Remove by _id in MongoDB console
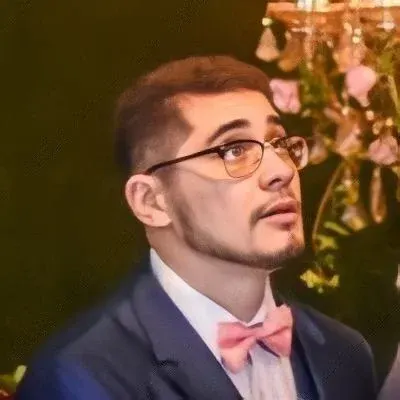
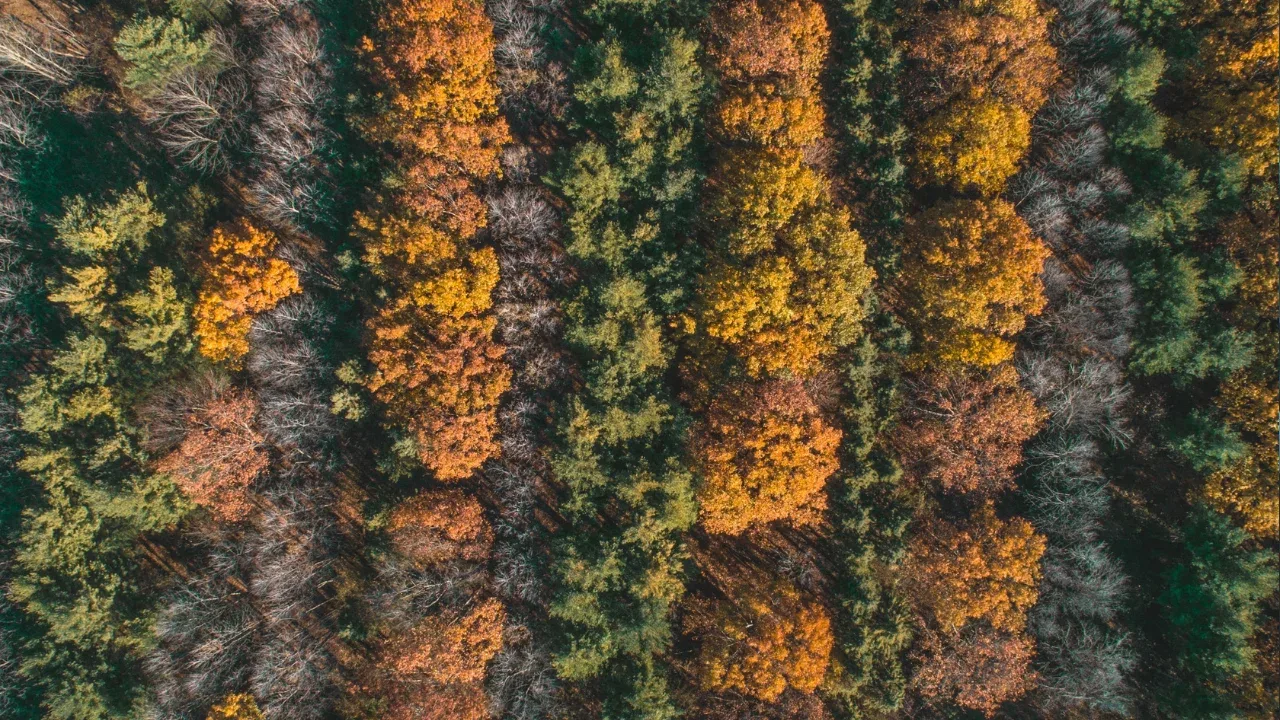
Removing a Record by _id in MongoDB Console
So, you want to remove a record by _id
in the MongoDB console? No worries, I've got your back! It's not as complicated as it seems. Let's dive right into it!
The Problem
In the provided context, the user wanted to remove a record by _id
in the MongoDB console. However, the commands they tried didn't work. 🤔
The Solution
To remove a record by _id
in MongoDB, you need to use the deleteOne()
or deleteMany()
method. Here's how you can do it:
db.collectionName.deleteOne({ "_id" : ObjectId("your_id_here") })
or
db.collectionName.deleteMany({ "_id" : ObjectId("your_id_here") })
Let's break down this command:
db.collectionName
refers to the name of the collection where you want to remove the record.deleteOne()
is used to delete a single record, whiledeleteMany()
is used to delete multiple records.{ "_id" : ObjectId("your_id_here") }
specifies the filter criteria to identify the record you want to remove. Replace"your_id_here"
with the actual_id
you want to target.
Applying the Solution
In the given scenario, let's assume we have a collection called test_users
, and we want to remove the record with _id
equal to "4d512b45cc9374271b02ec4f"
. Here's the correct command to achieve that:
db.test_users.deleteOne({ "_id": ObjectId("4d512b45cc9374271b02ec4f") })
Remember 🧠
Make sure to import the ObjectId
class from MongoDB in the console before using the deleteOne()
or deleteMany()
methods. If you haven't imported it, you might encounter errors.
use your_database_name // Switch to your desired database
ObjectId = require('mongodb').ObjectID // Import the ObjectId class
Conclusion and a Call-to-Action
Removing a record by _id
in the MongoDB console is not as intimidating as it might seem at first. Remember to use the proper deleteOne()
or deleteMany()
method and provide the correct _id
value in the filter criteria.
If you found this guide helpful, share it with others who might be struggling with the same issue. Also, feel free to drop any other MongoDB-related questions in the comments section below. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
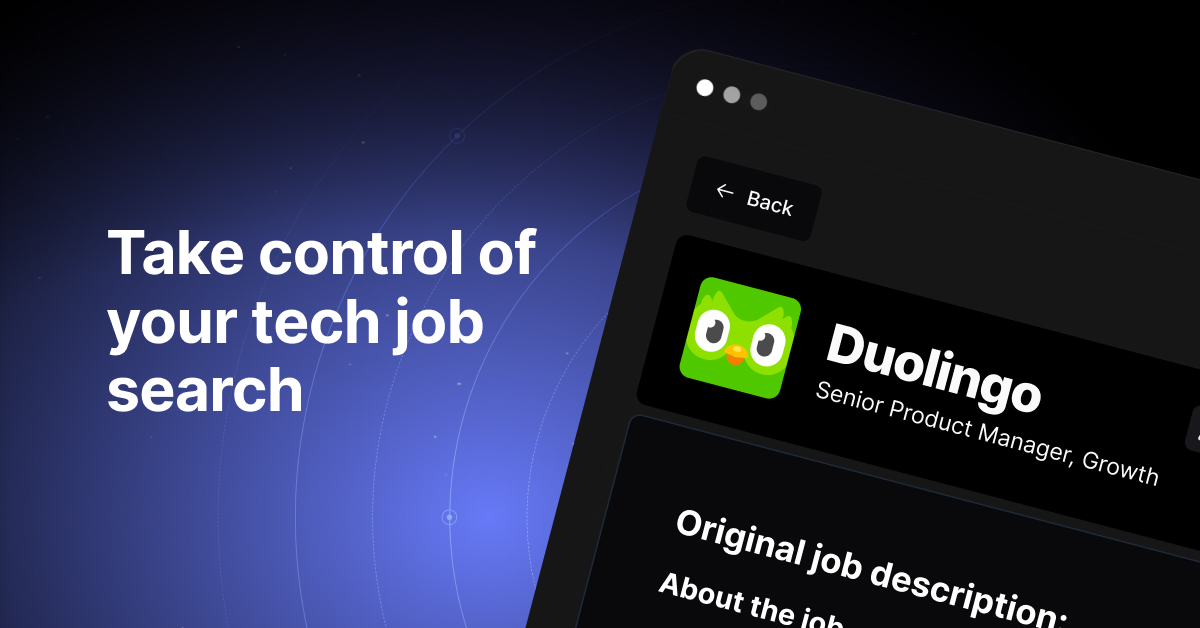