Get the new record primary key ID from MySQL insert query?
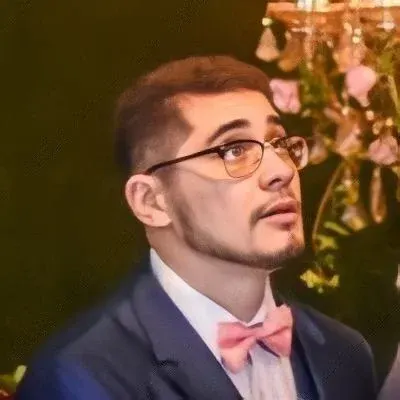
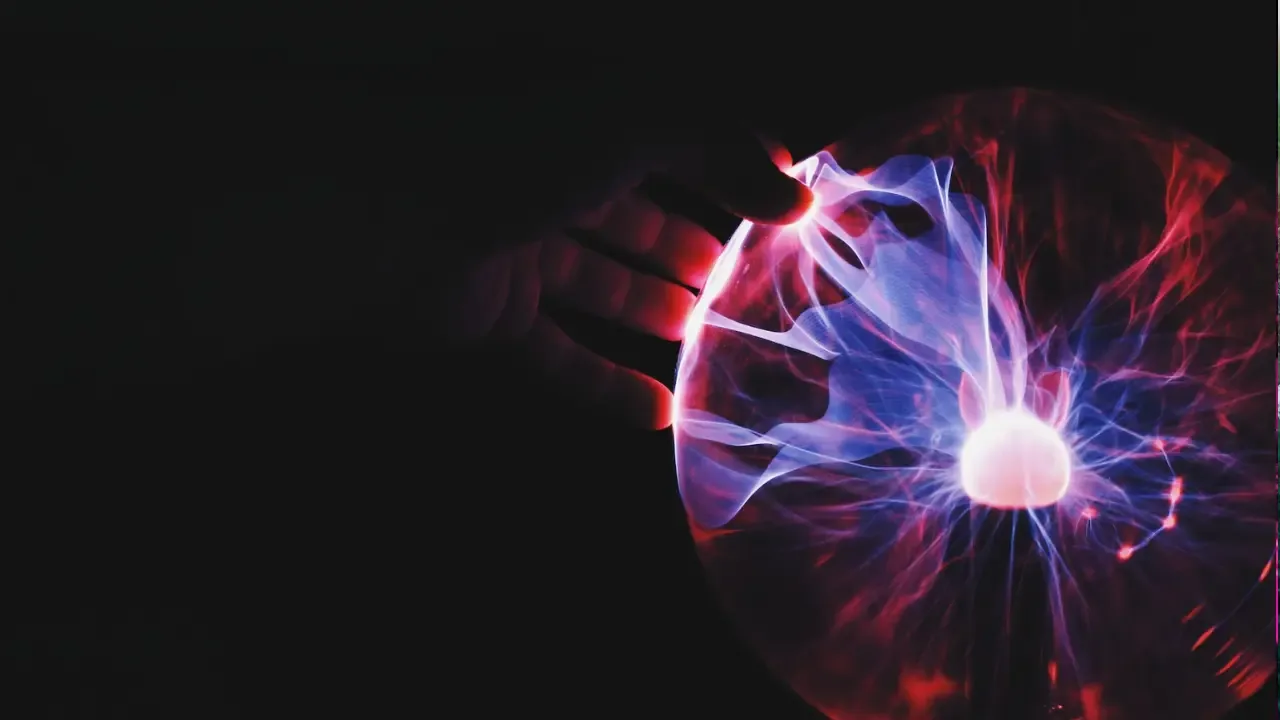
šš Getting the new record primary key ID from a MySQL insert query can be a tricky task, but fear not! In this blog post, we will address this common issue and provide you with easy solutions. So, let's dive in and find out how to tackle this problem!
ā”ļø The Problem: You have a table in MySQL with an auto-incrementing primary key column. You want to insert a new record into this table and, at the same time, retrieve the value of the newly generated primary key. Running a second query to retrieve the ID seems inefficient and prone to errors. What is the best practice to solve this problem?
š” The Solution: Fortunately, MySQL provides a way to retrieve the auto-generated primary key value directly within the query itself. Let's take a look at how to achieve this in a few simple steps:
Use the
LAST_INSERT_ID()
function:After executing the
INSERT
statement, simply call theLAST_INSERT_ID()
function to obtain the last generated primary key value.The function returns the value generated by the last statement that inserted an auto-incremented primary key.
Incorporate the function into your INSERT query:
Modify your
INSERT
statement to include theLAST_INSERT_ID()
function.Here's an example of how you can structure your query:
INSERT INTO your_table (column1, column2, ...) VALUES (value1, value2, ...); SELECT LAST_INSERT_ID();
Replace
your_table
,column1
,column2
, and so on with the appropriate table and column names.Replace
value1
,value2
, and so on with the values you want to insert.The
SELECT LAST_INSERT_ID()
statement will return the newly generated primary key value in the result set.
Retrieve the primary key value in your code:
To access the primary key value obtained from the query, fetch it from the result set using your programming language of choice.
The exact method will depend on the programming language and database abstraction layer you are using, but it typically involves retrieving the first row and column value from the result set.
š Example Code (Using PHP and PDO): Here's an example of how you can retrieve the primary key value using PHP and PDO:
$insertQuery = "INSERT INTO your_table (column1, column2, ...)
VALUES (:value1, :value2, ...);
SELECT LAST_INSERT_ID();";
$statement = $pdo->prepare($insertQuery);
$statement->bindValue(':value1', $value1);
$statement->bindValue(':value2', $value2);
// Bind other values accordingly if needed
$statement->execute();
$primaryKey = $statement->fetchColumn();
echo "The new primary key is: " . $primaryKey;
š£ Call-to-Action: Now that you know how to retrieve the new record primary key ID from a MySQL insert query, it's time to put this knowledge into practice. Experiment with it in your projects, and if you encounter any issues or have suggestions, let us know in the comments below!
š Remember, sharing is caring! If you found this blog post useful, don't hesitate to share it with your fellow developers and spread the knowledge.
Happy coding! š»š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
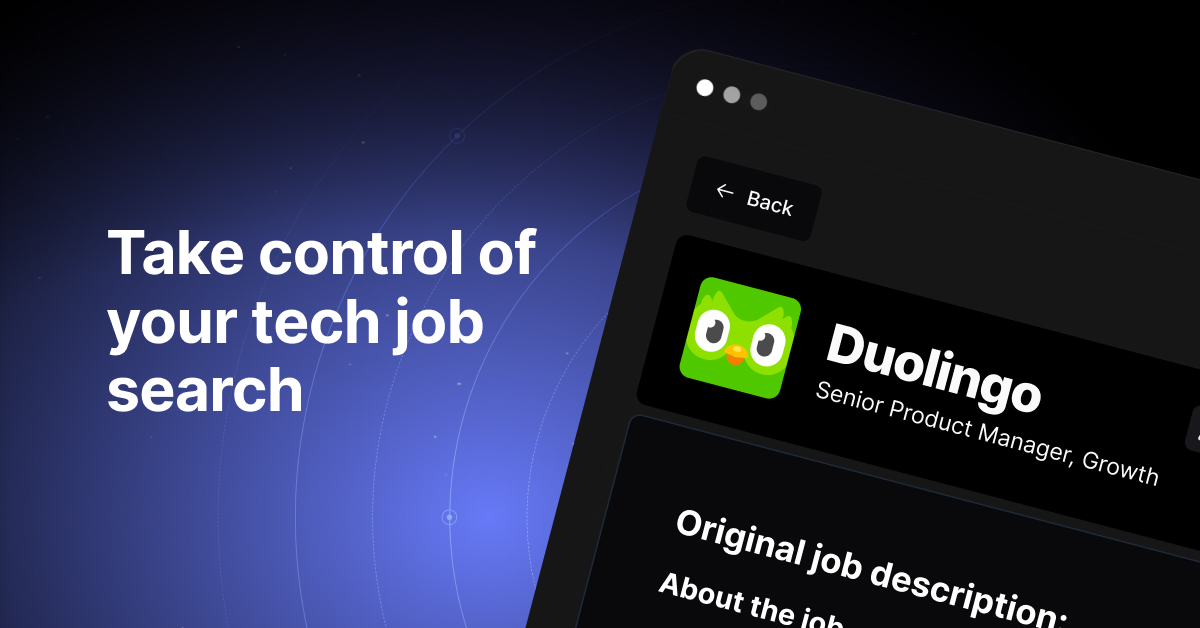