Mongoose Unique index not working!
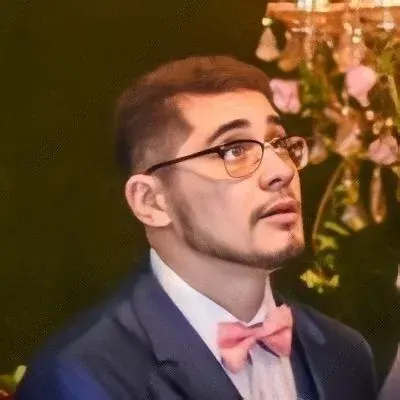
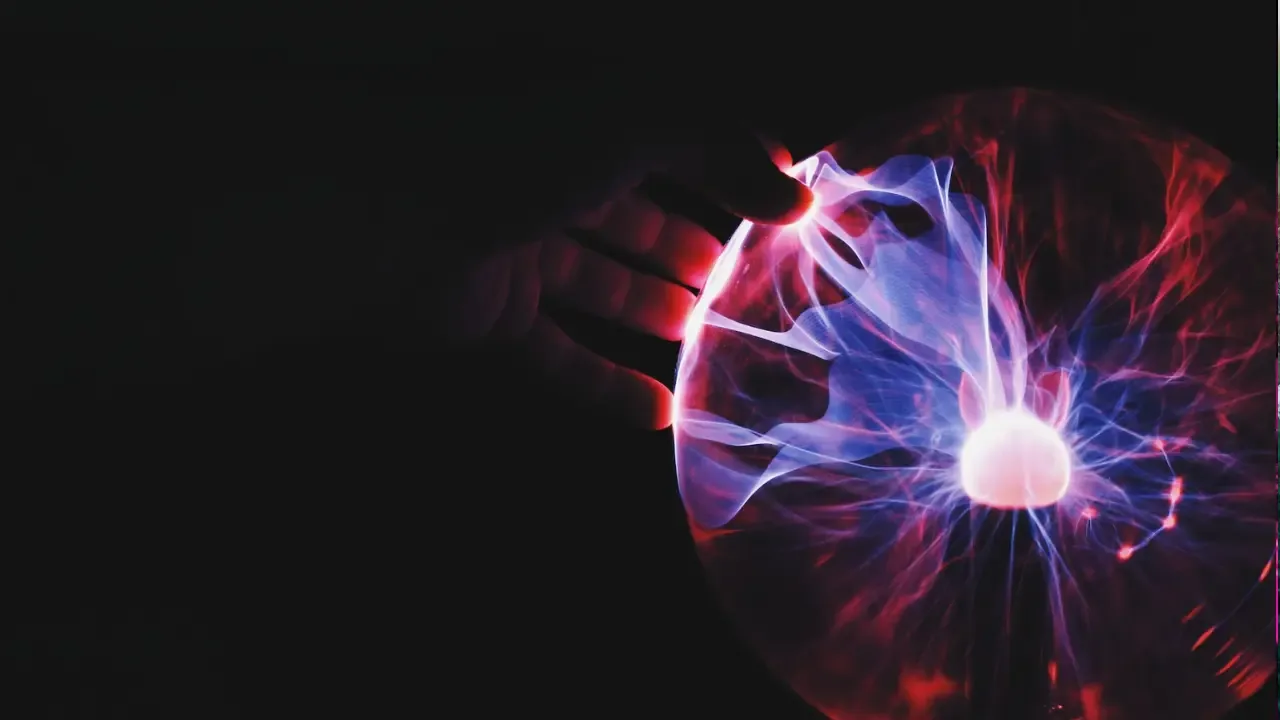
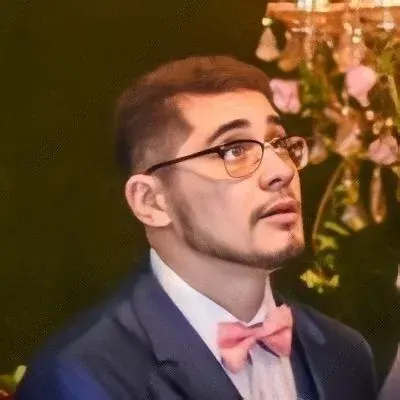
🐍 Mongoose Unique Index Not Working: A Common Issue and Easy Solutions
So, you're working with Mongoose and trying to create a unique index in MongoDB but it seems like it's not working as expected? Don't worry, you're not alone! This is a common issue faced by many developers. Let's dive into the problem and explore some easy solutions.
Understanding the Problem
In the context given, the developer was trying to create a unique index for the "email" field in the "User" schema. The code snippet used was:
User = new Schema({
email: {type: String, index: {unique: true, dropDups: true}}
});
However, despite specifying the unique: true
option, the system allowed the developer to save two users with the same email. Quite frustrating, right?
Why Mongoose Unique Index Fails
The reason behind this issue lies in how Mongoose handles unique indexes by default. Mongoose does not send an error when trying to save a duplicate value. Instead, it throws a MongoDB duplicate key error when the server responds.
Since Mongoose performs validation on the client-side, it cannot guarantee the uniqueness of the index. This behavior is intentional to allow flexibility in cases where you might want to handle the error differently or perform other actions before reaching the server.
Easy Solutions
Use the
unique
property as a ValidatorMongoose provides a way to define unique fields as validators in the schema. By using this approach, Mongoose will validate the uniqueness on the server-side and throw a validation error if a duplicate value is found.
const userSchema = new Schema({ email: { type: String, unique: true, dropDups: true } });
With this approach, when you attempt to save a duplicate value, Mongoose will throw a validation error that you can catch and handle accordingly.
Use the
index
method with theunique
optionAnother way to enforce the uniqueness of a field is by explicitly creating an index using the
index
method and setting theunique
option totrue
.const userSchema = new Schema({ email: String }); userSchema.index({ email: 1 }, { unique: true, dropDups: true });
This method creates an index on the "email" field with the
unique
option set totrue
. Now, if you try to save a duplicate value, MongoDB will throw an error at the server level, ensuring the uniqueness of the index.Manually check for duplicates before saving
If you prefer to handle the uniqueness check manually, you can query the database for existing values before saving the document. This approach can be useful in scenarios where you want to provide custom error messages or apply additional logic before saving.
const User = mongoose.model('User', userSchema); async function createUser(email) { const existingUser = await User.findOne({ email }); if (existingUser) { throw new Error('Email already exists!'); } const newUser = new User({ email }); await newUser.save(); }
By executing a find query before saving, you can check if the "email" value already exists and take appropriate actions based on the result.
Wrapping Up
Dealing with the "Mongoose Unique Index Not Working" issue can be frustrating, but now you have some easy solutions at your disposal.
Remember, Mongoose does not provide out-of-the-box validation for unique indexes, but you can overcome this by using the unique
property as a validator, creating an index explicitly, or manually checking for duplicates before saving.
Now it's your turn! Have you faced this issue before? Which solution worked best for you? Share your thoughts and experiences in the comments below! 🗣️💬