Node.js Mongoose.js string to ObjectId function
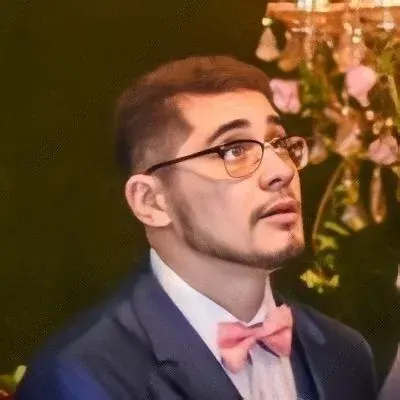
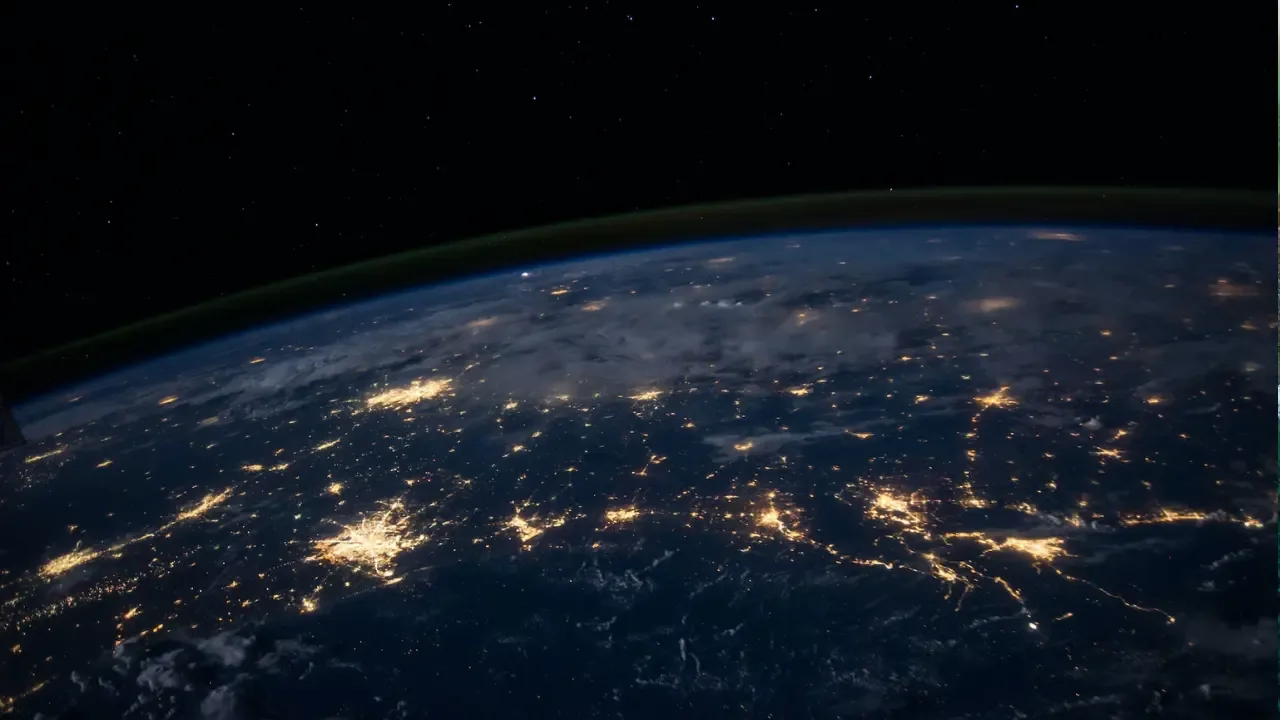
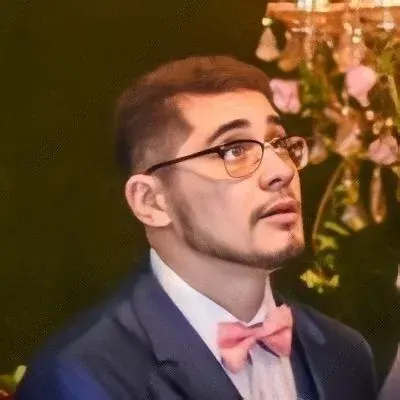
📢 Hey there! Are you struggling with converting a string to an ObjectId in Node.js using Mongoose.js? 🤔 Don't worry, you're not alone! Many developers have encountered this common issue and I'm here to help you find easy solutions. Let's dive right into it! 💪
The Problem
So, the problem you're facing is that despite specifying in your Mongoose schema that a field should be an ObjectId, when you save the value as a string, MongoDB still treats it as a regular string. 🙄 For example, the _id property of the object is displayed as objectId("blah")
.
The Solution
To overcome this hurdle, you can make use of Mongoose's built-in Types.ObjectId
function. It allows you to explicitly convert a string into an ObjectId, ensuring that MongoDB recognizes and treats it accordingly.
Here's how you can do it:
const mongoose = require('mongoose');
const { Types } = mongoose;
const myString = 'your_string_here';
const myObjectId = Types.ObjectId(myString);
// Now, you can safely use myObjectId in your Mongoose operations!
By utilizing the Types.ObjectId
function, you can seamlessly convert your string into a proper ObjectId. This ensures consistency and eliminates any issues that may arise when working with your data. 🎉
Further Tips
Make sure you have Mongoose installed and set up in your Node.js project. If you haven't done so yet, you can install it using npm:
npm install mongoose
.Remember to check if the converted ObjectId is valid. You can use the
Types.ObjectId.isValid
method provided by Mongoose:if (Types.ObjectId.isValid(myString)) { const myObjectId = Types.ObjectId(myString); // Rest of your logic here } else { // Handle invalid ObjectId string }
Share Your Thoughts!
I hope this guide has helped you solve the string to ObjectId conversion issue in Node.js using Mongoose.js. Feel free to drop a comment below and share your thoughts or any alternative solutions you may have come across! Let's grow together as a development community. 😊💬
Remember, if you have any more questions or face other challenges, I'm here to assist you. Keep coding and happy developing! 🚀👩💻👨💻