately represent the field they are associated with.
Using Enums in Mongoose
šØ Once you have defined an enum for a field in your schema, you can use it just like any other Mongoose schema type.
var ExampleModel = mongoose.model('Example', schema);
var exampleInstance = new ExampleModel({
fieldName: 'option1' // The value must be one of the enum options
});
exampleInstance.save(function(err) {
// Handle save callback
});
š You can now create, save, and query documents using the enum values defined in your Mongoose schema.
Benefits of Using Enums
š Enums offer several benefits in database modeling. They provide a predefined set of options, ensuring data consistency and reducing the chances of invalid or conflicting values.
š Enums can also act as a form of data validation, making it easier to enforce restrictions on specific fields.
š Additionally, enums can improve the readability of your code. When the accepted values are clearly defined, it becomes easier to understand the purpose and intent of each field.
š So, next time you encounter a situation where you need to restrict the value options for a field, consider using enums in your Mongoose schema.
š£ If you found this blog post helpful, feel free to share it with your fellow developers. And don't forget to leave a comment below if you have any questions or additional insights to share. Happy coding! š