Enabling HTTPS on express.js
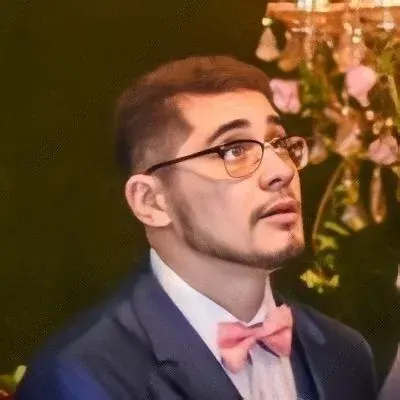
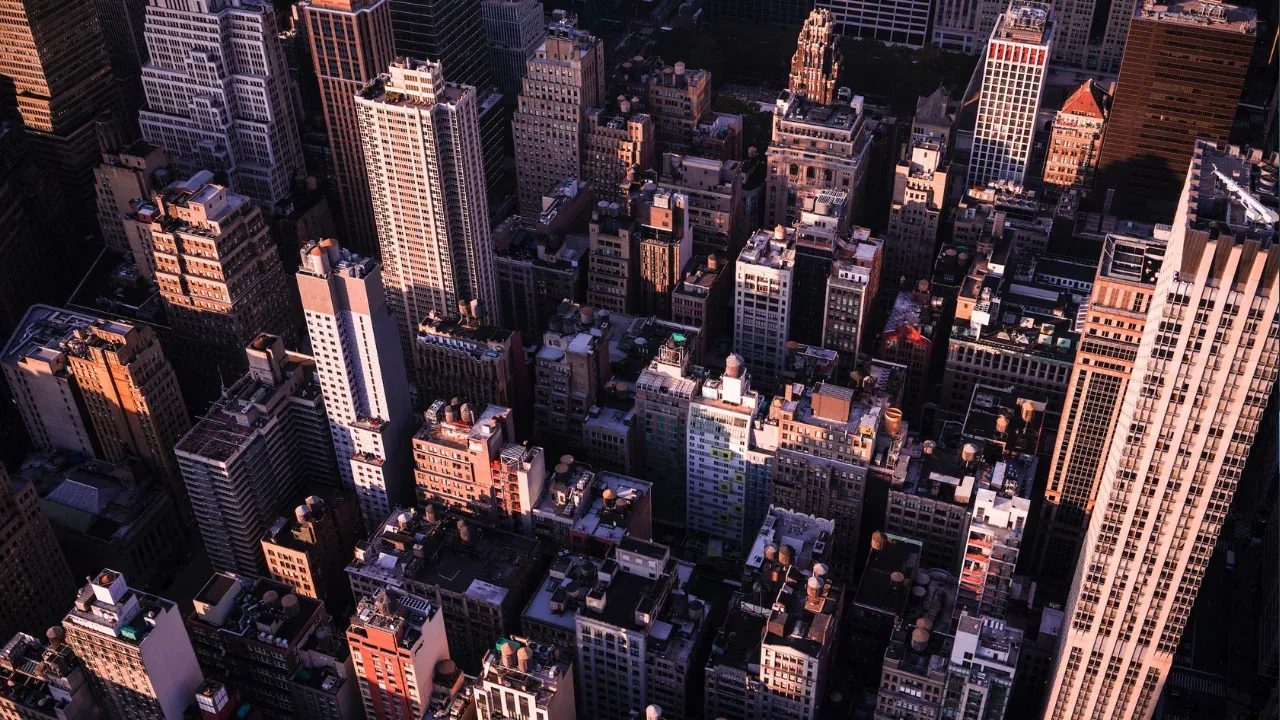
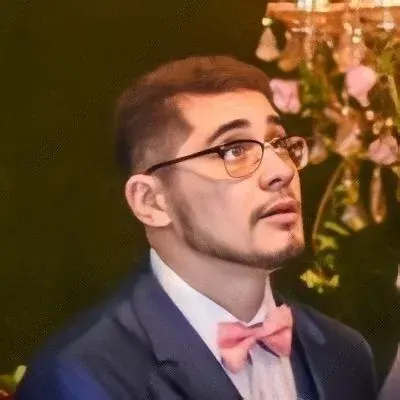
Enabling HTTPS on express.js: A Simple Guide 🚀
So you want to enable HTTPS on your express.js app? No worries, we've got you covered! In this guide, we'll walk you through the common issues and provide easy solutions to help you get your app up and running securely. Let's dive in!
The Problem 😓
Based on the context provided, it seems like you are having trouble getting your express.js app to respond to HTTPS requests. Your code only seems to work with HTTP requests. Don't worry, this can be easily fixed!
The Solution 💡
To enable HTTPS on your express.js app, you'll need to make a few modifications to your code. Let's go step by step:
First, make sure you have the necessary SSL certificate files (
server.key
andserver.crt
). If you don't have them, you can generate self-signed certificates or obtain valid ones from trusted certificate authorities.Next, update your
app.js
code as follows:
const express = require('express');
const https = require('https');
const fs = require('fs');
const privateKey = fs.readFileSync('sslcert/server.key', 'utf8');
const certificate = fs.readFileSync('sslcert/server.crt', 'utf8');
const credentials = { key: privateKey, cert: certificate };
const app = express();
app.get('/', (req, res) => {
res.send('hello');
});
const httpsServer = https.createServer(credentials, app);
httpsServer.listen(8000, () => {
console.log('HTTPS server listening on port 8000');
});
Save the changes and run the modified
app.js
file. Now, your express.js app should be able to respond to both HTTP and HTTPS requests.
That's it! 🎉 With these simple modifications, you have successfully enabled HTTPS on your express.js app.
Additional Considerations 🤔
While the above solution allows you to enable HTTPS on your express.js app, keep in mind that for production systems, it's often recommended to use a reverse proxy like Nginx or HAProxy to handle SSL termination.
Using a reverse proxy offers benefits such as improved performance, load balancing, and added security. You can configure the reverse proxy to handle SSL requests and communicate with your express.js app over regular HTTP.
AWS users can leverage EC2 Elastic Load Balancers (ELB) to handle SSL termination. By setting up your security group properly, you can ensure that only the ELB is allowed to send HTTP traffic to your EC2 instances, enhancing security.
Conclusion and Call-to-Action 🚀
Enabling HTTPS on your express.js app is essential for securing your web traffic. By following the simple steps outlined in this guide, you can easily ensure that your app responds to HTTPS requests.
So, why wait? Secure your express.js app today and provide a safe browsing experience for your users!
If you found this guide helpful, be sure to share it with your fellow developers and spread the knowledge. Happy coding! 😊