How do I manage MongoDB connections in a Node.js web application?
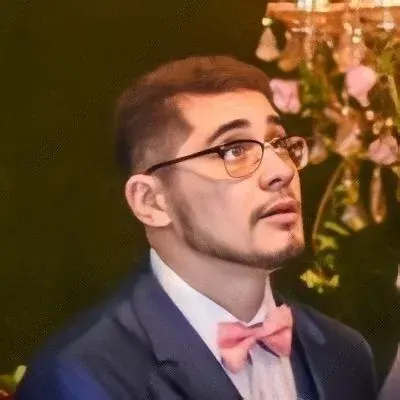
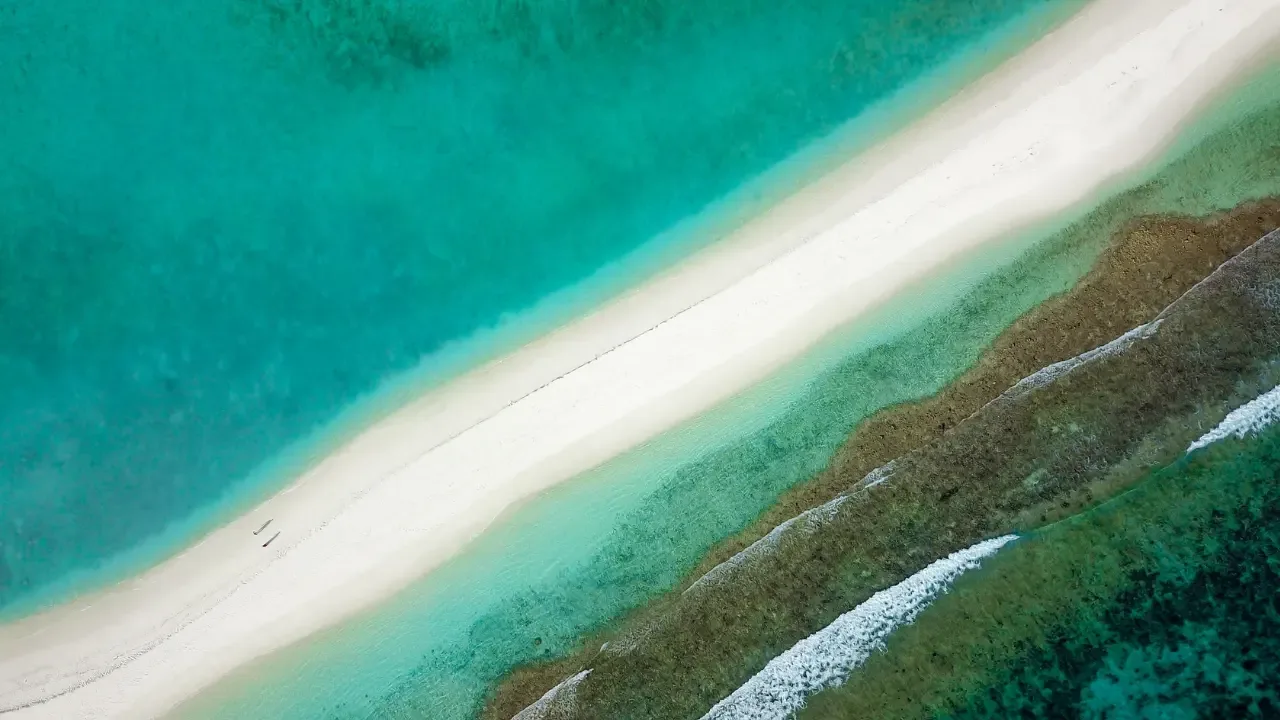
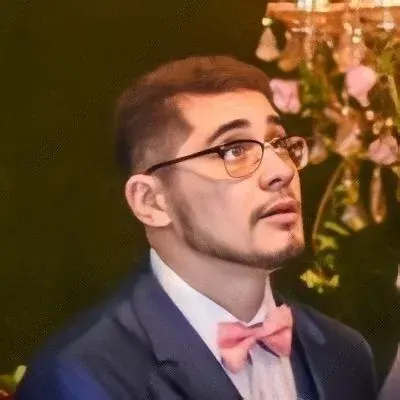
How to Efficiently Manage MongoDB Connections in a Node.js Web Application 🌐🔗
So you're writing a website using MongoDB with the node-mongodb-native driver, and now you find yourself wondering how to effectively manage your connections. Don't worry, we've got you covered! In this guide, we'll address common concerns and provide easy-to-implement solutions. Let's dive in! 💪🏼
1️⃣ Using a Single MongoDB Connection?
It's a common question whether using a single MongoDB connection for all requests will suffice. The good news is that yes, it can be enough! Creating a global connection can save you from unnecessary overhead. 😌✨
const { MongoClient } = require('mongodb');
// Create a global MongoDB connection
const uri = 'your-mongodb-uri';
const client = new MongoClient(uri, { useUnifiedTopology: true });
async function connectToMongo() {
try {
await client.connect();
console.log('Connected to MongoDB successfully!');
} catch (error) {
console.error('Failed to connect to MongoDB:', error);
}
}
module.exports = { client, connectToMongo };
2️⃣ Opening and Closing Connections?
If you decide to use a global connection, there's no need to open and close it for each request. This way, you save on the expense of establishing a new connection every time. However, you should handle reconnection and error scenarios gracefully in case the connection is lost. 💔
3️⃣ Utilizing the Connection Pool
For more advanced use cases, it's recommended to use the native connection pool provided by the node-mongodb-native driver. This can greatly enhance your application's scalability and performance. 🚀💯
const { MongoClient } = require('mongodb');
// Create a global connection pool
const uri = 'your-mongodb-uri';
const client = new MongoClient(uri, {
useUnifiedTopology: true,
maxPoolSize: 10, // Maximum number of connections in the pool
});
async function connectToMongo() {
try {
await client.connect();
console.log('Connected to MongoDB successfully!');
} catch (error) {
console.error('Failed to connect to MongoDB:', error);
}
}
module.exports = { client, connectToMongo };
4️⃣ How Many Connections Should I Use?
Determining the optimal number of connections depends on various factors such as the expected concurrency and workload of your application. A good starting point is to set the maxPoolSize
to a value that accommodates your anticipated traffic. You can always monitor and adjust this value as needed. 📈
5️⃣ Additional Considerations
Here are a few things to keep in mind when managing MongoDB connections in your Node.js web application:
Make sure to handle connection errors and automatic reconnections properly to ensure uninterrupted service. 💡
Be cautious when sharing connections of different users in a multi-tenant environment. Data isolation and security should be taken into account. 🔒
Consider implementing connection timeouts to prevent idle connections from consuming unnecessary resources. 💤⏳
Leverage connection pooling and connection reuse to improve performance and reduce latency in your application. 🏊♂️💨
Now that you have a better understanding of managing MongoDB connections in your Node.js web application, it's time to put this knowledge into action! Have fun building your MongoDB-powered website, and don't hesitate to reach out if you need further assistance. Happy coding! 🎉🚀
Let us know in the comments how you're managing your MongoDB connections or if you have any specific questions. We'd love to hear from you! 💬❓