MySQL: Insert record if not exists in table
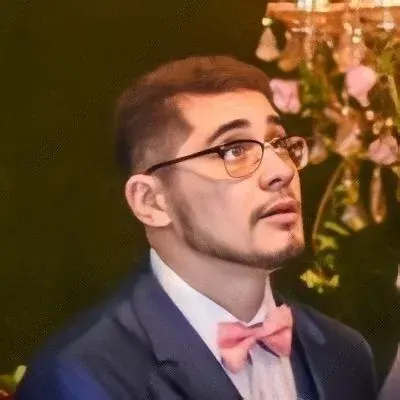
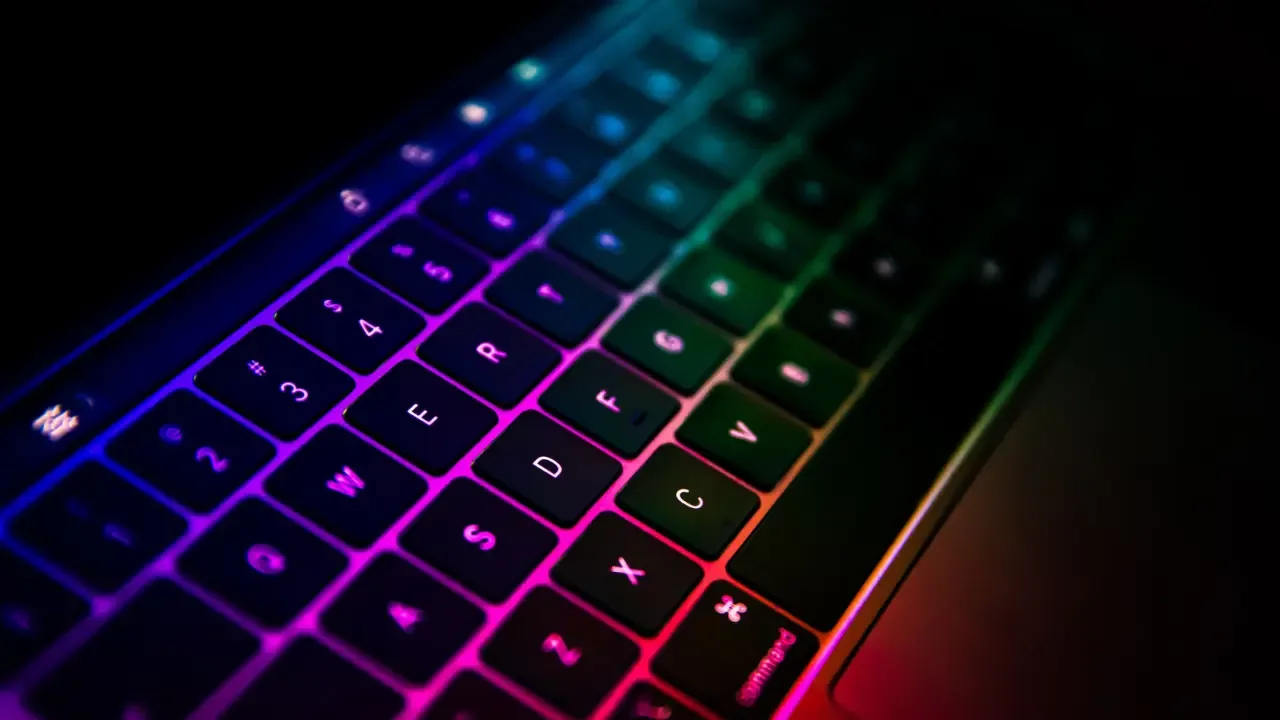
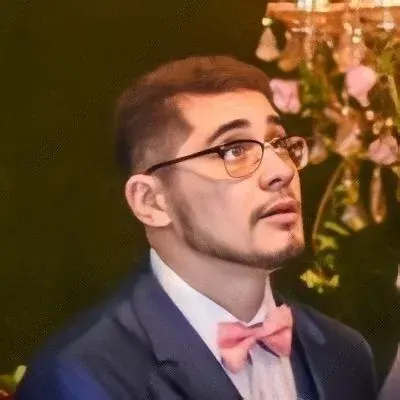
How to insert a record in MySQL only if it does not already exist
Are you trying to insert a record into a MySQL table only if a certain value does not already exist in the table? This can be a common scenario when you want to avoid duplicate entries in your database. In this blog post, we'll address this issue and provide you with easy solutions to achieve your goal.
The problem: duplicates in the MySQL table
Let's first understand the problem you are facing. You have a MySQL table called table_listnames
with columns like name
, address
, and tele
. When you execute the following query:
INSERT INTO table_listnames (name, address, tele)
VALUES ('Rupert', 'Somewhere', '022')
WHERE NOT EXISTS (
SELECT name FROM table_listnames WHERE name='value'
);
you encounter an error. The purpose of this query is to insert a new record into the table_listnames
table, but only if the name
field does not already exist. However, this approach is not working as expected.
Solution 1: Use INSERT IGNORE
One easy solution to avoid duplicates is to use the INSERT IGNORE
statement. The INSERT IGNORE
statement inserts a record into the table and ignores the entire row if a duplicate key error occurs. Here's how you can modify your query:
INSERT IGNORE INTO table_listnames (name, address, tele)
VALUES ('Rupert', 'Somewhere', '022');
If the name
field already exists in the table, this query will not cause an error and simply skip the insertion.
Solution 2: Use a unique constraint
Another effective way to handle this scenario is by adding a unique constraint to the name
column. A unique constraint ensures that the values in the column are always unique, preventing duplicate entries. You can alter your table schema with the following command:
ALTER TABLE table_listnames
ADD CONSTRAINT unique_name
UNIQUE (name);
Once the unique constraint is in place, you can use a simple INSERT
statement without any additional checks:
INSERT INTO table_listnames (name, address, tele)
VALUES ('Rupert', 'Somewhere', '022');
If the name
field already exists, MySQL will throw a duplicate entry error, and you can handle it according to your application's requirements.
Solution 3: Use a combination of SELECT and INSERT
Yet another way to achieve the desired result is by using a combination of a SELECT
statement and an INSERT
statement. This approach allows you to first check if the value exists, and then conditionally insert the record. Here's an example:
INSERT INTO table_listnames (name, address, tele)
SELECT 'Rupert', 'Somewhere', '022'
FROM dual
WHERE NOT EXISTS (
SELECT name FROM table_listnames WHERE name='Rupert'
);
The SELECT 'Rupert', 'Somewhere', '022' FROM dual
part acts as a dummy table, and the WHERE NOT EXISTS
clause ensures that the record is only inserted if the name
field does not already exist.
Conclusion: Choose the right approach for your needs
Congratulations! You now have multiple solutions to insert a record into a MySQL table only if it does not already exist. Whether you opt for the INSERT IGNORE
statement, add a unique constraint, or use a combination of SELECT
and INSERT
, make sure to choose the approach that best suits your specific requirements.
If you found this blog post helpful, don't forget to share it with your friends and colleagues who might also be struggling with this MySQL problem. And if you have any questions or alternative solutions, feel free to leave a comment below. Happy coding! 😊👍