Get file name from absolute path in Nodejs?
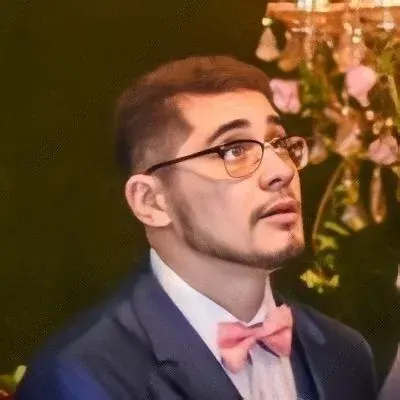
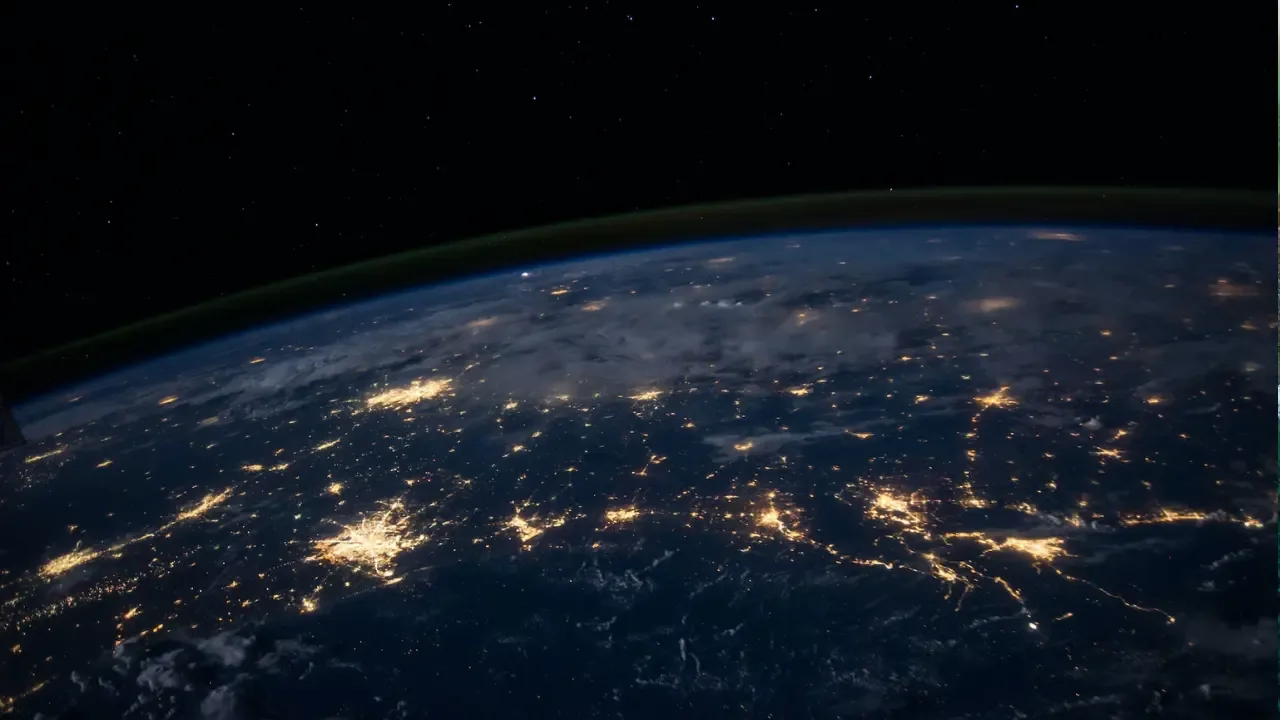
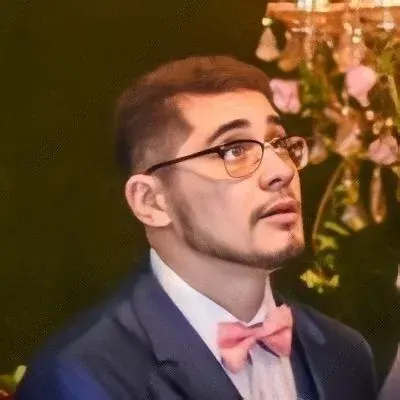
📜 Get File Name from Absolute Path in Node.js 🚀
Introduction
Have you ever found yourself in a situation where you need to extract the file name from an absolute path in Node.js? 🤔 Don't worry, you're not alone! Many developers face this challenge, but we're here to help you find a solution.
In this blog post, we'll explore different approaches to obtaining the file name from an absolute path in Node.js. We'll cover both string operations and explicit methods, so you can choose the approach that suits your needs best. Let's dive in! 💪
The Challenge
The problem at hand is extracting the file name from an absolute path, like '/var/www/foo.txt'. We want to obtain just 'foo.txt' from this string. 📝
Solution 1: String Operation
One approach is to use a simple string operation to extract the file name from the absolute path. Here's how you can do it in Node.js:
const filePath = '/var/www/foo.txt';
const fileName = filePath.replace(/.+\//, '');
console.log(fileName); // Output: foo.txt
In this approach, we use the replace
method with a regular expression to match everything before the last forward slash ('/'). By replacing that matched portion with an empty string, we end up with just the file name. 🎉
Solution 2: Using the Path Module
If you prefer a more explicit solution, you can use the path
module provided by Node.js. This module offers various path-related utilities, including a method to extract the file name. Here's how you can achieve it:
const path = require('path');
const filePath = '/var/www/foo.txt';
const fileName = path.basename(filePath);
console.log(fileName); // Output: foo.txt
In this solution, we import the path
module and use the basename
method to directly retrieve the file name from the absolute path. This method ensures cross-platform compatibility by handling different path delimiters.
Conclusion
Getting the file name from an absolute path in Node.js may seem daunting at first, but now you have two simple and effective solutions at your disposal.
If you prefer a concise solution, the string operation approach should serve you well. Just use the replace
method to remove the path before the file name.
On the other hand, if you are looking for a more explicit approach or cross-platform compatibility, the path
module is your best bet. Take advantage of the basename
method to extract the file name effortlessly.
I hope this guide has helped you in your Node.js development journey! If you have any questions or additional approaches, feel free to share them in the comments below. Happy coding! 🎉
Read more articles like this on our blog and stay tuned for exciting tech content! Don't forget to share this post with your fellow developers! 😃
Note: Remember to replace yourblogurl.com
with your actual blog URL and customize the call-to-action accordingly.