db.collection is not a function when using MongoClient v3.0
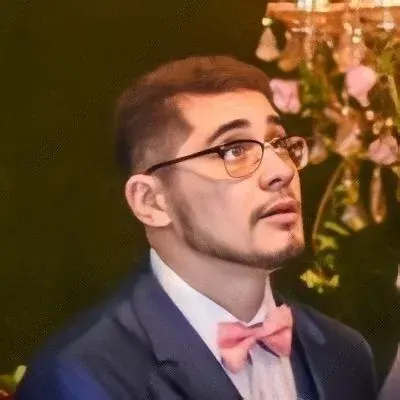
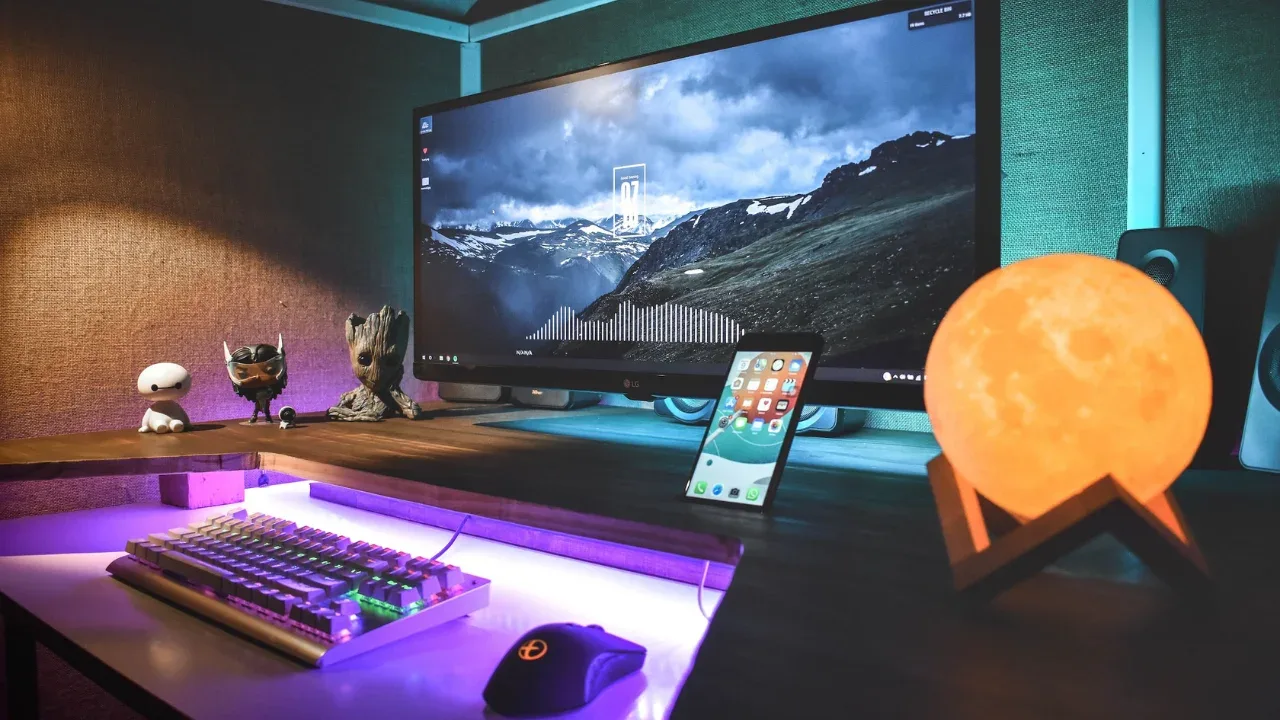
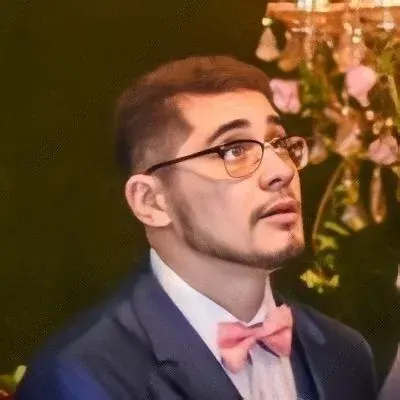
👋 Hey there! Have you faced the "db.collection is not a function" error while using MongoClient v3.0 in your Node.js application? Don't worry, you're not alone! This error can be a bit confusing, but I'm here to help you understand what's happening and how to fix it. Let's dive in! 🏊♂️
Understanding the Problem 🤔
The error you encountered is a TypeError, indicating that the db.collection
function is not recognized. This often occurs when there's a mismatch between the version of the MongoDB driver and the syntax used in your code.
Easy Solutions 💡
1. Check the MongoDB Driver Version 🖥️
As you mentioned, you have installed the MongoDB Node.js driver v3.0.0-rc0. Although it's the latest version of the driver, it might have some compatibility issues. To resolve this error, you have a couple of options:
Downgrade the MongoDB driver to a stable version that matches the tutorial you're following.
Update your code to use the syntax compatible with the v3.0.0-rc0 version of the driver.
2. Import MongoClient Correctly 📦
In the code snippet you shared, you imported MongoClient
using the following line:
var MongoClient = require('mongodb').MongoClient;
With the latest version of the MongoDB driver, this import might not work as expected. To fix it, try importing MongoClient
using the following syntax:
const { MongoClient } = require('mongodb');
3. Use the Connection Object 🤝
In some cases, the issue might be related to how you're establishing the connection with MongoDB. Instead of invoking db.collection()
directly on the db
object, try accessing it using the connection
object returned in the callback. Here's an example:
MongoClient.connect(url, function(err, client) {
if (err) throw err;
const db = client.db(); // Access the database object using client.db()
db.collection("customers").findOne({}, function(err, result) {
if (err) throw err;
console.log(result.name);
client.close();
});
});
By using client.db()
, you ensure that the db
object has access to the collection
function, resolving the error.
Wrapping Up 🎁
I hope these solutions help you resolve the "db.collection is not a function" error in your MongoClient v3.0 code. Remember, you can either match the MongoDB driver version to the tutorial you're following or update your code to be compatible with the latest driver version.
If you have any further questions or need additional help, feel free to ask. Happy coding! 🚀