How to select the nth row in a SQL database table?
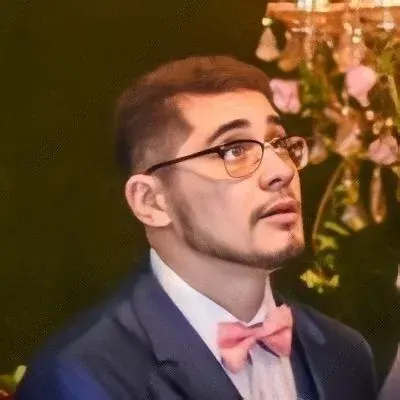
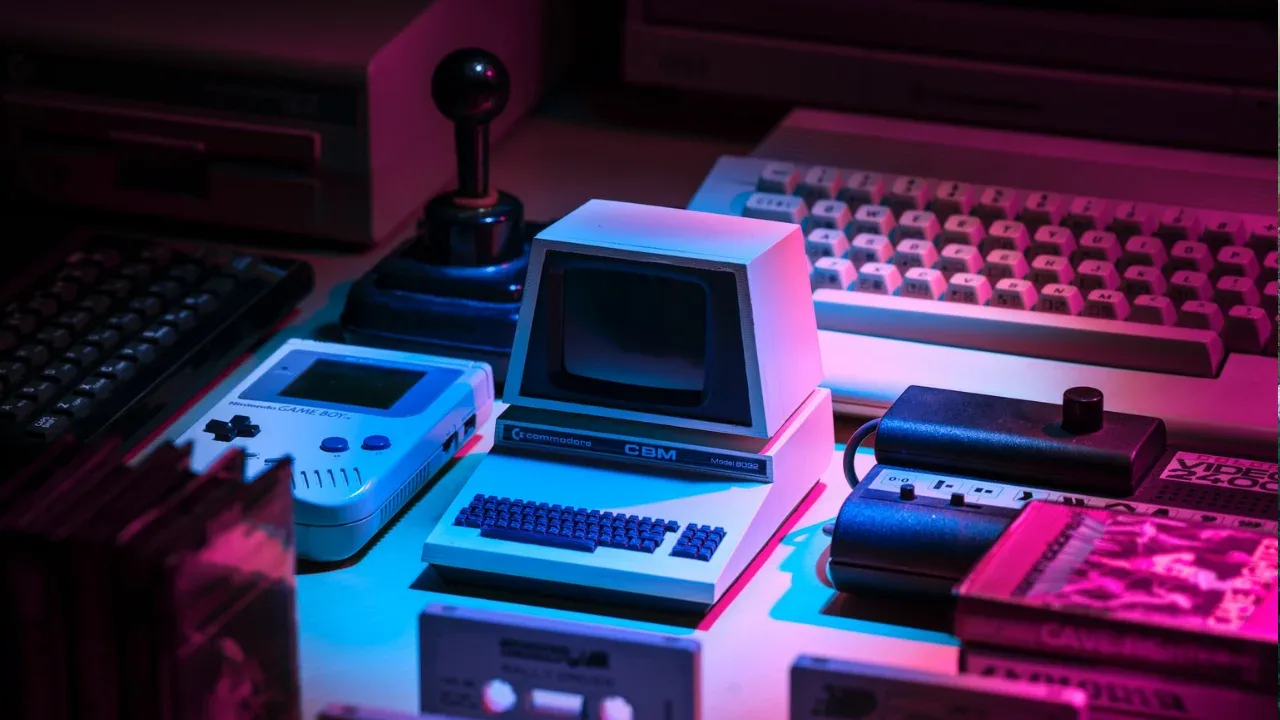
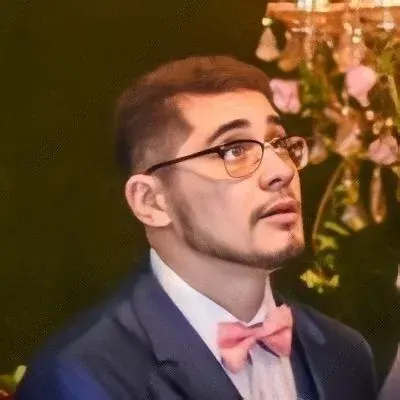
How to Select the nth Row in a SQL Database Table?
Do you ever find yourself in a situation where you need to select a specific row from a SQL database table? Maybe you want to retrieve the 100th customer's information or fetch the 5th highest salary in your employee table. In this blog post, we will explore the most effective and database-agnostic ways to accomplish this task. We will also dive into how you can achieve the same result using the native functionality of popular databases like SQL Server, MySQL, PostgreSQL, SQLite, and Oracle.
The SQL Server Approach
In SQL Server 2005 and above, you can utilize the ROW_NUMBER()
function to assign a unique number to each row based on a specific order. Then, you can simply filter the result by the desired row number. Here's an example:
WITH Ordered AS (
SELECT ROW_NUMBER() OVER (ORDER BY OrderID) AS RowNumber, OrderID, OrderDate
FROM Orders)
SELECT *
FROM Ordered
WHERE RowNumber = 1000000
The ROW_NUMBER()
function helps us achieve the desired result by ordering the rows by the OrderID
and assigning a unique RowNumber
to each row. Finally, we filter the result to retrieve the row with RowNumber
equal to 1000000.
Database-Agnostic Approach
If you want a solution that works across different databases, we can leverage the LIMIT
and OFFSET
clauses. Here's an example:
SELECT *
FROM Orders
ORDER BY OrderID
LIMIT 1 OFFSET 999999
In this example, we start by ordering the rows by OrderID
and then use the LIMIT
clause to specify that we only want one row. By adding the OFFSET
clause with a value of 999999, we skip the first 999,999 rows and retrieve the desired row.
Native Functionality in Other Databases
Now, let's take a look at how you can achieve the same result using the native functionality of other popular databases.
MySQL
SELECT *
FROM Orders
ORDER BY OrderID
LIMIT 999999, 1
PostgreSQL
SELECT *
FROM Orders
ORDER BY OrderID
LIMIT 1 OFFSET 999999
SQLite
SELECT *
FROM Orders
ORDER BY OrderID
LIMIT 1 OFFSET 999999
Oracle
SELECT *
FROM (
SELECT OrderID, OrderDate
FROM Orders
ORDER BY OrderID
)
WHERE ROWNUM = 1000000
In Conclusion
Selecting the nth row in a SQL database table might seem like a complex task, but with the right approach, it can be simplified. In this blog post, we explored both the database-specific and database-agnostic solutions to this problem. Whether you're using SQL Server, MySQL, PostgreSQL, SQLite, or Oracle, you now have the knowledge to retrieve the desired rows effortlessly.
Have you ever encountered a situation where you needed to select a specific row? How did you solve it? Let us know in the comments below!