How can I use an ES6 import in Node.js?
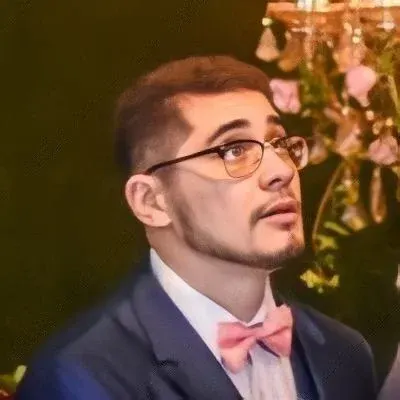
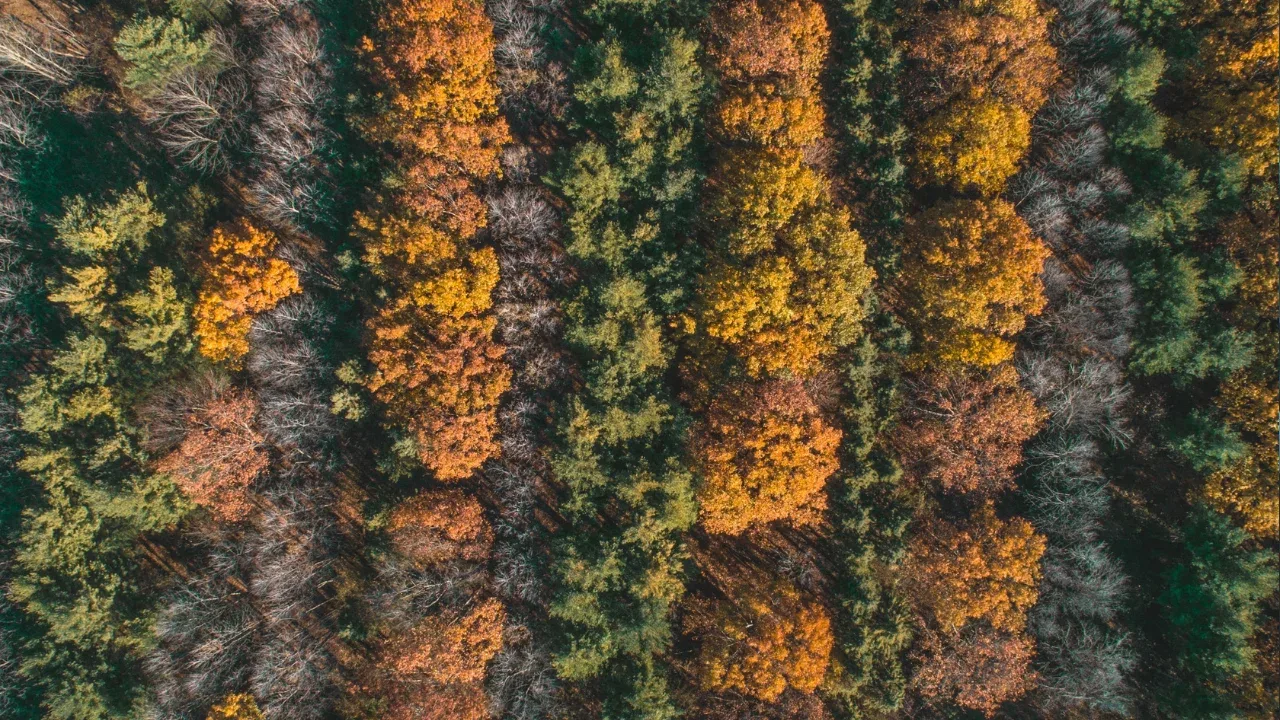
How to Use ES6 Imports in Node.js: A Comprehensive Guide
š Hey there! Are you facing issues trying to use ES6 imports in Node.js? You're not alone! Many developers run into this problem because by default, Node.js does not support ES6 module syntax. But fret not, my friend! In this blog post, we'll walk you through the process of using ES6 imports in Node.js, address common problems, and provide easy solutions. So let's dive right in! š
Background Information
Before we get into the nitty-gritty of using ES6 imports in Node.js, let's start with some background information. ES6 (ECMAScript 2015) introduced a new syntax for importing and exporting modules, which could not be directly used in Node.js until recently. However, Node.js has its own require
function for module imports, which uses CommonJS syntax. This is where the confusion arises. But fear not, we have a solution! šŖ
The Problem
You've probably stumbled upon some tutorial or code snippet that uses the ES6 import syntax, something like this:
import { square, diag } from 'lib';
But when you try running it in your Node.js environment, you encounter the dreaded error: SyntaxError: Unexpected token import
. š±
The Solution
To resolve this issue and enable ES6 imports in your Node.js code, follow these simple steps:
Update your Node.js version: š First things first, ensure that you have a Node.js version that supports ES6 module syntax. Take a look at the Node.green support table to find out which versions have native support for ES6 imports. If your current version does not support it, upgrade to a version that does. We recommend using Node.js 12.x or above for seamless ES6 imports.
Use the
--experimental-modules
flag: š© Once you have a compatible version of Node.js, you need to enable experimental module support using the--experimental-modules
flag. This flag allows you to use ES6 imports in your Node.js code. Here's an example of how you can run your file with the flag:node --experimental-modules your-file.js
Update file extension to
.mjs
: š By default, Node.js treats files with the.js
extension as CommonJS modules. To use ES6 imports, rename your file extension from.js
to.mjs
. This tells Node.js that the file should be treated as an ES6 module.Update import/export statements: š Now, you need to update your import and export statements in your code to use ES6 syntax. For example, change this:
const sqrt = Math.sqrt; export function square(x) { return x * x; } export function diag(x, y) { return sqrt(square(x) + square(y)); }
to this:
export const sqrt = Math.sqrt; export function square(x) { return x * x; } export function diag(x, y) { return sqrt(square(x) + square(y)); }
And that's it, my friend! You now have the power of ES6 imports in your Node.js code. Say goodbye to the SyntaxError: Unexpected token import
error and embrace the beauty of modern JavaScript! š
Bonus Tip: Use Babel for Wider Compatibility
If you are working on a project that needs to support older versions of Node.js or browsers that do not have native support for ES6 imports, you can use Babel. Babel is a powerful tool that transpiles your code to a compatible format. By configuring Babel with the @babel/preset-env
plugin, you can write code using ES6 imports and have it transformed to CommonJS syntax, allowing it to run smoothly in older environments. š
Conclusion
I hope this guide has helped you understand how to use ES6 imports in Node.js and resolve any issues you were facing. Remember to upgrade your Node.js version, use the --experimental-modules
flag, rename your file extensions, and update your import/export statements. And if you need wider compatibility, give Babel a try!
If you have any questions, or if you've found any other cool tips to share, let us know in the comments below! We love hearing from fellow developers. š
Now go forth and code with the power of ES6 imports! Happy coding! āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
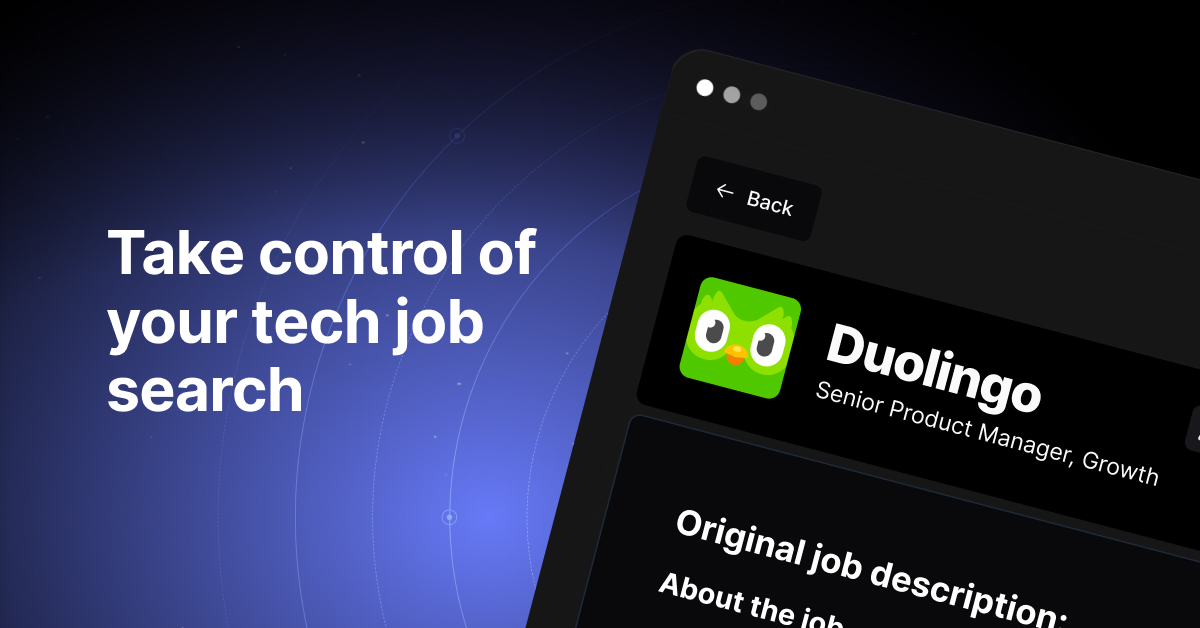