How can I generate an ObjectId with mongoose?
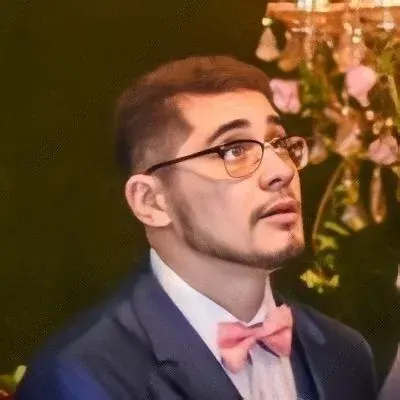
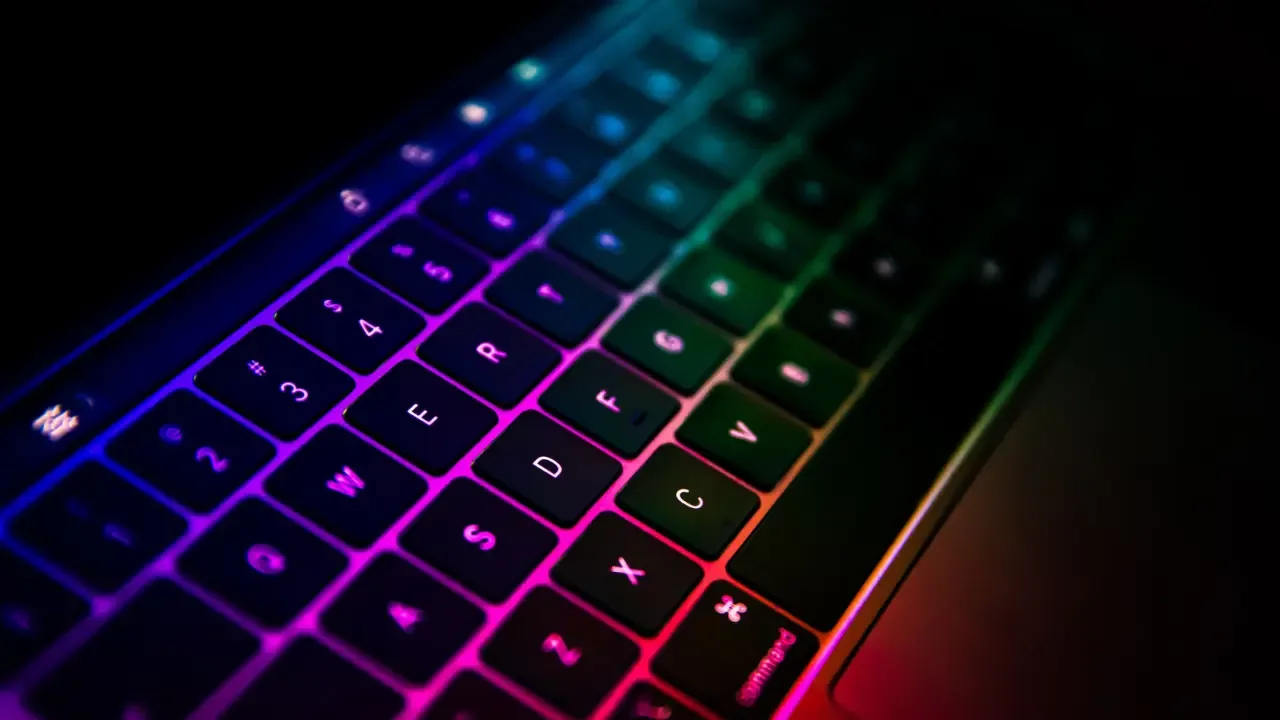
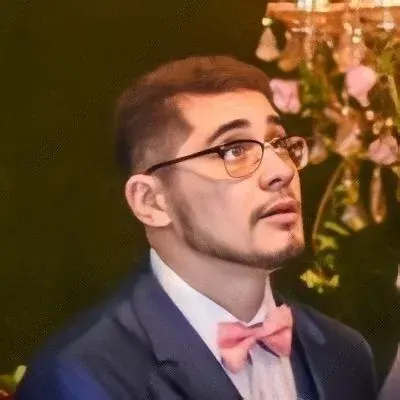
How to 👨💻 Generate an ObjectId with Mongoose?
So, you're looking for a way to generate a MongoDB ObjectId
with Mongoose, huh? 🤔 Well, you're in luck because I'm here to guide you through it! Let's dive right in. 🏊♂️
Generating a New ObjectId 🆕
This question specifically revolves around generating a new ObjectId
from scratch. This means you want to create a fresh, universally unique identifier. 💪
To achieve this, you can access the ObjectId
constructor provided by Mongoose. Here's how you can do it in your code:
const mongoose = require('mongoose');
const newId = new mongoose.Types.ObjectId();
console.log(newId);
By calling new mongoose.Types.ObjectId()
, you'll get a shiny new ObjectId
as a result. You can store it, use it, or impress your friends with it. 😎
Reusing an Existing String Representation 🔄
Now, let's address another common question. What if you already have a string representation of an ID and you want to create an ObjectId
from it? 🧐 Don't worry, Mongoose has got you covered! 🙌
const mongoose = require('mongoose');
const existingIdString = '5f6a84b9ee28c924c4e6f00a';
const existingObjectId = mongoose.Types.ObjectId(existingIdString);
console.log(existingObjectId);
In this example, we take an existing string representation of an ID and pass it as an argument to the mongoose.Types.ObjectId()
constructor. This will parse the string and create a valid ObjectId
for you.
Time for Action! ⚡️
Are you ready to generate some ObjectIds with Mongoose? Go ahead and give it a try in your projects! 💻 Don't forget to share your ObjectId generating skills with your fellow developers. Together, we can create a world full of unique identifiers! 🌍❤️
If you have any questions, thoughts, or even ObjectId-related stories you'd like to share, feel free to drop a comment below. Let's start an ObjectId-loving community! 🙌🎉