Get all directories within directory nodejs
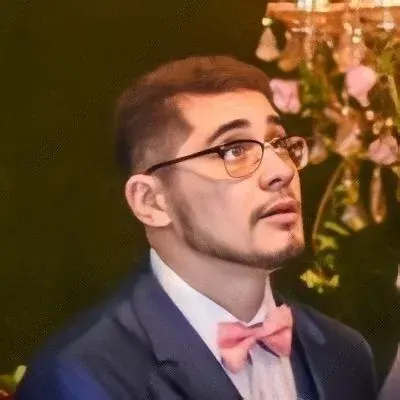
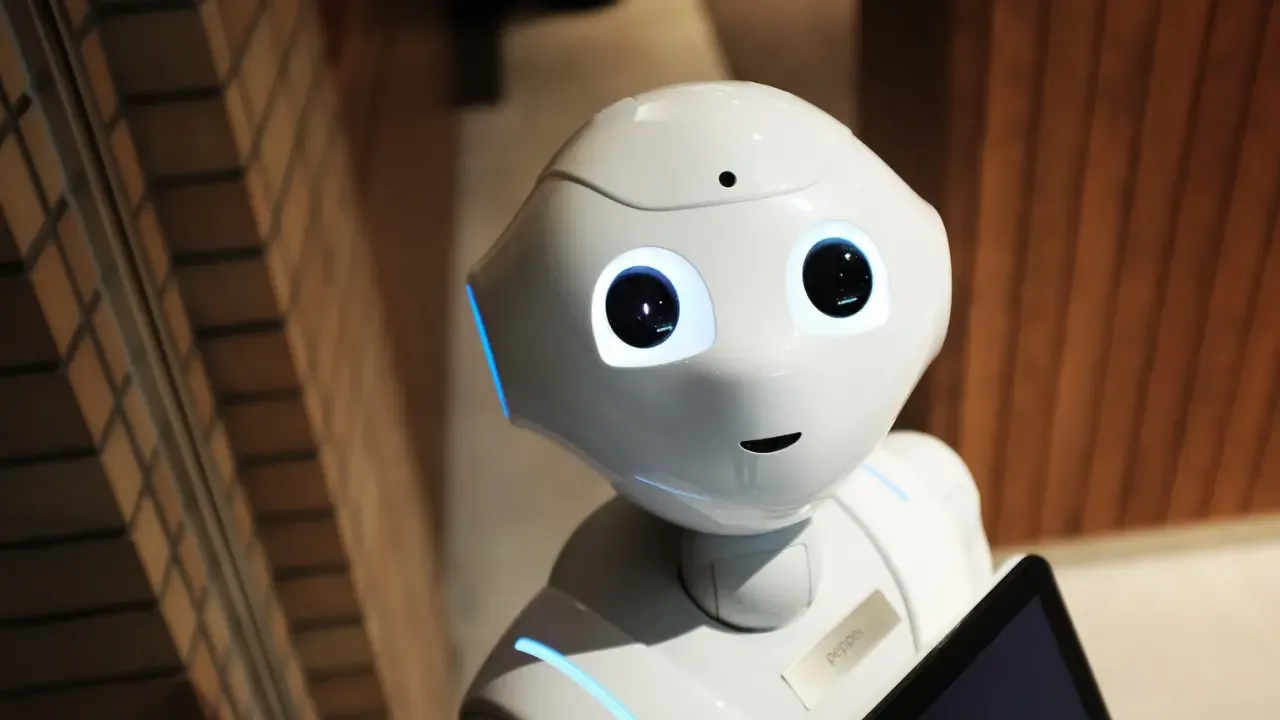
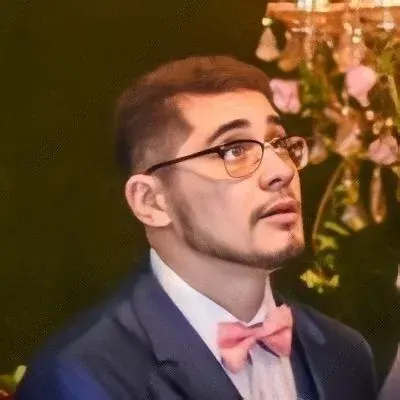
Get all Directories within a Directory in Node.js: A Handy Guide 📂
So, you're looking to retrieve all the directories within a directory in Node.js? You're not alone! This seemingly simple task can become a bit tricky without the right knowledge. But worry not, my dear reader! In this guide, we'll explore common issues, provide easy solutions, and help you accomplish this task effortlessly. Let's dive in! 🏊♂️
The Challenge 🎯
You want to obtain a list of all the directories within a given directory. Using your example, let's say we have the following structure:
<MyFolder>
|- SomeFolder
|- SomeOtherFolder
|- SomeFile.txt
|- SomeOtherFile.txt
|- x-directory
And your desired result is an array containing the directories: ["SomeFolder", "SomeOtherFolder", "x-directory"]
. Seems simple, right?
The Solution 💡
To achieve this, we need to leverage the power of Node.js and its built-in modules. The module we're particularly interested in is fs
(filesystem). Let's walk through the steps to obtain the desired result:
First, import the
fs
module in your Node.js script using therequire
function:
const fs = require('fs');
Next, define the path to the directory for which you want to retrieve the directories. In our case, it would be
<MyFolder>
.
const directoryPath = '<MyFolder>';
Now, we can use the
readdir
function from thefs
module to read the contents of the directory. This function takes two arguments: the directory path and a callback function.
fs.readdir(directoryPath, (err, files) => {
if (err) {
throw err;
}
// Handle files here
});
Inside the callback function, we can filter out the directories from the
files
array using theisDirectory
method of thefs.Stats
object.
fs.readdir(directoryPath, (err, files) => {
if (err) {
throw err;
}
const directories = files.filter((file) => {
return fs.statSync(directoryPath + '/' + file).isDirectory();
});
console.log(directories);
});
That's it! 🎉 Running your script should print out an array containing all the directories within the specified directory.
A Word of Caution ⚠️
Keep in mind that the above solution assumes a synchronous execution of fs.statSync
. If your directory contains a large number of files, it's recommended to use the asynchronous counterpart fs.stat
. Doing so will prevent blocking the event loop and provide a more efficient solution.
Your Turn to Code! 💻
Now that you've learned the ins and outs of retrieving directories within a directory in Node.js, it's time to put your newfound knowledge into practice. Share your implementation with us or let us know if you encounter any hardships along the way. Together, we can conquer any coding challenge! 💪
Happy coding! 🚀