Extend Express Request object using Typescript
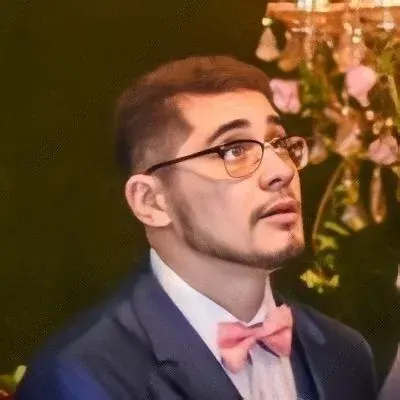
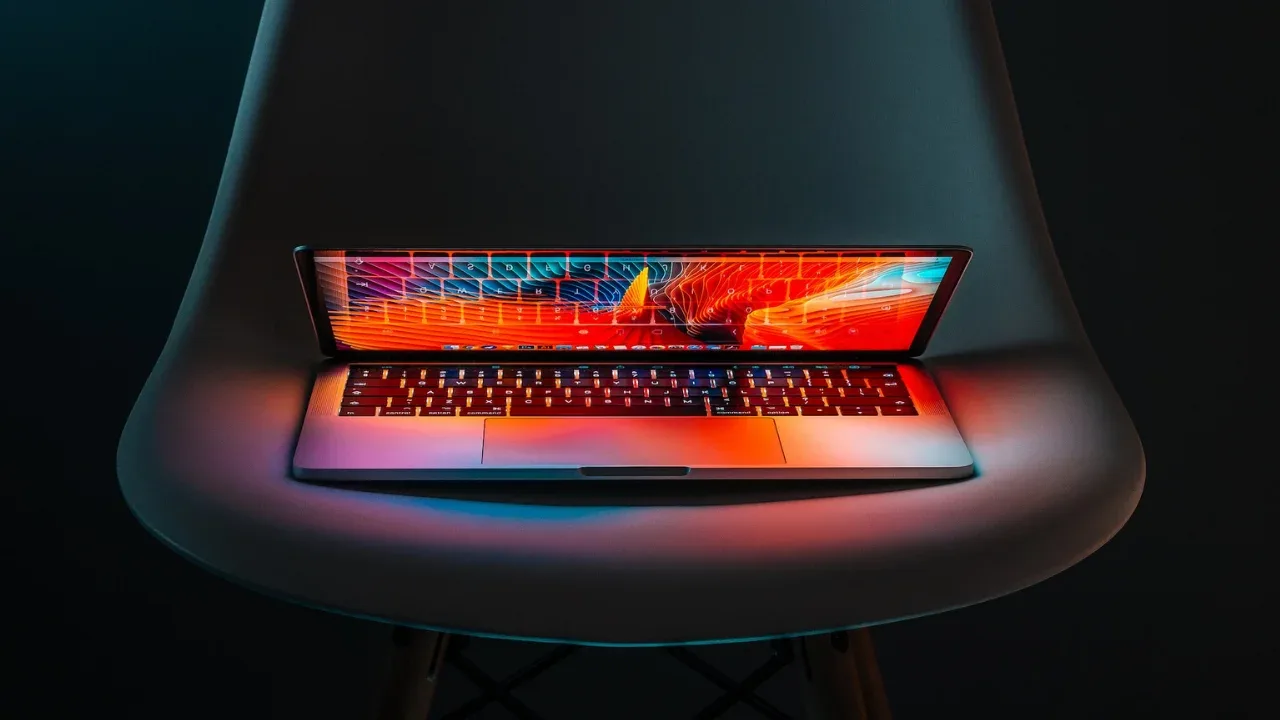
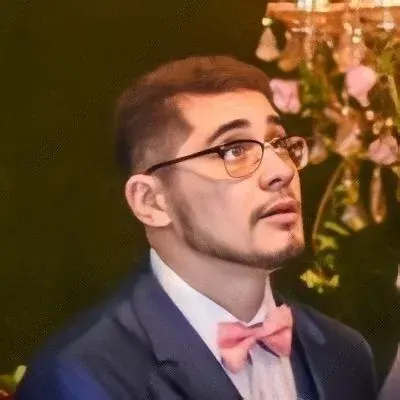
🌟 Extending Express Request Object using TypeScript
So, you want to add some extra properties to the Express request object in your TypeScript middleware, huh? 🤔 I feel you! It can be a bit tricky at first, but fear not! I'm here to guide you through this process and provide you with easy solutions. Let's get started! 💪
The Problem: Adding Extra Properties to the Request Object
From what I understand, you're looking for a way to add properties to the request object without using bracket notation. You'd prefer a cleaner and more TypeScript-friendly solution, right? Luckily, I've got a couple of approaches for you! 🙌
Solution 1: Declaration Merging
One way to extend the Express request object is through TypeScript's declaration merging feature. This allows us to augment existing types with additional properties or methods. Here's how you can do it:
// Create a new file (e.g., express.d.ts) in your project's root directory
// and add the following code:
declare namespace Express {
interface Request {
property: YourDesiredType;
}
}
In the code above, we're augmenting the Request
interface from the express
module with a new property called property
. Make sure to replace YourDesiredType
with the actual type you want to assign to property
. This declaration merging technique will now enable you to safely access req.property
within your middleware. 🎉
Solution 2: Custom Middleware
If you'd rather not mess with declaration merging, you can achieve the same result through a custom middleware. This approach allows you to add the desired property to each request passing through the middleware stack, as shown in your example. Check it out:
app.use((req, res, next) => {
(req as any).property = setProperty();
next();
});
In this code snippet, we're explicitly casting req
to any
to avoid TypeScript's type checking. This approach is useful when you don't want to change the global typings but still need to add custom properties to the request object.
Time to Dive In!
Now that you have a couple of methods to extend the Express request object, go ahead and start exploring the possibilities! 🚀 Remember to choose the approach that best suits your project's needs, and don't hesitate to experiment a bit.
I hope this blog post has answered your question and provided you with a solid understanding of how to extend the Express request object using TypeScript. If you found this helpful, feel free to share it with others who might be facing the same issue. Sharing is caring! ❤️
If you've got any questions, suggestions, or additional tricks, drop them in the comments below. Let's engage in a conversation and help each other out! 😊📝
Keep coding, keep learning, and keep extending those objects, my friend! See you in the next blog post! ✌️