Performing regex queries with PyMongo
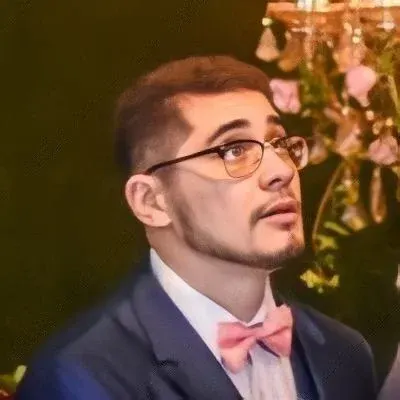
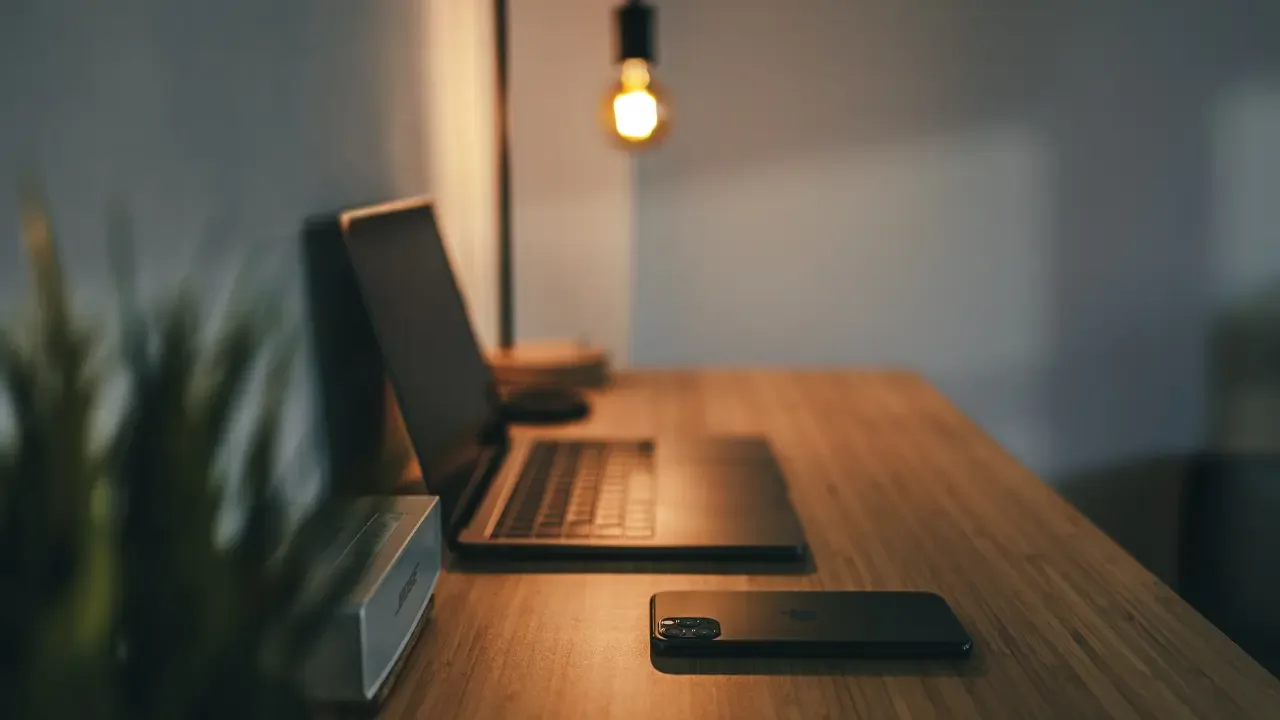
Performing regex queries with PyMongo: A Complete Guide 👨💻
Are you trying to perform a regex query using PyMongo against a MongoDB server but getting empty results? Don't worry, you're not alone! Many developers face difficulties when it comes to performing regex queries with PyMongo. In this blog post, we'll address common issues, provide easy solutions, and ensure you leave with a clear understanding of how to use regex queries effectively.
The Scenario 📚
Let's consider the following document structure in our MongoDB collection:
{
"files": [
"File 1",
"File 2",
"File 3",
"File 4"
],
"rootFolder": "/Location/Of/Files"
}
The Problem ❗
Our goal is to retrieve all the files that match the pattern *File
. Initially, you might attempt the following query:
db.collectionName.find({'files':'/^File/'})
However, when executing this query, you end up with no results. Frustrating, isn't it? But fear not, there's a simple explanation behind this unexpected outcome.
The Solution 💡
The issue lies in the way we're using the regex pattern within the query. To perform a regex query in PyMongo, we need to use the $regex
operator. The correct query to achieve our goal would be:
db.collectionName.find({'files': {'$regex': '^File'}})
By using the $regex
operator, we inform PyMongo to interpret the value as a regular expression pattern.
The Explanation 🧐
In the initial query, we mistakenly used the /^File/
syntax, which is commonly used in other programming languages, but not supported by PyMongo. This syntax is used in JavaScript and MongoDB console to perform regex queries. However, PyMongo uses Python syntax, and therefore requires the $regex
operator within a dictionary.
The Call-to-Action 📣
Now that you understand the correct way to perform regex queries with PyMongo, go ahead and give it a try! If you come across any other issues or have questions, don't hesitate to reach out to us in the comments section below. We're here to help you.
Happy regex querying! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
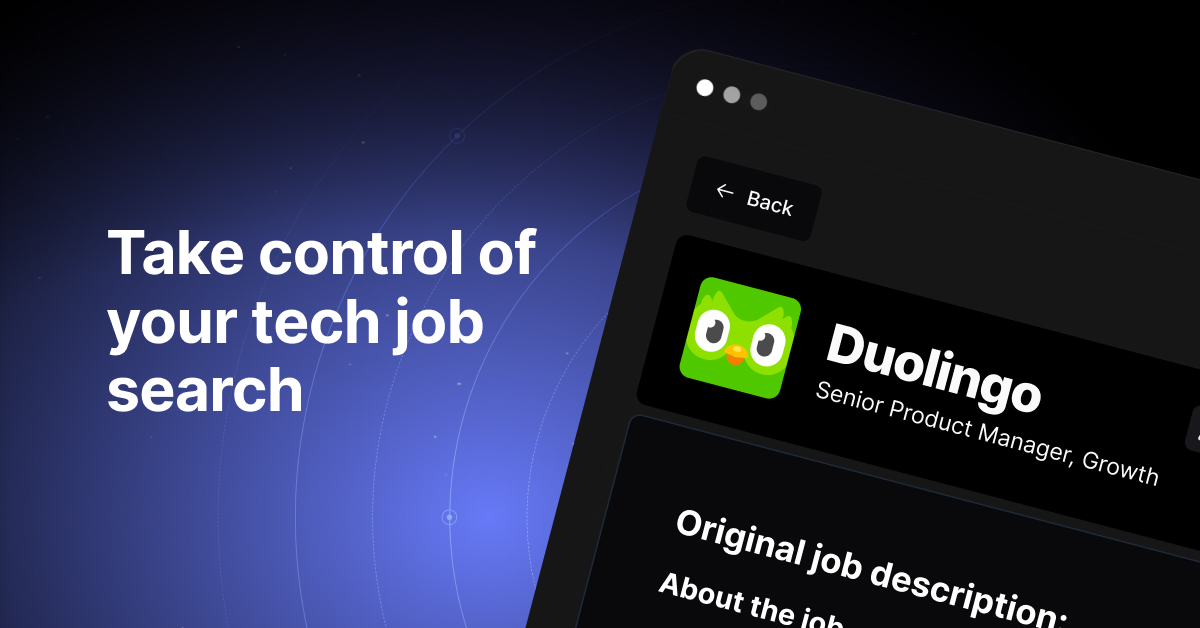