Avoid "current URL string parser is deprecated" warning by setting useNewUrlParser to true
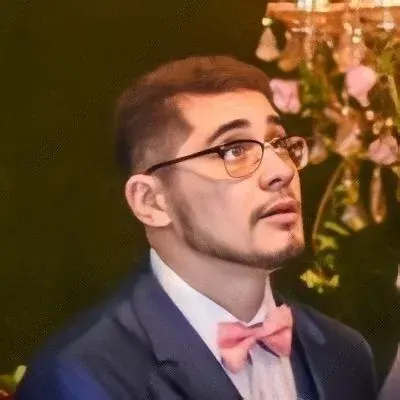
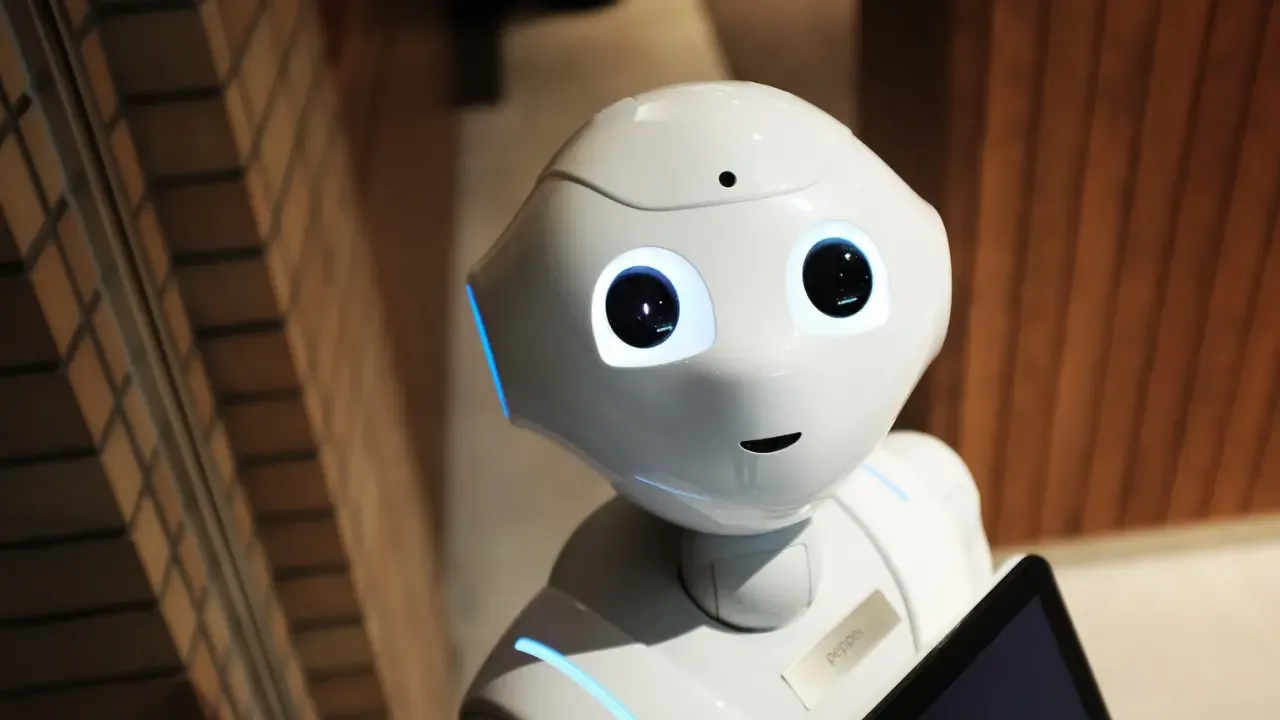
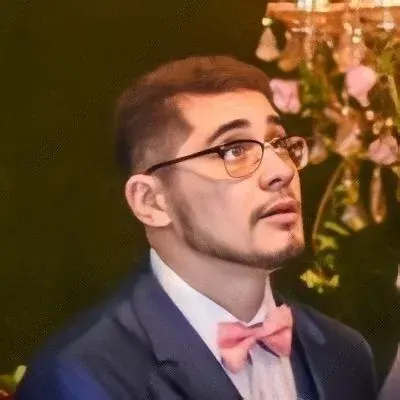
Avoiding the "current URL string parser is deprecated" warning: A Simple Solution
If you're using the mongodb
driver, you might have come across the warning message:
š (node:4833) DeprecationWarning: current URL string parser is deprecated, and will be removed in a future version. To use the new parser, pass option { useNewUrlParser: true } to MongoClient.connect.
Don't worry! We've got you covered with an easy solution to remove this warning.
The Problem
Let's take a look at the code causing the warning:
async connect(connectionString: string): Promise<void> {
this.client = await MongoClient.connect(connectionString)
this.db = this.client.db()
}
When establishing a connection to a MongoDB instance, calling MongoClient.connect()
triggers the deprecation warning.
Trying the Obvious Solution
You might have tried adding the useNewUrlParser
property to the MongoClientOptions
instance as the second argument, like this:
async connect(connectionString: string): Promise<void> {
this.client = await MongoClient.connect(connectionString, { useNewUrlParser: true })
this.db = this.client.db()
}
But, as you might have noticed, this doesn't work. There's no useNewUrlParser
property available in MongoClientOptions
.
Another Failed Attempt
You may have also attempted to set the useNewUrlParser
property in the connection string itself, like this:
connectionString = "mongodb://127.0.0.1/my-db?useNewUrlParser=true"
But, unfortunately, this approach has no effect on the warning.
The Solution: Downgrading your MongoDB driver
To remove the deprecation warning, one straightforward solution is to use an older version of the mongodb
driver and its corresponding @types/mongodb
package.
In your package.json
, update the version of "mongodb"
and "@types/mongodb"
to:
"mongodb": "~3.0.8",
"@types/mongodb": "~3.0.18"
Why Downgrade?
The warning message is triggered by the latest version of the mongodb
driver, specifically versions 3.1.0-beta4 and above. By using the recommended lower versions mentioned above, you can avoid the warning altogether.
The Benefits of Downgrading
Making this change will not only remove the deprecation warning but also prevent it from affecting other parts of your code. Additionally, you won't receive unwanted spam emails caused by these warnings when running the script as a cron job.
Conclusion
Removing the "current URL string parser is deprecated" warning is as simple as downgrading your mongodb
driver to version 3.0.8 and its corresponding @types/mongodb
package to version 3.0.18. This ensures a smooth and error-free operation of your codebase.
Feel free to leave a comment below if you have any questions or other solutions to this issue. Let's keep our code clean and updated! š»šŖ