Comparing mongoose _id and strings
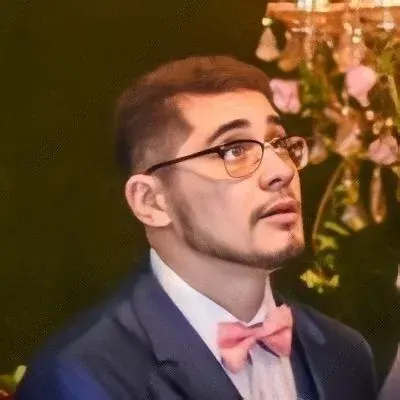
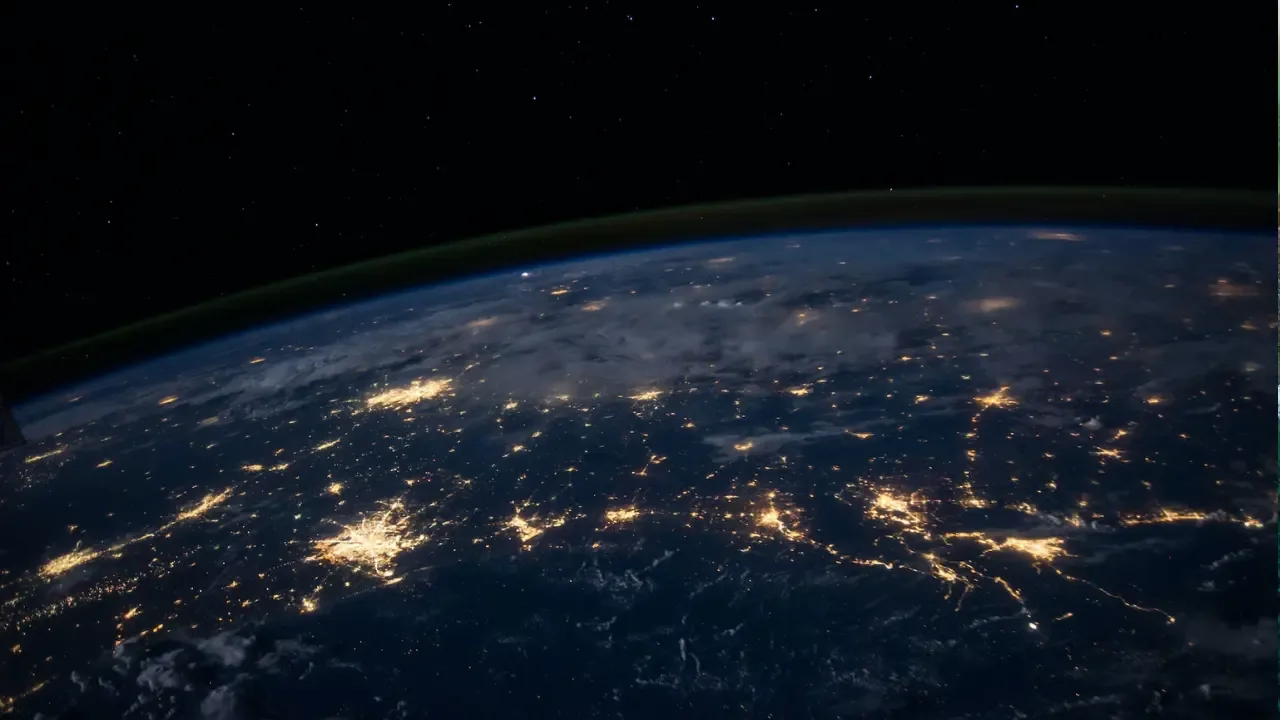
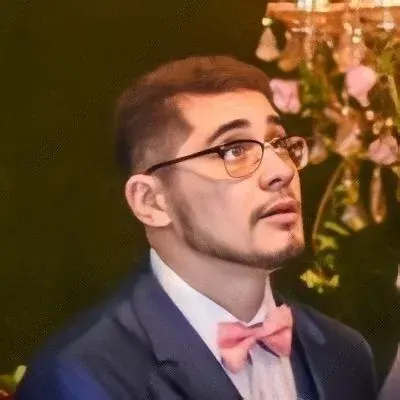
Comparing mongoose _id and strings: Understanding the Issue 👥💻
So you have a node.js application and you're facing a problem when comparing mongoose _id and strings. You're not alone - many developers have faced this issue before. But fear not, we're here to help you understand and solve this problem! 😎
The Problem: Mismatched Comparison 🤷♂️❗
In your code snippet, you're storing a user's _id from MongoDB into the results
object. Later, when you compare results.userId
with AnotherMongoDocument._id
, the comparison is never true. 😱
When you logged both ids, you noticed that they are an exact match. So what's the problem? 🤔
Understanding mongoose _id and Strings 🧐
Mongoose _id values are actually objects, specifically instances of the ObjectId
class. On the other hand, when you retrieve data from MongoDB, the _id is converted to a string. This can lead to a mismatch when comparing the two. 🔄
In JavaScript, the ==
operator compares values by their content while ignoring their data types. This means that even if the content (the string representation of the _id) is the same, the comparison will still return false if the data types don't match.🚫✅
The Solution: Converting the data types ✅🔄
To solve this problem, you need to ensure that both operands of the comparison have the same data type. Since the _id in AnotherMongoDocument
is a string, you need to convert results.userId
to a string as well. 🎉
Here's how you can do it:
if (results.userId.toString() === AnotherMongoDocument._id.toString()) {
console.log('This is true now!');
}
By using the toString()
method on both _ids, you convert them to strings and ensure a proper comparison between them. Now your if/then statement will work as expected! 😄
One More Thing: Consider Using '===' 🙌
While ==
works for this specific case, it's generally a good practice to use the strict equality operator ===
in JavaScript. The ===
operator not only compares the values but also checks whether their data types match. This can help you catch potential errors and ensure more accurate comparisons. 💪
Share Your Experience! 📣
Have you encountered this issue before? How did you solve it? We'd love to hear your experiences and solutions in the comments below! Let's help each other out. 🤝🗯
Remember, understanding the problem and finding a solution is what makes us better developers. Keep coding, stay curious, and never stop learning! 💻🚀
Stay connected with us for more exciting tech tips and tricks! Follow us on Twitter and Facebook. Don't forget to subscribe to our newsletter for regular updates! 📩
Happy coding! 😄💻✨