Express.js req.body undefined
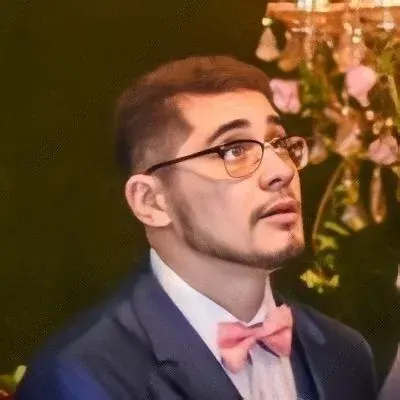
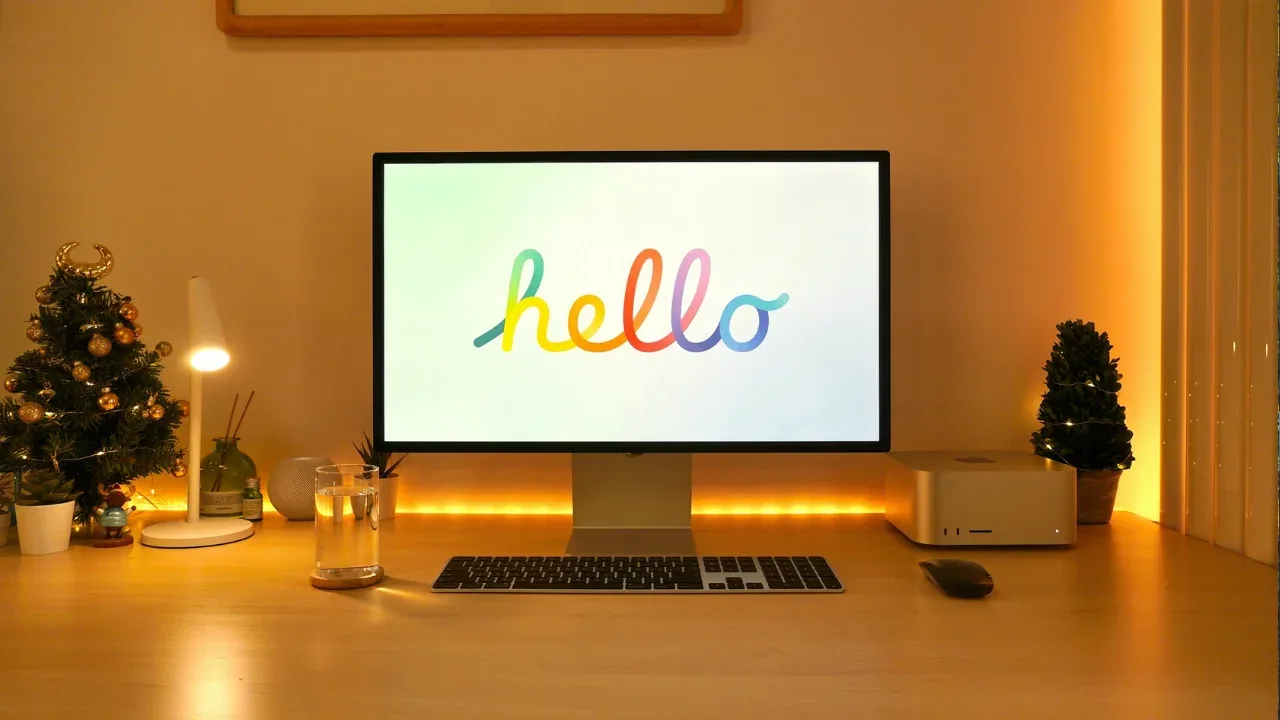
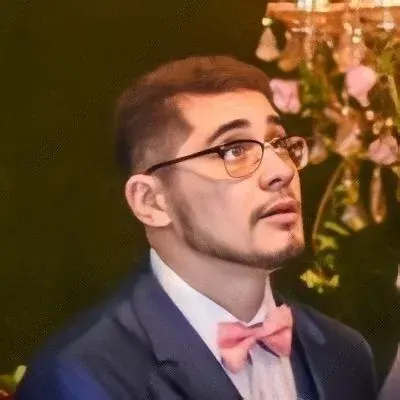
🚀 Understanding and Fixing "Express.js req.body undefined" Issue
So, you're running into some trouble with req.body
being undefined in your Express.js application? Don't worry, you're not alone! This is a common issue that many developers face. But fear not, in this guide, we'll walk you through the problem, explain what might be causing it, and provide easy solutions to get you back on track. Let's dive in! 💪
📝 Problem Overview
First, let's understand the problem. You mentioned that you have the following configuration for your Express server:
app.use(app.router);
app.use(express.cookieParser());
app.use(express.session({ secret: "keyboard cat" }));
app.set('view engine', 'ejs');
app.set("view options", { layout: true });
app.use(express.bodyParser());
app.use(express.methodOverride());
However, even with the express.bodyParser()
in place, when you try to access req.body.something
in your routes, you're getting an error that says body is undefined
. You also shared an example route that uses req.body
:
app.post('/admin', function(req, res){
console.log(req.body.name);
});
Now, let's find out what might be causing this issue and how to fix it.
🤔 Identifying the Cause
The most common reason for req.body
being undefined is the absence of the app.use(express.json())
and app.use(express.urlencoded({ extended: true }))
middleware in your application. Before Express version 4.x, you relied on the express.bodyParser()
middleware, which is a combined middleware for handling JSON and URL-encoded form data. However, starting from Express 4.x, it was deprecated, and you need to include the individual middleware for JSON and URL-encoded form data.
💡 Solution
To solve the problem, you need to make a small adjustment to your code. Replace the express.bodyParser()
middleware with the following:
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
These two middlewares will handle JSON and URL-encoded form data, respectively. By including them, you ensure that req.body
will be populated with the appropriate data.
Here's the updated configuration for your Express server:
app.use(app.router);
app.use(express.cookieParser());
app.use(express.session({ secret: "keyboard cat" }));
app.set('view engine', 'ejs');
app.set("view options", { layout: true });
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.use(express.methodOverride());
Give it a try, and you should see that the req.body.something
error is gone! 🎉
📣 Share Your Experience
We hope this guide helped you resolve the "Express.js req.body undefined" issue in your application. If you have any questions or faced a different problem, feel free to share it in the comments section below.
Share this post with your developer friends who might encounter a similar problem. Let's help each other learn and grow! 👥💻
Happy coding! 💙