Mongoose"s find method with $or condition does not work properly
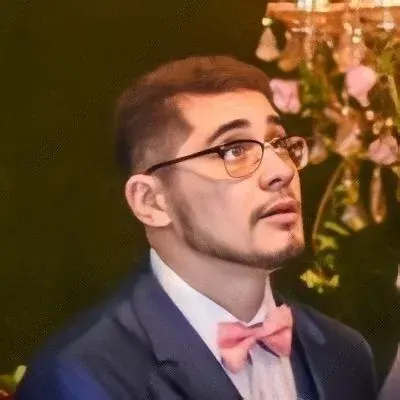
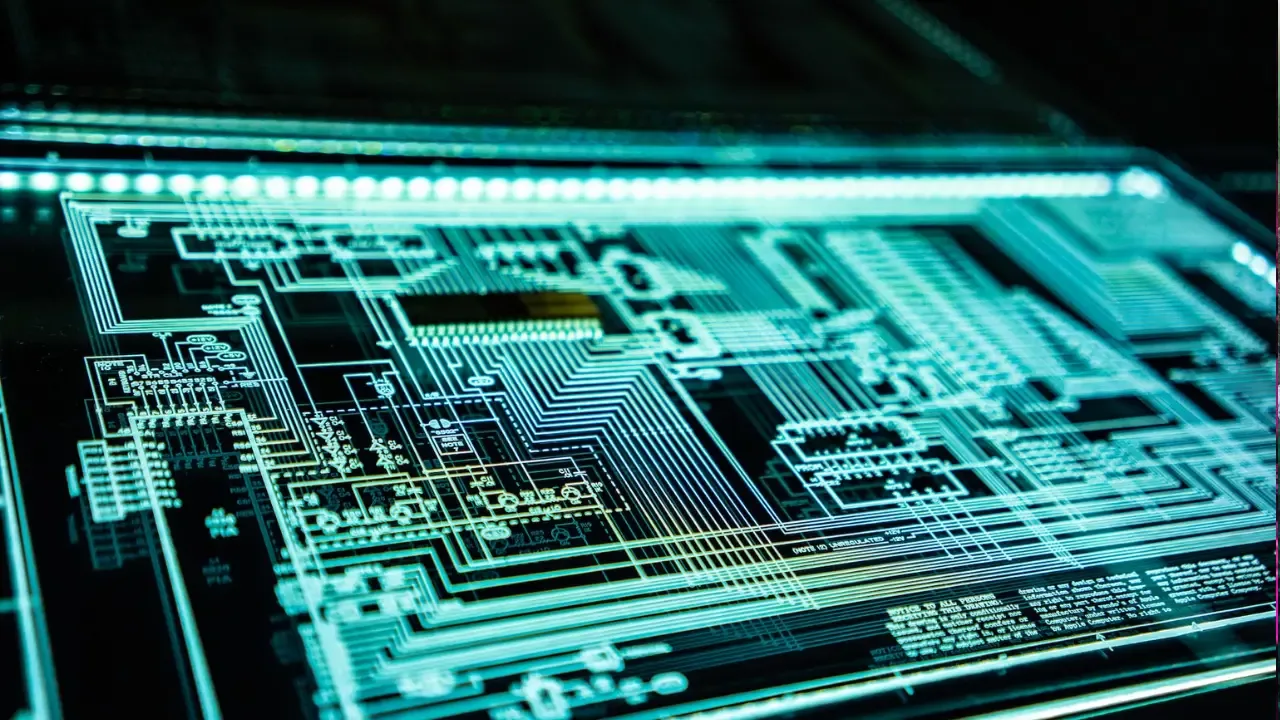
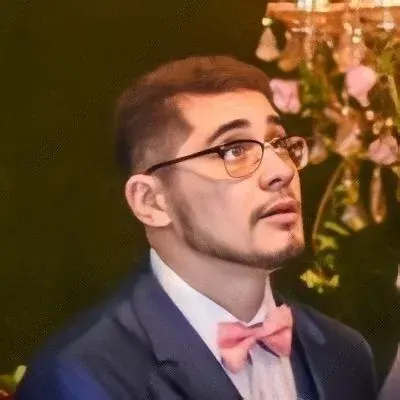
Mongoose's find method with $or condition: A Common Issue and Easy Solutions 😕🔍
<p>👋 Hey there! Welcome to another tech blog post where we'll tackle a common issue that developers face when using Mongoose's find method with $or condition. If you've been scratching your head wondering why it's not working as expected, you're in the right place! </p>
The Problem 🤔
<p>Here's the scenario: you started using MongoDB with Mongoose on your Node.js app, and everything seems to be going smoothly. Until you try to use the Model.find method with $or condition and the _id field. Suddenly, Mongoose throws a curveball and doesn't work properly. What's going on here? Let's dissect the issue further. </p>
<p>In the given context, a code snippet demonstrates the problem:</p>
User.find({
$or: [
{ '_id': param },
{ 'name': param },
{ 'nickname': param }
]
}, function (err, docs) {
if (!err) res.send(docs);
});
<p>Surprisingly, this code snippet fails to fetch the expected results. However, when the specific '$or' condition involving '_id' is removed, it magically starts working again:</p>
User.find({
$or: [
{ 'name': param },
{ 'nickname': param }
]
}, function (err, docs) {
if (!err) res.send(docs);
});
<p>To add to the mystery, both versions work properly when used directly in the MongoDB shell. So why does Mongoose behave differently? Let's dive into the solutions! </p>
Solution 1: Verify the 'param' Value 🧐📝
<p>Sometimes, the issue lies in the value of the 'param' variable. Double-check if it contains a valid value that matches the documents in your MongoDB collection. Keep in mind that Mongoose performs strict comparison when searching for documents. Make sure the 'param' value matches the data type and format of the field you're querying.</p>
Solution 2: Use Mongoose's ObjectID Properly 🆔💡
<p>In the context of the '_id' field issue, it's crucial to ensure you're using Mongoose's ObjectID properly. If 'param' is a string representation of a valid MongoDB ObjectID, Mongoose might not handle the comparison correctly. To solve this, convert the 'param' to an ObjectID before passing it to the query. You can do this by using the mongoose.Types.ObjectId method:</p>
const mongoose = require('mongoose');
const objectIdParam = mongoose.Types.ObjectId(param);
User.find({
$or: [
{ '_id': objectIdParam },
{ 'name': param },
{ 'nickname': param }
]
}, function (err, docs) {
if (!err) res.send(docs);
});
<p>By converting the 'param' to an ObjectID, Mongoose will now perform the comparison accurately and fetch the expected results.</p>
Solution 3: Enable Mongoose's 'useUnifiedTopology' Option 🏁🔌
<p>Another solution for this quirky behavior is related to Mongoose's connection settings. If you're using an older version of Mongoose (5.x or earlier), the default value of the 'useUnifiedTopology' option is 'false'. This can potentially lead to unexpected behavior, such as the $or condition not working properly.</p>
<p>To mitigate this, ensure you set 'useUnifiedTopology' to 'true' when establishing the Mongoose connection:</p>
mongoose.connect(uri, {
useUnifiedTopology: true,
// ...
});
<p>By enabling this option, you ensure that Mongoose uses the new unified topology engine, avoiding any potential glitches that might affect the functionality of certain queries.</p>
Time to Tackle the Issue! ⏰🚀
<p>Now that you have three handy solutions to fix the Mongoose find method issue with $or condition, it's time to dive back into your code and implement the one that suits your specific situation. Remember to double-check the 'param' value, use Mongoose's ObjectID properly if needed, and enable the 'useUnifiedTopology' option when establishing the Mongoose connection. </p>
<p>If you found this blog post helpful, be sure to share it with your fellow developers who might be facing the same Mongoose issues. Also, leave a comment below if you have any questions, additional insights, or if you encountered any other peculiar Mongoose peculiarities. Let's help each other out! Happy coding! 🎉👩💻👨💻</p>