How is an HTTP POST request made in node.js?
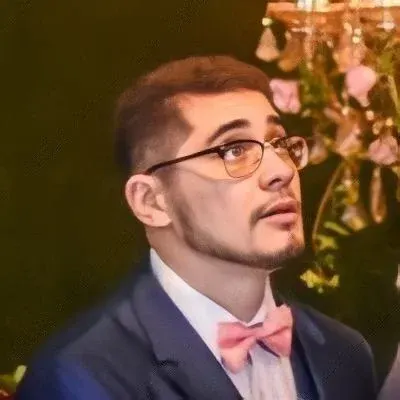
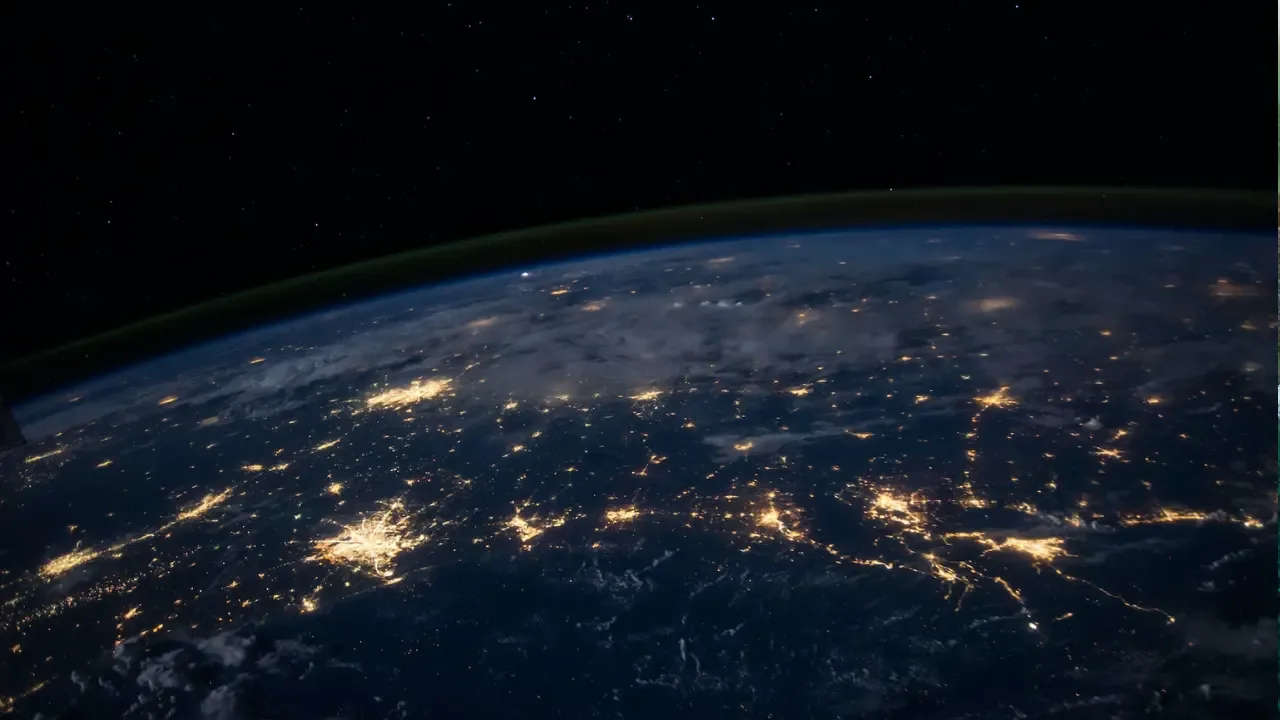
🚀🌐 Making an HTTP POST Request in Node.js: A Simple Guide 📝🎉
Introduction 📢
Hey there, tech enthusiasts! 😃 Are you struggling with making an outbound HTTP POST request in Node.js? Don't worry, we've got your back! In this blog post, we'll guide you through the process and help you overcome any common issues you might encounter along the way. Let's dive right in! 💪🏼
Understanding the HTTP POST Request 🕵️♀️
To make an HTTP POST request in Node.js, we need to understand the basics. The HTTP POST method allows us to send data to the server and create a new resource. Unlike the GET method, which retrieves data, the POST method is used for submitting data.
The Code Snippet ✍️
const http = require('http');
const postData = 'This is the data to be sent';
const options = {
hostname: 'www.example.com',
port: 80,
path: '/your-endpoint',
method: 'POST',
headers: {
'Content-Type': 'text/plain',
'Content-Length': Buffer.byteLength(postData)
}
};
const req = http.request(options, (res) => {
console.log(`Status Code: ${res.statusCode}`);
res.on('data', (chunk) => {
console.log(`Response Data: ${chunk}`);
});
});
req.on('error', (error) => {
console.error(`Error: ${error.message}`);
});
req.write(postData);
req.end();
Deconstructing the Code 💡
Let's break down the code snippet above:
We start by requiring the
http
module, which allows us to work with HTTP requests in Node.js.We define the
postData
variable to hold the data we want to send with our POST request.Next, we create an
options
object with details such as hostname, port, path, method, and headers.We call
http.request()
method with the options object and handle the response in a callback function.Inside the callback function, we handle the response data by listening to the
'data'
event. Here, we log the response data to the console.We handle any errors that might occur during the request using the
'error'
event.Finally, we use
req.write()
to send the postData andreq.end()
to complete the request.
That's it! With this code snippet, you can make a successful HTTP POST request in Node.js.
Common Issues and Solutions 🛠️
Handling JSON Data: If you want to send data in JSON format, update the
'Content-Type'
header to'application/json'
and useJSON.stringify()
to convert your data to a string before sending it.Handling Different Encodings: If your data is not in plain text format, such as binary or base64, you need to update the
'Content-Type'
header accordingly and encode your data before sending it.HTTPS Requests: If you want to make an HTTPS POST request instead, replace
http
with thehttps
module, and adjust the port number and other settings accordingly.
Call-to-Action and Conclusion 📣🔚
Voila! You've now learned how to make an HTTP POST request in Node.js. We hope this guide helped you overcome any challenges you faced. Now, it's your turn to try it out and create amazing things with your newfound knowledge! 💡
Have any questions or encountered any problems? We'd be more than happy to help you out. Share your thoughts, experiences, or any other handy tips in the comments section below. Let's learn and grow together! 🚀🌟
Happy coding! 💻✨
Note: Remember to npm install the required modules (http or https) before running the code.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
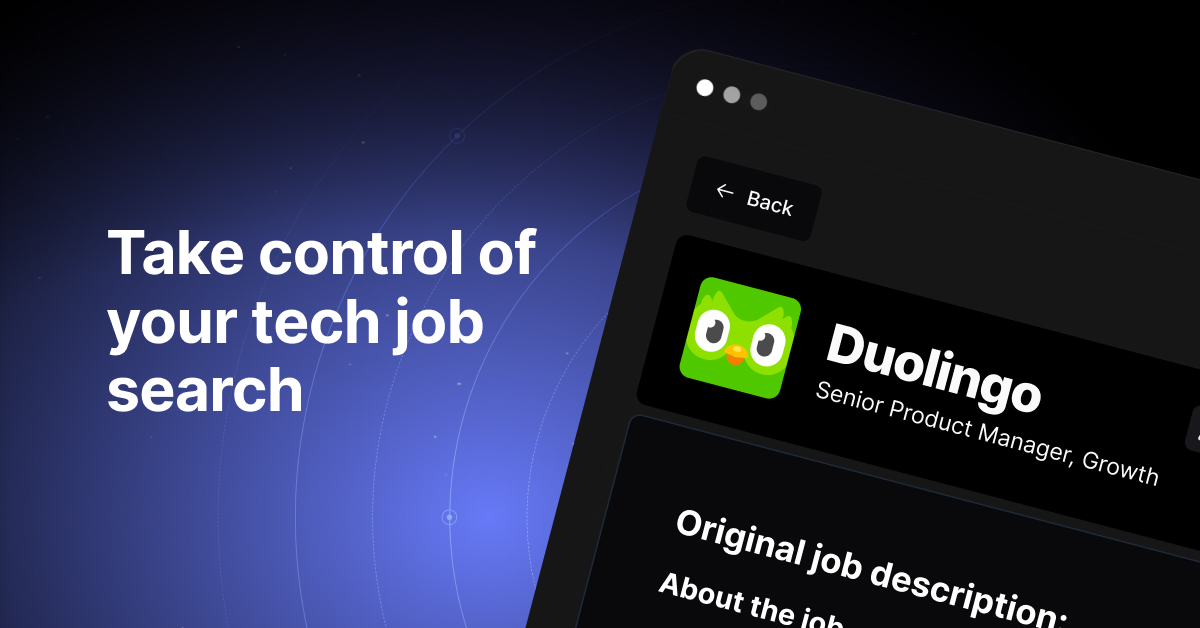