MySQL with Node.js
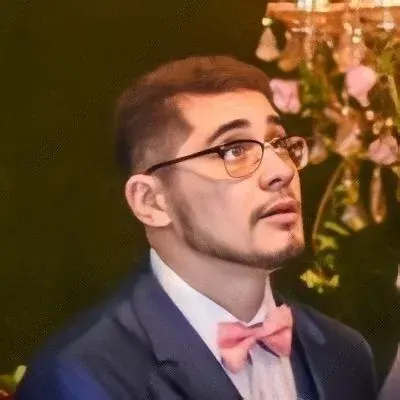
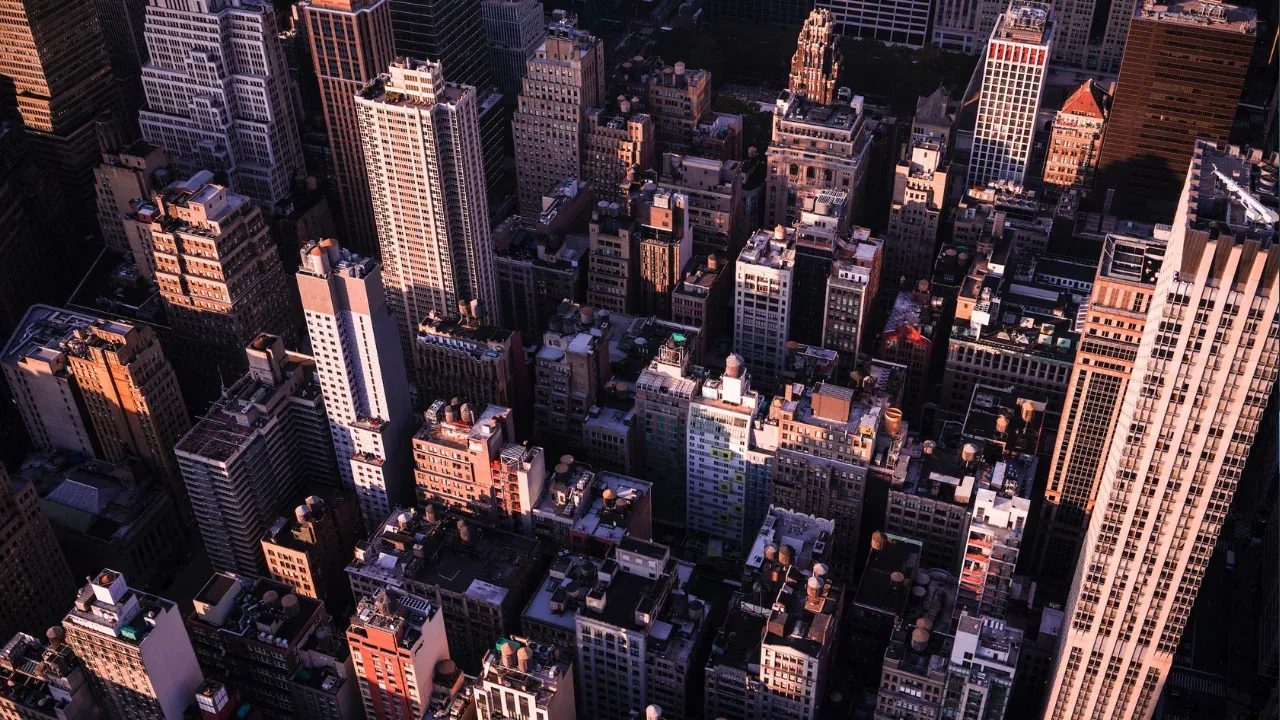
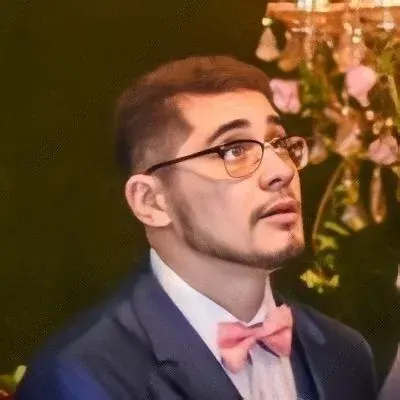
📝 How to Use MySQL with Node.js: A Guide for Web Developers 💻
If you're a web developer, chances are you're familiar with MySQL, a powerful open-source relational database management system. However, if you've recently started diving into Node.js, you might be wondering how to integrate MySQL into your new tech stack. 🤔
⚡️ Why Use MySQL with Node.js?
Using MySQL with Node.js offers several advantages. Firstly, MySQL is a mature and widely adopted database system known for its stability and performance. Secondly, Node.js, with its non-blocking and event-driven architecture, is an ideal match for building scalable and high-performing web applications. By using MySQL in conjunction with Node.js, you can tap into the power of both technologies, effectively managing your data while providing a seamless user experience. 🚀
🛠 Setting Up MySQL Connection in Node.js
Before diving into using MySQL with Node.js, make sure you have both MySQL and Node.js installed on your machine. Once that's done, follow these steps to establish a connection:
Install the
mysql
package: Usenpm
to install the MySQL package by running the following command in your terminal:
npm install mysql
Require the
mysql
module: In your Node.js file, require themysql
module to gain access to its functions and methods:
const mysql = require('mysql');
Create the connection: Next, create a connection by configuring the MySQL options and invoking the
createConnection
method:
const connection = mysql.createConnection({
host: 'localhost',
user: 'your_mysql_username',
password: 'your_mysql_password',
database: 'your_database_name'
});
Replace your_mysql_username
, your_mysql_password
, and your_database_name
with your actual MySQL credentials and the name of the database you want to connect to.
4. Establish the connection: Finally, establish the connection by calling connect()
:
connection.connect((error) => {
if (error) {
console.error('Error connecting to MySQL:', error);
} else {
console.log('Connected to MySQL!');
}
});
⚠️ Common Issues and Easy Solutions
While working with MySQL and Node.js, you might encounter some common issues. Here are a few solutions to help you overcome them:
Escaping queries: To prevent SQL injection attacks and handle special characters properly, use the
mysql.escape()
function to escape dynamic values in your SQL queries. For example:
const userId = 1;
const query = `SELECT * FROM users WHERE id = ${mysql.escape(userId)}`;
Handling asynchronous nature: Node.js is asynchronous, meaning that operations may not complete in the order you expect. To handle this, use promises, callbacks, or async/await syntax to ensure your database operations are executed and controlled correctly.
Pooling connections: Creating a new database connection for every request can be resource-intensive. Instead, consider implementing connection pooling, which allows you to reuse existing connections, improving performance. The
mysql
package provides acreatePool
method for this purpose.
Remember, these solutions are just the tip of the iceberg. 🧊 Dive deeper into MySQL's documentation and explore Node.js libraries to leverage even more powerful tools and techniques.
📢 Join the Conversation!
Using MySQL with Node.js opens up a world of possibilities for web developers. Have you encountered any other MySQL-related challenges while working with Node.js? Found any other handy tricks or libraries? Share your experiences, tips, and questions in the comments below! Let's keep the conversation flowing. 🗣️💬
And don't forget to share this post with your fellow developers who are seeking guidance on combining MySQL with Node.js. Sharing is caring! 🤝✨