Declare multiple module.exports in Node.js
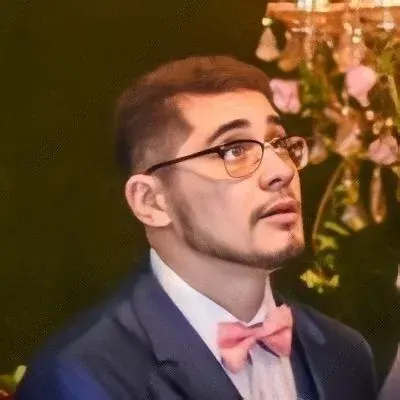
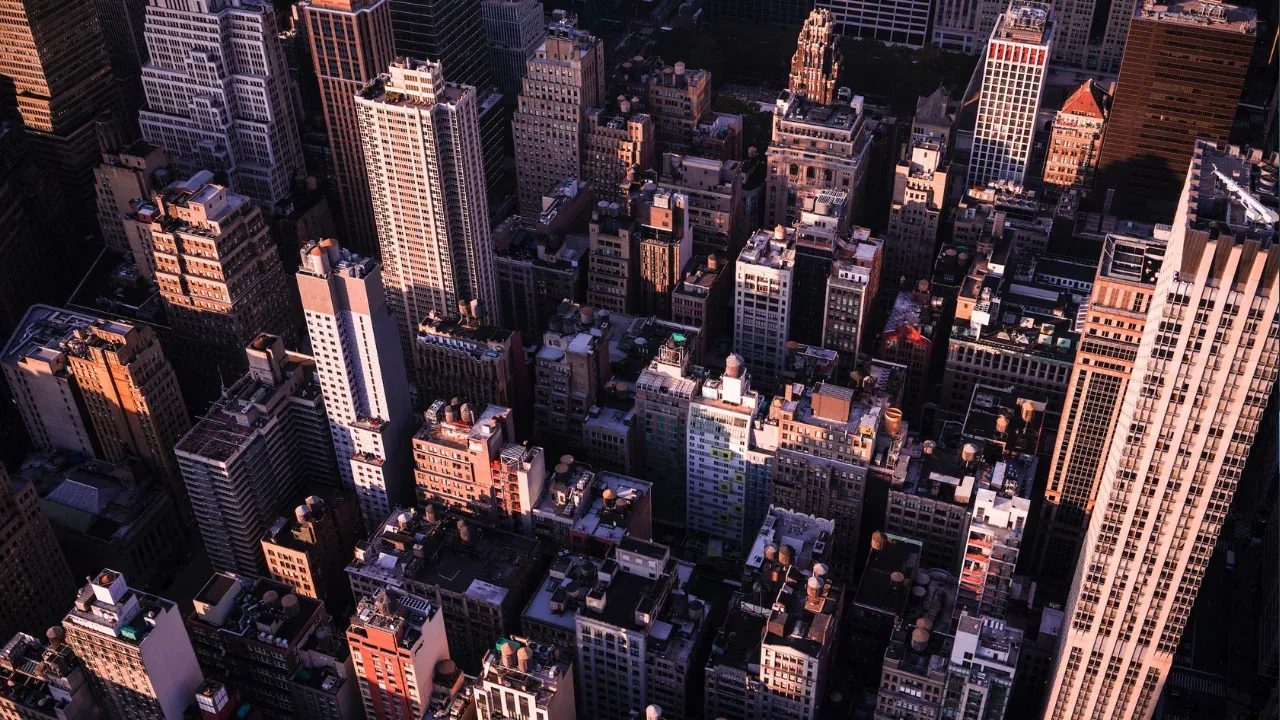
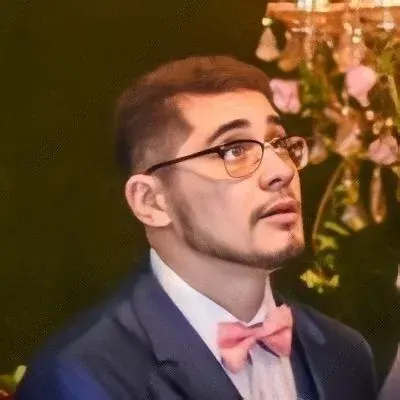
📚 Node.js: Declaring Multiple module.exports
So, you're trying to create a module in Node.js that contains multiple functions. But when attempting to use the module in your main file, you're encountering some issues with the parameter types. 🔧 Fear not, for we have the solutions you seek!
🤔 The Problem
The code snippet you shared tries to export two functions using multiple module.exports
. However, this approach won't work as expected because each subsequent module.exports
overwrites the previous one. 😱
Your code:
module.exports = function(firstParam) { console.log("You did it"); },
module.exports = function(secondParam) { console.log("Yes you did it"); }
This results in only the second function being exported, while the first one is lost. 😢
💡 The Solution
To overcome this challenge and export multiple functions from a single module, let's use an object to encapsulate these functions. This way, we can export the object containing all our functions.
Here's an updated version of your code:
module.exports = {
firstFunction: function(firstParam) { console.log("You did it"); },
secondFunction: function(secondParam) { console.log("Yes you did it"); }
};
With this revised code, we export an object with two functions: firstFunction
and secondFunction
. 🔁
🚀 Implementing the Solution
Now, let's see how you can use these exported functions in your main.js
file.
var moduleFunctions = require('./module.js');
var firstParam = {}; // Your object type
var secondParam = "https://example.com"; // Your URL string
moduleFunctions.firstFunction(firstParam);
moduleFunctions.secondFunction(secondParam);
In your main.js
, you import the module.js
file and assign it to a variable (moduleFunctions
). Then, you can call the functions using the dot notation on the moduleFunctions
object.
By doing this, you can pass any kind of parameter to your functions without worrying about type conflicts. 🎉
🎉 Level Up Your Code!
Congratulations! You've successfully learned how to declare and use multiple functions within a single module in Node.js. 🙌
Feel free to explore further possibilities with this approach. You can add more functions to the moduleFunctions
object, allowing you to organize and reuse code logic effectively.
💬 Join the Conversation
We would love to hear your thoughts about this topic and any alternative solutions you may have. Have you encountered any other challenges while working with Node.js modules? Let us know in the comments below! 👇
Happy coding! 💻