How do I debug "Error: spawn ENOENT" on node.js?
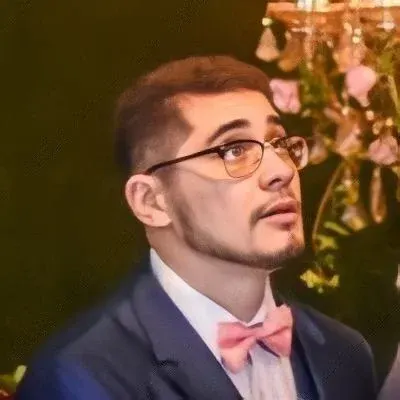
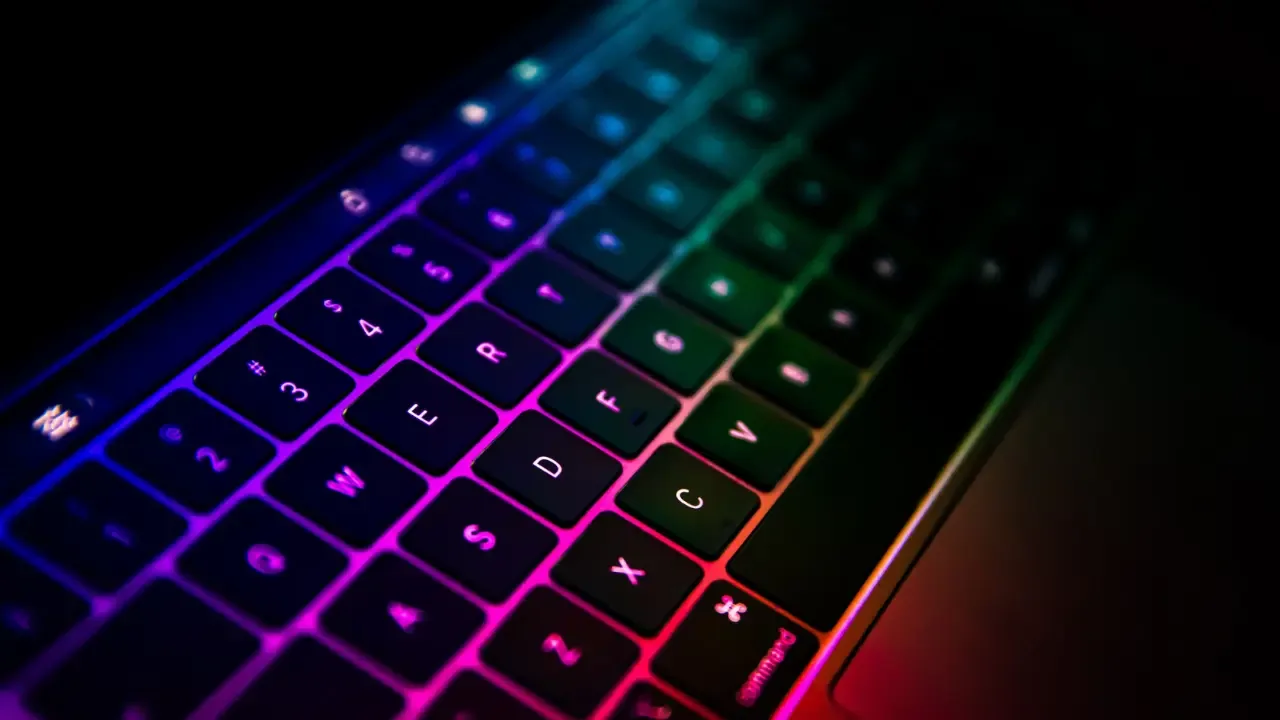
How to Debug "Error: spawn ENOENT" on Node.js? š»š
š Hey there! If you're seeing the dreaded "Error: spawn ENOENT" in your Node.js application, don't panic! This is a common issue that many developers encounter when working with child processes. In this blog post, we'll dive into the root causes of this error and provide you with easy-to-follow solutions to debug and fix it. Let's get started! š
Understanding the Error šµļøāāļø
When you encounter the "Error: spawn ENOENT" message, it means that the child process you're trying to spawn (execute) could not be found. This can happen due to various reasons, such as:
The command or executable you're trying to run doesn't exist.
The command or executable is not in the system's PATH.
There are permission issues preventing the execution of the command.
The environment variables are not correctly set up.
How to Debug the Issue š
To debug the "Error: spawn ENOENT" error, follow these steps:
1. Check if the Command or Executable Exists š
Verify whether the command or executable you're trying to run actually exists on your system. For example, if you're trying to execute the command npm install
, make sure that npm
is installed on your machine.
2. Check the System's PATH Variable š£ļø
The system's PATH variable contains a list of directories where executables are located. Ensure that the directory containing the command or executable you're trying to run is included in the system's PATH. If it's not, you can add it manually or reinstall the command-line tool to automatically update the PATH variable.
3. Verify Permissions š
If you're facing permission issues, make sure that the user running the Node.js application has the necessary permissions to execute the command or executable. You may need to grant executable permissions to the file or use the appropriate user when running the application.
4. Check Environment Variables š
Certain child processes rely on environment variables to function correctly. Ensure that the required environment variables are correctly set up. You can check this by logging the values of the relevant environment variables before spawning the child process.
5. Print Detailed Error Information š
To get more information about the exact cause of the "Error: spawn ENOENT" error, you can listen for the 'error' event on the child process. By logging the error details, you can gain insights into the specific problem and troubleshoot accordingly.
const { spawn } = require('child_process');
const childProcess = spawn('your-command');
childProcess.on('error', (error) => {
console.error('Failed to spawn command:', error);
});
Conclusion and Next Steps š
You made it! Now you know how to debug and fix the annoying "Error: spawn ENOENT" on Node.js. By following the steps outlined above, you can identify the root cause of the error and take appropriate action.
I hope this guide was helpful to you! If you have any questions or encountered any other errors, feel free to reach out. Happy coding! āØš»
š¦š¦š¦ Additional Resources
To learn more about debugging and troubleshooting in Node.js, check out these helpful resources:
Stack Overflow: using spawn function with NODE_ENV=production
Stack Overflow: node.js child_process.spawn ENOENT error - only under supervisord
Stack Overflow: nodejs spawn ENOENT error on Travis calling global npm package
Stack Overflow: Node JS - child_process spawn('npm install') in Grunt task results in ENOENT error
Remember, the community is always there to support you! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
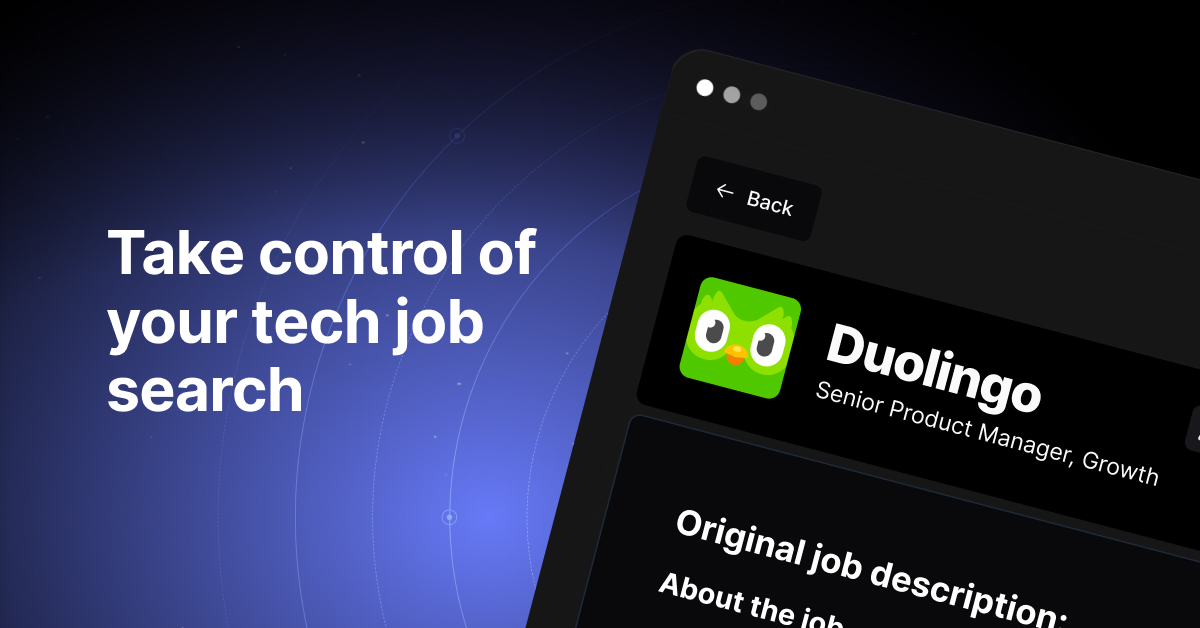